Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial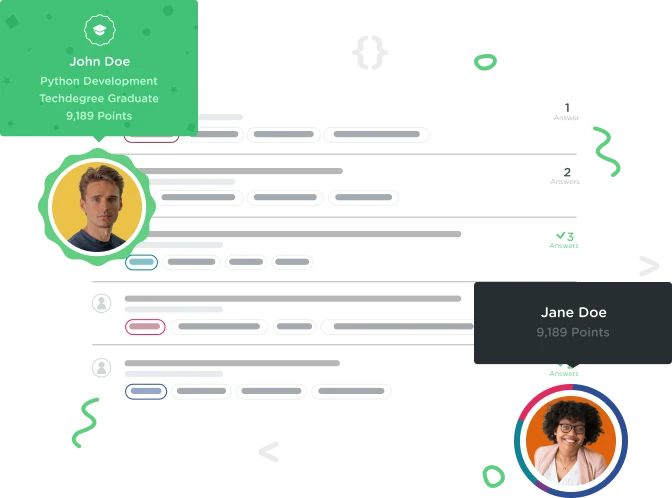

Bobtrade Limited
265 PointsWithout renaming any of the variables, modify the data callback to concatenate the stream of data to the
Without renaming any of the variables, modify the data callback to concatenate the stream of data to the
const https = require("https");
const request = https.get("https://teamtreehouse.com/chalkers.json", response => {
let responseBody = "";
response.on("data", dataChunk => {
console.log(Body.response);
});
response.on("response", (responseBody) => {
console.log(responseBody);
});
});
request.on("error", error => {
console.error(error.message);
});
3 Answers

Ravindra Shekhawat
5,869 Points// i guess it will work for you...
const https = require("https"); const request = https.get("https://teamtreehouse.com/chalkers.json", response => { let responseBody = "";
response.on("data", dataChunk => {
responseBody += dataChunk.toString();
});
response.on("end", () => {
console.log(responseBody);
console.log(typeof responseBody);
});
});
request.on("error", error => { console.error(error.message); });

Sandra Jonsson
4,875 PointsFINAL CODE:
const https = require("https");
const request = https.get("https://teamtreehouse.com/chalkers.json", response => {
let responseBody = "";
response.on("data", dataChunk => {
responseBody += dataChunk.toString();
});
response.on("", () => {
console.log(responseBody);
});
});
request.on("error", error => {
console.error(error.message);
});
If you are wondering what's happening on line 1 const https = require("https");
:
see "https://stackoverflow.com/questions/31769175/what-is-the-purpose-of-this-var-http-requirehttp"
HOW TO SOLVE:
First: Look at the data
Callback
(This is the 'data callback' they speak of in the question)
response.on("data", dataChunk => {
console.log(Body.response);
});
Here we call response.on("data")
.
.on
is an event listener that waits on the "data" from our URL.
"data" is an event that emits a Buffer by default, if .setEncoding()
has not been used.
(Example of how .setEncoding()
is used: see "API for stream Consumers": "https://nodejs.org/api/stream.html")
OBS they do not ask for us to use .setEncoding()
in this question.
When data is received from our URL, our .on
event listener triggers our callback dataChunk
.
Here, is where ThreeHouse wants us to write code that concatenates (i.e. adds/strings together) the stream of data we just received and the variable responseBody
(currently set equal to an empty string).
let responseBody = "";
issue: our stream of data saved in dataChunk
is a buffer & not a string.
solution: add the .toString()
method to the name of our callback dataChunk
More info on toString() on MDN: "https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/toString"
We do this because:
dataChunk
holds the value of response
once it has received its "data" ( dataChunk = response.on('data')
)
and response
holds the value of the get request answer from the ThreeHouse URL.
i.e. in loose terms:
dataChunk.toString();
in this specific case and position is the same as:
https.get("https://teamtreehouse.com/chalkers.json")~.on("data")~.toString();
dataChunk.toString();
should therefore give us a stringy-fied version of the data stream received from our get-request.
And to concatenate our new-made string of data to the "responseBody" 's empty string we can write:
responseBody += dataChunk.toString();
more on the types of concatenation in JS see: "https://masteringjs.io/tutorials/fundamentals/string-concat"
Hope this helped Good Luck!

Rana Dugal
9,506 Pointsconst https = require("https"); const request = https.get("https://teamtreehouse.com/chalkers.json", response => { let responseBody = "";
response.on("data", dataChunk => { responseBody += dataChunk.toString(); });
response.on("end", () => {
console.log(responseBody);
console.log(typeof responseBody);
});
});
request.on("error", error => { console.error(error.message); });