Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial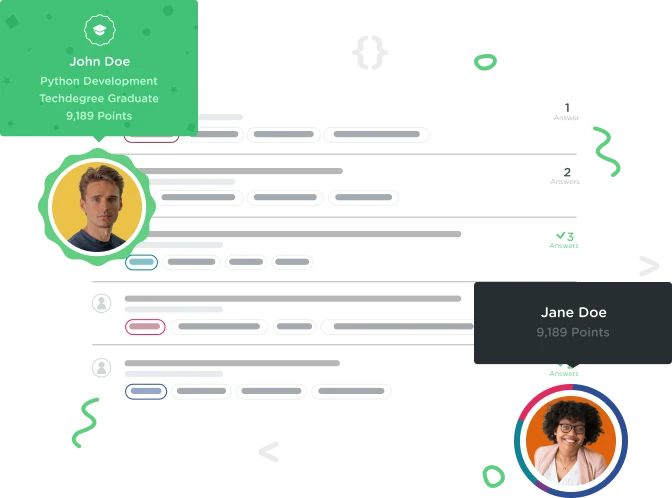

Anthony Lopez
963 Pointsword count problem is hard!
Hey I have really been struggling with this problem. I've been working on it every day and looking back through all of the videos and notes to see if there is something I'm missing.
With what has been taught I have not been able to make a connection on how to make this problem work! I don't want to just google an answer, I'd like to be able to take the knowledge that's been given to me so far to solve it.
I'm having two issues:
I don't know how to make sure that if there is more than one word in the string that only one word gets put into the dict as a key.
I don't know how to make the loop recognize an identical word. If I put value = 0 inside the loop, when it hits another word it resets the count. But if I put value = 0 outside the loop then it will count all the words in the loop as a total number of words in the list.
Would love the help! Thank you.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(sentence):
starting_point = sentence.split()
for word in starting_point:
value = 0
value += 1
final_word = {word: value}
2 Answers
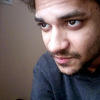
Kunal Gupta
Courses Plus Student 928 PointsTo make sure that the length of string is more than 0 you can add a conditional before the loop.
if len(sentence) > 0:
In your code you need to first declare the variable final_word as an empty dictionary and the value variable before the loop.
value = 0
final_word = {}
for word in starting_point:
Initiate another conditional to check for the if the word already in the dictionary.
if word in final_word: value=final[word]+1
In the above statement we incremented the value of the word to the dictionary. final[word] gives us the value of the key. For example in a Dictionary final = {I:1, Do:1}, final["i"] would gives us a value of 1.
Then we assign the value to the key bt ** final_word[word]=value**
if the word is not in dictionary, for that we use else statement else: ** final_word[word]=1**
Where final_word[word] would assign the value of the key to one
and then return the variable final_word outside of the loop and conditional. return final_word
Here's the code.
if len(sentence) > 0:
value = 0
final_word = {}
for word in starting_point:
if word in final_word:
value = final_word[word]+1
final_word[word]=value
else:
final_word[word]=1
return final_word
You should always look for resources online. I'm not suggesting you to look for the answer, but look for solution to the particular problem in your code, like you did here. There are plenty of resources like stackoverflow where people have already asked the questions you are looking for. :)
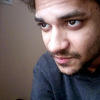
Kunal Gupta
Courses Plus Student 928 PointsI would like to apologize for my carelessness, I've updated my answer above. Please review. :)

Anthony Lopez
963 PointsKunal this is great thank you!
I have two questions though.
When defining value, you are adding final_word[word] + 1. How is it okay to add an integer and a string together and just get the integer as a result?
When you wrote if word in final_word, how is it that you can define a condition before the dictionary is even defined? The condition is searching for word in final_word before you even say that define anything within final_word. Am I understanding that correctly?
Anthony Lopez
963 PointsAnthony Lopez
963 PointsThanks for the post Kunal!
I appreciate the explanation, it helped me to better understand how to put keys and values in dictionaries.
However some of this code still has the same problem. Because value = 0 is outside of the loop. The value variable will hold the number every time it works through the for loop. I end up getting the below dictionary instead. Any suggestions?
{'i': 7, 'do': 2, 'not': 3, 'like': 4, 'it': 5, 'sam': 6, 'am': 8}
Also what is the purpose of if len(sentence) > 0:? I'm still trying to understand that part.