Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial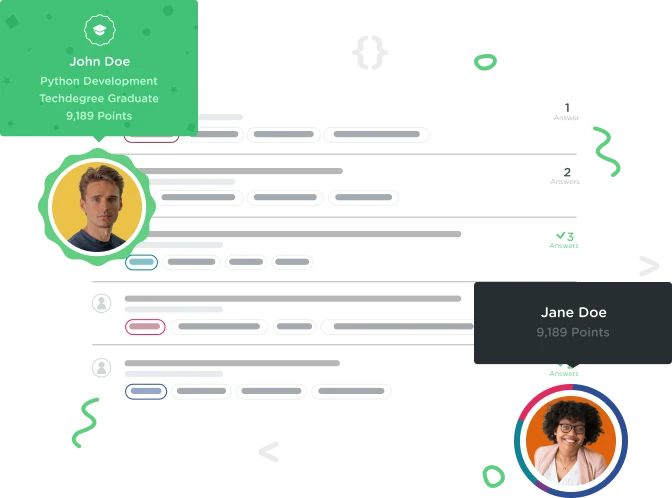

Steven Wheeler
2,330 PointsWord after "for" in a for loop?
Hey there!
There's one thing really holding me back understanding "for" loops in Python.. and that is the construction of them.
In the "Shopping List Redux" example, this appears:
for item in shopping_list:
I read that as "for every instance of item in shopping_list do this:".. but to me that would be strange.. because "item" is never defined before that. In JavaScript (however, I'm not expert in this either) it would be like this:
list1 = ["bananas", "socks", "apples"];
for (item = 0; item < list1.length; item++) {
console.log(list1[item]);
}
There we can see that "item" is defined as 0 and then incremented so that it runs through every index of the list.
It seems ridiculous that I seem to understand a more complicated looking concept than the nice and clean Python way of doing things!
Anyway, I hope that I have explained myself well... thanks!
5 Answers
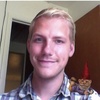
Andrew K
13,774 PointsHi Steven, Your confusion is totally understandable! I will defer to anyone better able to explain it, but basically once you initiate a for loop in python with the word "for", the next word will be interpreted as the singular unit of the iterable object you're iterating over. That word can be anything. Instead of 'item', you could say:
for x in shopping_list:
and get the same results. It just means "for every individual in this list do the following". In the function code, however, you will have to be consistent in using the same word:
for x in shopping_list:
print x
And now I defer to someone else for clarity!

Steven Wheeler
2,330 PointsI think I might have just got it! Maybe...
Maybe I should think that "item" is defined each time it is looped? So if our list is:
exampleList = ["bananas", "apples", "pears"
then "item" (for item in exampleList) is defined as exampleList[0], exampleList[1] and so on? So it does the same thing for each item in our list?
My head hurts :D
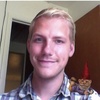
Andrew K
13,774 PointsEssentially, yes!

Ron Fisher
5,752 PointsStephen,
I appreciate your confusion on the iterator concept and I will try to clear it up for you.
Let's use the list you have in your JavaScript example (list1) as our list in Python. Except for the semi-colon in JavaScript they are defined exactly the same.
When the code:
for item in list1: print(item)
is run, the for loop will iterate over every item in the list1. So the first item = "bananas" and the second is "socks" and the third is "apples". In the JavaScript example, you are counting how many items there are and iterating over the list using the index of the item in the list ie. list1[item]. You could do the same thing in Python with:
for num in range(len(list1)): print(list1[num])
range(len(list1)) will create an iterator of [0, 1, 2] and then loop setting the value of num first to 0, then to 1, and last to 2.
I hope this helps clear up the confusion and not create more.
Ron

Steven Wheeler
2,330 PointsYep! That helps a lot, thank you :). I think sometimes I don't trust Python and its lack of syntax and definition, it can confuse me :P.

Ron Fisher
5,752 PointsSteven,
You could think of the Python for item in iterable_item: like the JavaScript arr.forEach(). I'm not a JavaScript expert (yet) but it looks like they are very similar in actions.
Ron

silashunter
Courses Plus Student 162 PointsHow do you call in general that 'thing' ? A container ?
Because for the future, when I will be asking someone about that part of code, which don't need to be extra defined, how to call it ? A random, iteration floating marker.... must have one name in programming, I bet.
Tell me !! :)
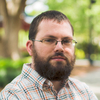
Kenneth Love
Treehouse Guest TeacherI don't really know if it has a name There isn't one in the docs.

silashunter
Courses Plus Student 162 PointsBut you programmers, have to call it somehow. Also in other languages too I guess.
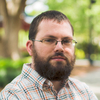
Kenneth Love
Treehouse Guest TeacherMost other languages don't move through loops the same way that Python does. It's really just the current value of the loop.

silashunter
Courses Plus Student 162 PointsA container word is bad for it ?
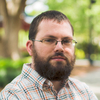
Kenneth Love
Treehouse Guest TeacherIt's not really a container, though. A container would be something that holds other things, like a list or dict or tuple. This is just a place marker.
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherI think that's a solid answer. Thanks, Andrew K !
Steven Wheeler
2,330 PointsSteven Wheeler
2,330 PointsThanks!