Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial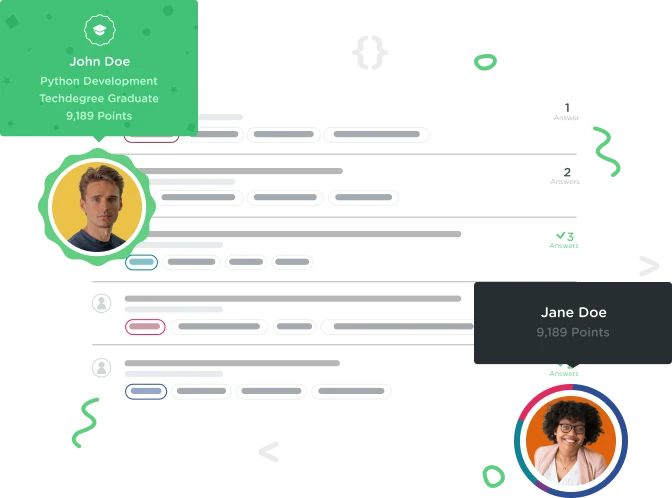

Danielle Fankhauser
1,338 PointsWith Rails 4 and Devise, I get an error with this code - undefined method `authenticate'
Hi, I use Devise for authentication in my app. I would like users to be able to sign in and out, and use current_user for users accessing the app through the API as well.
I am getting this error: undefined method `authenticate' for #<User:0x007fc16c3a3e48>
for this line: if user && user.authenticate(password)
I do not have a Sessions Controller because I use Devise. Can you point me in the right direction? Thanks!
API Controller
module Api
class ApiController < ApplicationController
skip_before_filter :verify_authenticity_token
protect_from_forgery with: :null_session
#before_action :authenticate_api_user!, only: [ :create ]
before_filter :authenticate
def current_user
@current_user
end
def authenticate
authenticate_or_request_with_http_basic do |email, password|
Rails.logger.info "API authentication:#{email} #{password}"
user = User.find_by(email: email)
if user && user.authenticate(password)
@current_user = user
Rails.logger.info "Logging in #{user.inspect}"
true
else
Rails.logger.warn "No valid credentials."
false
end
end
end
end
end
2 Answers

Manuel Quintanilla
3,083 PointsYou get that error because of nesting devise within the :api namespace in your routes.rb file. Therefore, you should authenticate users in the following way:
class Api::PostsController < ApplicationController
before_action :authenticate_api_user!, only: [ :create ]
end

Gareth Jones
2,095 PointsDid you ever find a solution to this? Currently having the same issue.
Danielle Fankhauser
1,338 PointsDanielle Fankhauser
1,338 PointsHi, thanks for the answer - I tried that but I am actually just trying to log in and out, I haven't even gotten to creating posts yet. I added more detail above in case you can help. Thanks!