Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial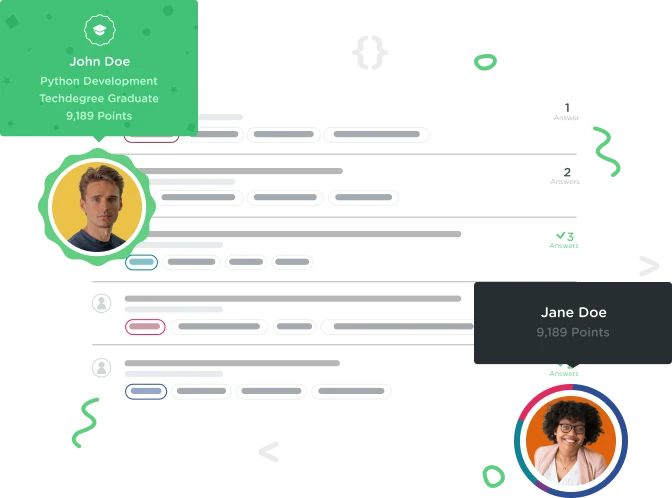
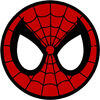
Taylor Hall
Front End Web Development Techdegree Graduate 14,496 PointsWith these Array videos, please can someone explain to me why we don't include an S when we are referencing our arrays?
const names = ['Selma', 'Ted', 'Mike', 'Sam', 'Sharon', 'Marvin'];
let sNames = [];
names.forEach((name, index) => console.log(${index + 1}) ${name}
));
For instance how does the computer know that 'name' is in reference to the 'names' array?
4 Answers
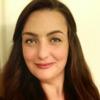
Jennifer Nordell
Treehouse TeacherHi there, Taylor Hall! The short answer is... it doesn't know that But let's back up. When you run a
forEach
on an array, it's going to go through the whole thing and each individual item will be assigned to a variable. In this case, name
. So you run names.forEach()
That's how it knows it should be working with the names
array.
In the first iteration, name
will be equal to 'Selma' and 'index' will be 0. The second iteration name
will be equal to 'Ted'and
index` will be equal to 1. It goes on like the entire length. But you could have also written that like this:
const names = ['Selma', 'Ted', 'Mike', 'Sam', 'Sharon', 'Marvin'];
let sNames = [];
names.forEach((student, number) => console.log(`${number + 1}) ${student}`));
This would produce exactly the same results. The first iteration the variable student
is assigned 'Selma', and number
is assigned 0.
Hope this helps!
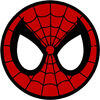
Taylor Hall
Front End Web Development Techdegree Graduate 14,496 PointsJennifer Nordell, thank you for explaining my question. I think I understand certain elements of it. But what is now confusing me, how does it know:
names.forEach(student, number)
How does it know that student means log the string and number means put a number next to it? Sorry for the questions.

Nick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsHey Taylor Hall , I won't speak for Jennifer Nordell here, but the way that JavaScript knows what goes where is all a part of how the method works. For example, when you check the MDN page forEach, it shows you what arguments to add when you use it. The first thing that gets applied to each item in the array is the callback function.
Per MDN:
arr.forEach(callback(currentValue[, index[, array]]) {
// execute something
}[, thisArg]);
So in your example above, you would need a callback function added that uses student
and number
as your arguments. names
is your array, forEach
is the JavaScript method that applies the callback function
you provide to each item in the array (in Jennifer's example above it's an anonymous function that console.logs the template literal ${number + 1}) ${student}
for each item in the array with
student
and number
as the parameters (i.e. arguments)). student
is the currentValue
and number
is the index
.
student
and number
could be monkey
and banana
or cat-poop
and litterbox
. As long as you keep track of what you want and where you want them.
Hopefully this makes sense!
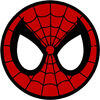
Taylor Hall
Front End Web Development Techdegree Graduate 14,496 PointsNick Huemmer Hey Nick, thank you for taking the time to write all of that. I will try to figure it out on my own as I am sorry but I still do not understand why in this example:
const names = ['Selma', 'Ted', 'Mike', 'Sam', 'Sharon', 'Marvin'];
let sNames = [];
names.forEach((student, number) => console.log(${number + 1}) ${student}
));
why this results in a number next to the student name. No worries though I will try to find something that explains it in layman terms.

Junior Aidee
Front End Web Development Techdegree Graduate 14,657 PointsHello Taylor Hall ! I think the confusion may stem from the fact that the example in the MDN docs is using a declaration function whereas the example in the video is using an anonymous function but the concept is still the same.
Nick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsNick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsThis is pretty helpful for understanding the parameters passed into the callback functions - we see them used but it hasn't been explained that what they're called can be arbitrary, just as long as something is passed in!
Jennifer Nordell - in the example above, how does JS or the JS engine know to assign the array index number to
number
and the student names in the array tostudent
? Is it a matter of the order that the parameters are passed into the function?Ooops - nevermind - I just watched the start of the video and it's explained in the syntax on the MDN site
forEach
e.g. from the page:arr.forEach(callback(currentValue[, index[, array]]) {
index is the second argument for this function. Hopefully I'm understanding this right.