Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial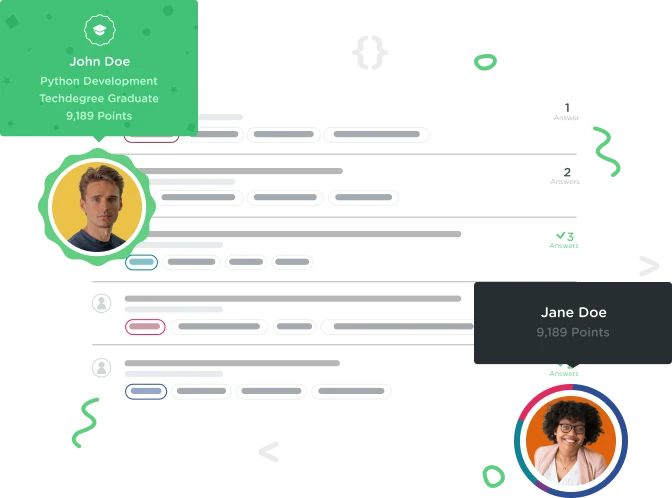
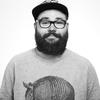
Øyvind Stokke-Zahl
3,169 PointsWon't compile
In the code challenge (Swift Recap Pt 1) I get a compiler error on this code:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
init(title: String, author: String, tag: String) {
self.title = title
self.author = author
self.tag = Tag(name: tag)
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "iOSDevelopment", author: "Apple", tag: "swift")
let postDescription = firstPost.description()
But in Xcode I get no errors, and when I open Preview to see the error, it's empty. What am I doing wrong?
3 Answers
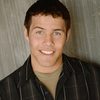
Kyler Smith
10,110 PointsVery close! Here is how I did it. I skipped the init method and declared the Tag name in the parameter for the firstPost.
struct Tag {
let name: String
}
struct Post {
var title: String
var author: String
var tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "aTitlte", author: "anAuthor", tag: Tag(name: "aTag"))
let postDescription = firstPost.description()
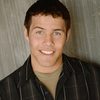
Kyler Smith
10,110 PointsBoris Likhobabin,
You are still giving tag a name, just inside the parameters of the Post instance. You could do the same thing and put the tag instance on a separate line as is below. They both accomplish the same thing.
// two lines
let swiftTag = Tag(name: "swift")
let firstPost = Post(title: "iOSDevelopment", author: "Apple", tag: swiftTag)
// one line
let secondPost = Post(title: "iOSDevelopment", author: "Apple", tag: Tag(name: "swift"))
Ryan Doyle
8,587 PointsI think maybe Xcode thinks it's fine, but the challenge wants it without the init method since it's a struct and wants you to rely on the member wise init.

Boris Likhobabin
3,581 PointsKyler, could you please explain to me this: let firstPost = Post(title: "aTitlte", author: "anAuthor", tag: Tag(name: "aTag")) by doing this you create an instance of a Tag right in brackets without giving it a name! Hoe so? Is this allowed?
Thanks
Øyvind Stokke-Zahl
3,169 PointsØyvind Stokke-Zahl
3,169 PointsPerfect. Thanks!
Kyler Smith
10,110 PointsKyler Smith
10,110 PointsNo Problem!