Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial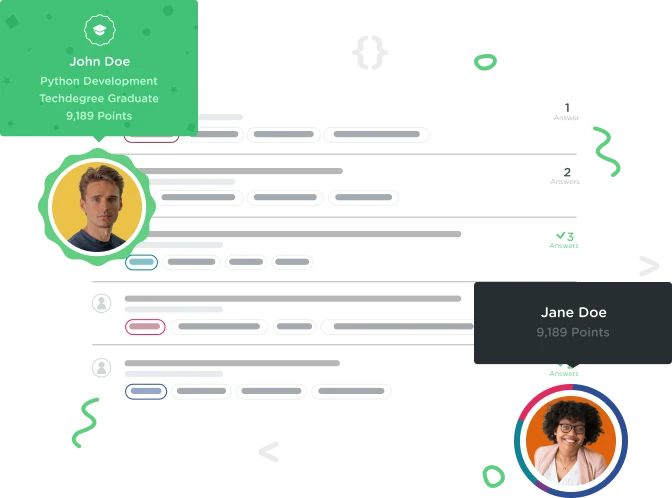

Michael Lo
2,785 PointsWondering why this code prints the number '4' to the document.
//data structure
//name, track, achievments(num), points(num)
var students = [
{name: 'David', track: 'IOS', achievments: 10, points: 200},
{name: 'Kate', track: 'JS, IOS', achievments: 20, points: 1200},
{name: 'Emily', track: 'Windows', achievments: 30, points: 2200},
{name: 'joan', track: 'Ruby', achievments: 40, points: 2030},
{name: 'Ben', track: 'IOS', achievments: 50, points: 2001},
];
//print function
function print(message) {
var div = document.getElementById('output');
div.innerHTML = message;
}
//loop the student records to the page.
for (var key in students) {
print(key, ', ', students[key]);
}

Tolumi Adamson
Front End Web Development Techdegree Student 11,645 PointsYeah, that drove me crazy for a bit. I believe the problem is that you are using an object loop to try and access an array. I might be wrong, but that is what I figure. to access an array, you generally need to use the array name plus the location of the item you are trying to access i.e. students[0]. whereas using the for in loop, you are trying to access it in a different manner. I actually had to use a regular for loop to first try and access the array itself, then a for in loop to access the object within the array. my code looked something like this
for (var i=0; i < students.length; i += 1 ) {
for (var key in students[i]) {
html += key + ':' + students[i].key + '<br><br>';
}
}
1 Answer
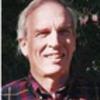
jcorum
71,830 PointsSet this up in my IDE. This index.html file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Students</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<h1>Students</h1>
<div id="output">
</div>
<script src="js/students.js" type="text/javascript"></script>
</body>
</html>
and this students.js file:
//data structure //name, track, achievments(num), points(num)
var students = [ {name: 'David', track: 'IOS', achievments: 10, points: 200}, {name: 'Kate', track: 'JS, IOS', achievments: 20, points: 1200}, {name: 'Emily', track: 'Windows', achievments: 30, points: 2200}, {name: 'joan', track: 'Ruby', achievments: 40, points: 2030}, {name: 'Ben', track: 'IOS', achievments: 50, points: 2001}, ];
//print function
function print(message) {
var div = document.getElementById('output'); div.innerHTML = message; }
//loop the student records to the page.
for (var key in students) {
print(key, ', ', students[key]);
}
print("Hello");
and it printed Hello.
Not sure how you were calling the print() function in your html, but everything seems to be working fine. I thought at first that perhaps you were asking for the value of one of the keys in the data structure, but since none is 4, it must be something else.
Happy coding!
Jacob Mishkin
23,118 PointsJacob Mishkin
23,118 Pointsedited code for formating