Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial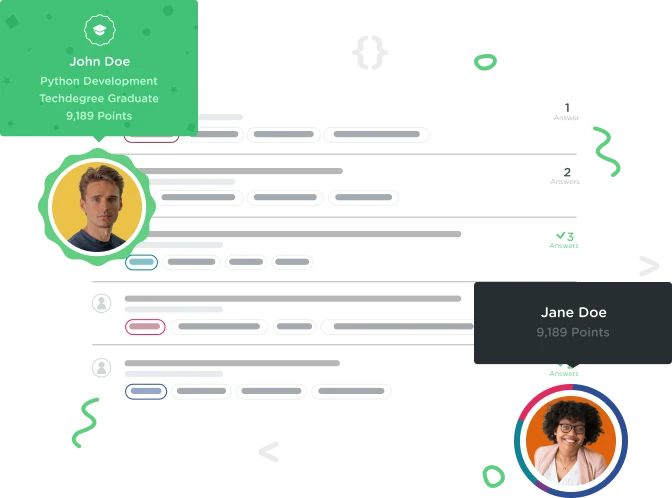

Hank de Roover
1,578 PointsWord count.py
This is not working for me:
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(a_string):
#split the string into a list.
split_string = a_string.split()
string_dict = {}
for word in split_string:
if string_dict[word]:
string_dict[word] += 1
else:
string_dict[word] = 1
return split_string
If anyone has suggestions that would be great.
6 Answers
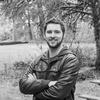
Ryan Ackermann
14,665 PointsHello Hank,
I'll walk you through my solution:
def word_count(string):
d = {}
for char in string.lower().split():
if char in d:
d[char] += 1
else:
d[char] = 1
return d
So first we define a function that intakes one parameter, assuming a string. We then declare an empty dictionary d to store the letters and their occurrence. Next we loop over the string which we lowercase and split the whitespace and assign each iteration to a variable char. Then we check if the letter, represented in char is present in the dictionary d. If our dictionary d contains our value stored in char then we precede to increment the value in "d" for char. If our value stored in char is not in the dictionary we will assign that key a initial value of one, thus instantiating the entry for char. Finally we return the dictionary d.
I hope this helps you & feel free to ask any follow up questions :)

Mark Christian Roma
5,232 PointsI also have another approach for this challenge ...
def word_count(string):
new_dict = {}
new_string = string.lower().split()
for word in new_string:
if word not in new_dict:
new_dict.update({word:new_string.count(word)})
return new_dict

Sylvia Walker-Wavell
2,935 PointsHere's an alternative. It uses the Counter Class.
from collections import Counter
def word_count(a_string):
counted_dict = Counter(a_string.split())
return counted_dict
Hopefully that's not considered a cheat, since Classes haven't been covered in this course; I just thought it was a nice, simple solution :)
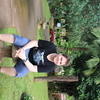
Adi Strasser
3,846 PointsCan you tell me whats wrong here?
Code:
import copy
def word_count(some_string):
some_string = some_string.lower()
some_string = some_string.split(" ")
some_dictionary = {}
broken = copy.copy(some_string)
for word in some_string:
if word in broken:
some_dictionary[word] = 0
while True:
if word in broken:
some_dictionary[word] += 1
broken.remove(word)
continue
else:
break
return some_dictionary

Adam Boyko
1,087 PointsThis took me a bit of brain power, new to python but not as new (just really rusty) for other languages.
I kept running into a simple issue where (before converting the string to count against into a list of its own), count() would count each 'word' literal as a substring in "word_to_count".
where "I like this" would return {"i": 3, "like": 1, "this": 1}. Obviously "I" should've only been equal to 1. The behavior of "count()" is interesting when applied to different classes.
def word_count(word_to_count):
word_dict = {}
for word in word_to_count.lower().split():
if not word_dict.__contains__(word):
word_dict[word] = word_to_count.lower().split().count(word)
return word_dict
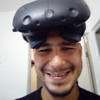
Fernando Alarcon
16,289 PointsI am stuck on this one and don't know why. It outputs the correct dictionary. Here is my code. Any help will be greatly appreciated:
def word_count(word):
wl = word.split(" ")
wc = []
d = {}
i = 0
wl.sort()
while i <= len(wl) - 1:
wc.append(1)
i += 1
i = 0
while i <= len(wl) - 1:
print(wl[i])
if i <= len(wl) - 2:
if wl[i] == wl[i + 1]:
wc[i] += 1
del wc[i + 1]
del wl[i + 1]
i += 1
i = 0
while i <= len(wl) - 1:
d[wl[i].lower()] = wc[i]
i += 1
return(d)
Hank de Roover
1,578 PointsHank de Roover
1,578 PointsThanks man! I was stuck on that for a while and I was trying to figure it out on my own, but sometimes you just need to ask! Thanks a lot man.
-Hank
Ryan Ackermann
14,665 PointsRyan Ackermann
14,665 PointsI totally know what you mean, but I'm glad I could help :)
sandeep krishnappa
1,571 Pointssandeep krishnappa
1,571 PointsHello Ryan, I have a question about your code as I am still quite new to python and hope you don't mind.
my question is that when the code is running through the for loop first time, d is actually an empty dictionary, how can char be able to match with d? Unless all the char has already been allocated in d as keys? Then I don't understand from which line in the code, char has been allocated as keys in d.
Hope my question makes sense. Thanks.
Lucas Diz
4,804 PointsLucas Diz
4,804 PointsThis is great. I was stuck here.
But please, could anyone explain about the (**) double stars? We didnt use it so far.