Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial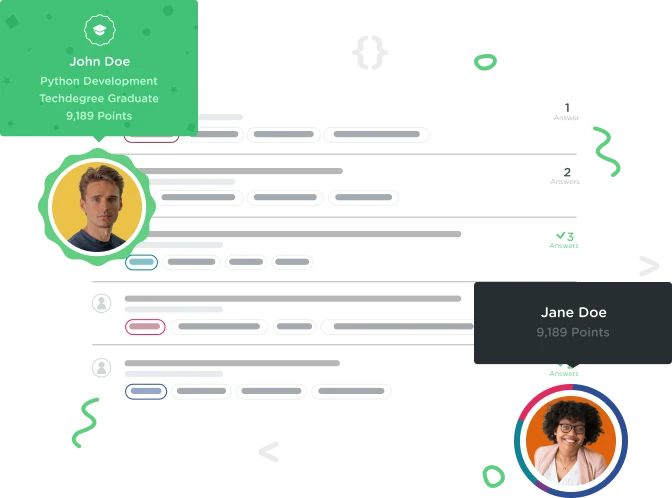

Alex Hickey
6,570 PointsWith a slightly different approach, can't get the overlay to disappear on click.
I tried an alternate solution to make the lightbox overlay show:
// User clicks on <a> tag wrapping an image inside a list item -- capture click event
$( ".gallery li a" ).click( function( event ) {
// prevent default link behavior
event.preventDefault();
// get the image attributes to pass to the overlay function
var imageLocation = $( this ).attr( "href" );
var alt = $( this ).children( "img" ).attr( "alt" );
// console.log( imageLocation );
// call the overlay function to create the lightbox elements
overlay( imageLocation, alt );
});
// Function definition to add overlay with
// image
// caption
function overlay( src, alt ) {
// append the overlay div and child elements to the document body
$( "body" ).append( "<div id='overlay'><img><p></p></div>" );
// add href, alt and caption content to selected elements
$( "#overlay img" ).attr( "src", src );
$( "#overlay img" ).attr( "alt", alt );
$( "#overlay p" ).append( alt );
}
That part all works, and looks just like Andrew's version. Then I added a click listener to the #overlay element:
// When the overlay is clicked, remove from DOM
$( "#overlay" ).click( function() {
$( this ).remove();
});
But for some reason I can't fathom, nothing at all happens when I click. Not sure where I'm going wrong... any thoughts? This is very similar to Andrew's approach except that I didn't use a variable to store a jQuery object, I just create a new object with appropriate attributes whenever the function is called.
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Alex,
I think the problem you're having is that the click handler never gets attached to the overlay element because it's not in the dom yet when that click handler code is processed. The click
method does not work for dynamically added events that are added later on.
You can try using event delegation instead which will work on dynamically created elements that are added later on.
$("body").on ("click", "#overlay", function() {
$( this ).remove();
});
What this does is attach the click handler to the body element. Then any element with an id of overlay can receive clicks and that click event will then bubble up to the body which will run the handler for it.
I did not test this with your code so let me know if it doesn't work.
I'm not sure if you were doing this just to get more practice and see how things worked this way but repeatedly creating and destroying the overlay seems like it would be an inefficient approach. As opposed to just hiding and showing it.
Alex Hickey
6,570 PointsAlex Hickey
6,570 PointsThanks Jason. I've come to a similar conclusion after playing around with it a little bit--the div with the #overlay id isn't available for the click handler early enough.
As for why, I'm just trying to get a better feel for jQuery--I like to try to solve each piece my own way, before looking at the teacher's code, then compare them or use the example code to fix problems in my solutions. I find I learn a lot faster that way than just following along.