Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial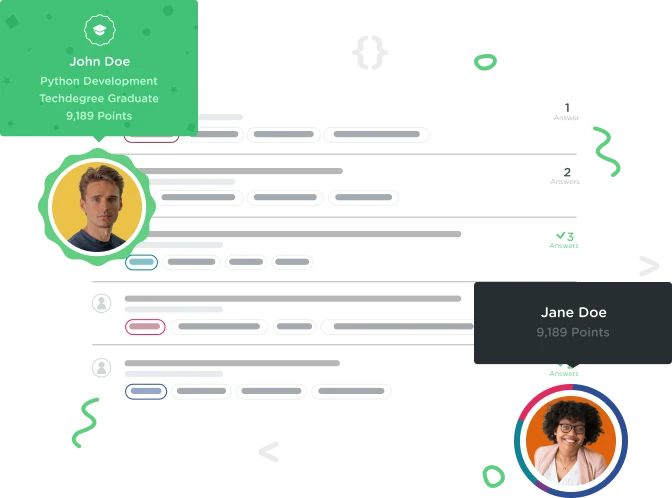
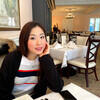
Hanwen Zhang
20,084 PointsWithout renaming any of the variables, modify the data callback to concatenate the stream of data to the response
What does this question trying to ask here? I dont quite understand this question
const https = require("https");
const request = https.get("https://teamtreehouse.com/chalkers.json", response => {
let responseBody = "";
response.on("data", dataChunk => {
});
response.on("", () => {
console.log(responseBody);
});
});
request.on("error", error => {
console.error(error.message);
});
3 Answers
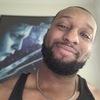
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi Hanwen,
The data callback, is the callback that's invoked on the "data" event. In this case, it's the callback that goes dataChunk => {});
.
As you may notice, there's no code in this function's code block (ie. between the parens).
The challenge wants you to take the stream of data that's being passed as an argument into the callback (when the "data" event is fired) and concatenate it to the response (which is the empty string stored into the responseBody variable).
Hopefully, that offers enough of a breakdown of the question for you to figure out the answer on your own, but if you can't, let me know and I'll write down the solution.
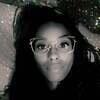
Kelinia Johnson
10,189 PointsI don't understand your explanation
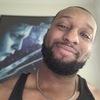
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi Kelinia Johnson,
Say you have some code that looks something like this...
button.addEventListener('click', event => {
console.log(event.target)
})
Assuming that button is a valid variable/element, we know that when the button is clicked, the target of that click will be logged to the console (I'm assuming that based on your point total in JavaScript that you've encountered code that looks like this; if I'm wrong about this let me know).
We know the event object is what is automatically passed to the callback function (which is the arrow function that is invoked once the button is clicked).
event (like dataChunk) is just the name we give to represent the the argument/data that's being passed in. In theory, you could switch the name of event (or dataChunk) to barbecue and nothing about the way your code runs would change (it just wouldn't be a semantic name, and might potentially confuse other coders).
The code in the challenge is very much like that, except there isn't only one dataChunk that is passed into the callback function.
Envision dataChunk as if it were some really long string. A string that's 5000 characters long. And because it's so long it can't all be passed into the callback function at the same time. The first 1250 characters are passed in, then the next 1250, and so on and so on.
Each time the data is ready, the 'data' event is triggered on the response object. And so each time the data is ready, the callback function of ((dataChunk) => {}) is called.
dataChunk is the variable that represents the first 1250 characters, then the second 1250 characters, then the third 1250 characters, and finally the last 1250 characters.
What code would you need to write inside of the callback function ((dataChunk) => {}) to make sure that the responseBody variable holds all 5000 characters, and not just a portion of it? Does that make more sense?
My first experience with XMLHttpRequests was a little difficult to process/comprehend. But once I grew to being able to see "response.on" like any other element/object that I was listening for an event on, it became easier to visualize the mechanics behind it.
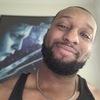
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsJust in case, that explanation doesn't clear things up enough, I'm including the solution for the first part of the challenge below:
const https = require("https");
const request = https.get("https://teamtreehouse.com/chalkers.json", response => {
let responseBody = "";
response.on("data", dataChunk => {
responseBody += dataChunk
});
response.on("", () => {
console.log(responseBody);
});
});
request.on("error", error => {
console.error(error.message);
});
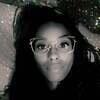
Kelinia Johnson
10,189 PointsThank you for the explanation!