Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial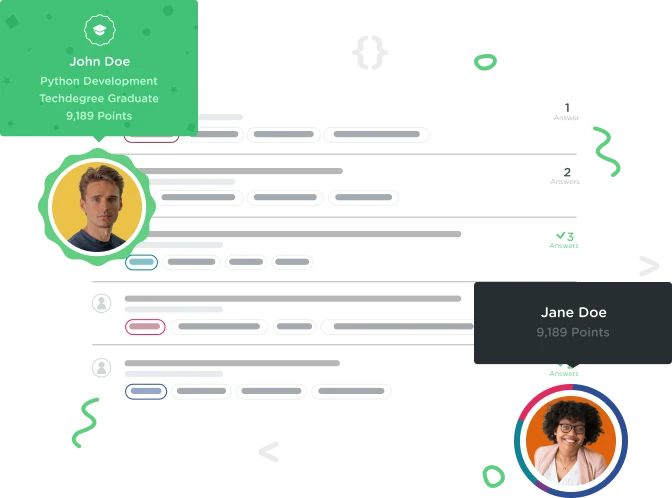

Jean Beltre
1,181 PointsWord Count Python Challenge.
def word_count(string):
new_list=string.split()
unique=set(new_list)
my_dict={}
for unique in new_list:
my_dict[unique]=new_list.count(unique)
return my_dict
Give me your opinions on how you did it
9 Answers
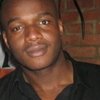
Ogaga Ejuoneatse
Courses Plus Student 2,441 Pointsimport collections
def word_count(word):
new_list = word.lower().split()
return collections.Counter(new_list)
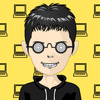
Erion Vlada
13,496 Pointsstring = "erion jade erion jade jade jade erion eleksa eleksa eriona"
string_list = string.lower().split()
string_dict = {}
def word_count(word):
for word in string_list:
if word in string_dict:
string_dict[word] = string_dict[word] + 1
continue
else:
string_dict[word] = 1
return string_dict
dict = word_count(string)
print(dict)
I did it without the use of Counter which Kenneth suggested. I looped through all words (after split to list) and added the word to the dict as a key if they did not already exist with a value of 1. If the key already existed, then I simply added 1 to the value already assigned to the key.
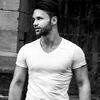
Pablo Xabron
8,111 Pointsdef word_count(string):
words = string.split(' ')
dic = dict('')
for word in words:
dic[word] = dic[word] + 1 if word in dic else 1
return dic
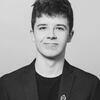
John MacDonald
8,593 PointsI did it like this:
def word_count(string):
word_count = {}
string = string.lower().split(" ")
for word in string:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
print(word_count("I aint no tadpole I is what I is"))

lfkwtz
12,295 Pointsdef word_count(sentence):
sentence = sentence.lower().split()
blank = {}
for word in sentence:
if word not in blank:
blank[word] = 1
else:
blank[word] += 1
return blank
here's mine.
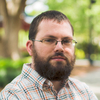
Kenneth Love
Treehouse Guest TeacherLook up Counter
and you'll have even less code :)
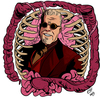
Aleksandre Martashvili
7,373 Pointsdef word_count(string):
split_string = string.lower().split()
my_dict = {}
for word in split_string:
word_occurence = 0
while word in split_string:
word_occurence += 1
my_dict.update({word:word_occurence})
split_string.remove(word)
return my_dict
I didn't know about the Counter or count() so I ended up with this medieval solution

fahad lashari
7,693 PointsThis should pass the challenge:
word_dict = {}
def word_count(string):
string_list = (string.lower()).split()
for item in string_list:
if item in word_dict:
word_dict[item] +=1
else:
word_dict[item] = 1
print(word_dict)
word_count("I am that I am I that am guy guy")

henriqueprimo
3,162 PointsI try to do without a split or Counter. My code end like this (some kind of lines of code could be remove, but i want a new challenge =P)
def word_count(string): new_string = string.lower() list_of_string = [] new_string_to_see = [] for word in new_string: if not word.isspace(): new_string_to_see.append(word)
else:
end_string="".join(new_string_to_see)
list_of_string.append(end_string)
new_string_to_see = []
if not new_string[-1].isspace():
end_string="".join(new_string_to_see)
list_of_string.append(end_string)
count = 0
list_of_string.sort()
words = []
counts = []
i=len(list_of_string)-1
while i>=0:
count = 1
words.append(list_of_string[i])
j = i-1
while list_of_string[i]==list_of_string[j]:
count +=1
j-=1
counts.append(count)
i=j
dictionary = {}
i=0
for string in words:
dictionary[string] = counts[i]
i+=1
return dictionary
string_teste = "This code is so so so long for good sake." resultado = word_count(string_teste) print(resultado)
Elmer Scott
891 PointsElmer Scott
891 PointsLess code:
from collections import Counter
def word_count(word): l_word = word.lower().split() print Counter(l_word)