Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial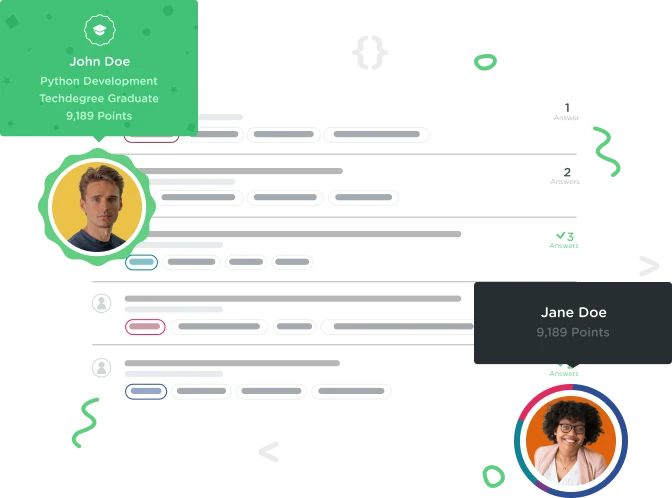
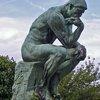
Payton Dwight
5,751 PointsWon't pass test. Working on my end.
See title. Thanks!
// After you've completed the TODOs locally paste Main.java here
package com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) throws IOException {
// write your code here
Prompter prompt = new Prompter();
String story = null;
try {
story = prompt.promptForStory();
} catch (IOException e) {
e.printStackTrace();
}
// String story = "Thanks __name__ for helping me out. You are really a __adjective__ __noun__ and I owe you a __noun__.";
Template tmpl = new Template(story);
prompt.run(tmpl);
/*List<String> fakeResults = Arrays.asList(
"friend",
"talented",
"java programmer",
"high five");*/
/*String results = tmpl.render(fakeResults);
System.out.printf("Your TreeStory:%n%n%s", results);*/
}
}
// After you've completed the TODOs locally paste Prompter.java here
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String ans = tmpl.render(results);
System.out.printf("Your tree story: %n%n %s", ans);
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
System.out.printf("Please enter a word for %s:%n", phrase);
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
String word = mReader.readLine();
while (mCensoredWords.contains(word)) {
System.out.printf("Censored word (%s) found. Please try again. %n", word);
word = mReader.readLine();
}
return word;
}
public String promptForStory() throws IOException {
System.out.printf("Please enter story template:%n");
return mReader.readLine();
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story
4 Answers
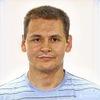
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsHi, Payton Dwight
The problem was funny :) I'll report to Craig tomorrow.
To fix first error, that is
JavaTester.java:77: error: unreported exception IOException; must be caught or declared to be thrown
Main.main(null);
^
1 error
The problem is that, when you catch IOException, you should not leave your main
method declared throws
// if you try catch Exception, remove throws from here
// if you don't use try catch, then leave it here.
public static void main(String[] args) throws IOException { // remove throws !!!
// write your code here
Prompter prompt = new Prompter();
String story = null;
try {
story = prompt.promptForStory();
} catch (IOException e) {
e.printStackTrace();
}
The second error that comes is
Bummer! Whoops looks like you forgot to remove the TODO's after you completed them. This helps everyone know it is done
That is the one you know how to fix right ? :)
When you remove all TODOs comes, the hardest one
Whoops looks like you didn't censor the words (2a). mCensoredWords contains 'dork', but it made it past somehow.
This one is impossible to solve, unless you know the answer :) Which I do, fortunately.
It has nothing to do with your code, it has to do with the testing.
Long story short, replace
System.out.printf("Censored word (%s) found. Please try again. %n", word); // won't pass :( sorry
With
System.out.printf("Censored word found. Please try again. %n");
And you will pass the challenge :)
If you want to know why it does not pass, let me know I'll try to explain.
So try it again, let's if it works now :)
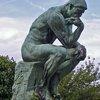
Payton Dwight
5,751 PointsHey Alexander,
Thank you for taking the time to write such a detailed response. I am curious as to why the last error arises in testing. Is it something to do with the way the tests are written?
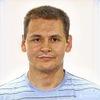
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsSo I don't know for sure, but he is testing all System.out.print
for censored words,
because he has to write tests not knowing details of your implementation.
So if you put anywhere in your output
System.out.printf("dork%n");
You will not pass.
Basically because they just look for censored words like "dork", and if they find such words, boom, you've got an error.
It is extremely hard to write tests for these challenges, I saw his code for Techdegree project 1, so yeah sometimes it is just hard to test the code that you don't know.
And that is exactly how he writes tests, inferring your structure.
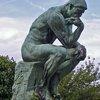
Payton Dwight
5,751 PointsThanks!

Nicholas Pretorius
18,684 PointsHi, I struggled for some time with this issue. I understand it is hard to write these tests. On the other hand, it is frustrating to be sitting with a local solution that 'works' but still 'failing' the challenge repeatedly.
Granted, failing the challenge several times lead to me re-evaluating parts of the solution and improving it. It also lead me to more thoroughly searching the forums. So, in the end, maybe it is good to be bumping your head against this for a while. However, had I not found this thread, who knows how long I would have sat with it.
My suggestion is to put more detailed instructions into the challenge or add more detailed 'tips' when errors are shown. For example, when displaying the censored word error, add a note to the reader to make sure not to include censored words in their prompts.
All things considered, it is more satisfying to solve the challenge and see the green success message after struggling with it, than it is to pass it the first time!
Luis Onate
13,222 PointsLuis Onate
13,222 PointsI was having issues with the "Whoops, looks like you didn't censor the words..." error. The above mentioned changed solved it.