Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial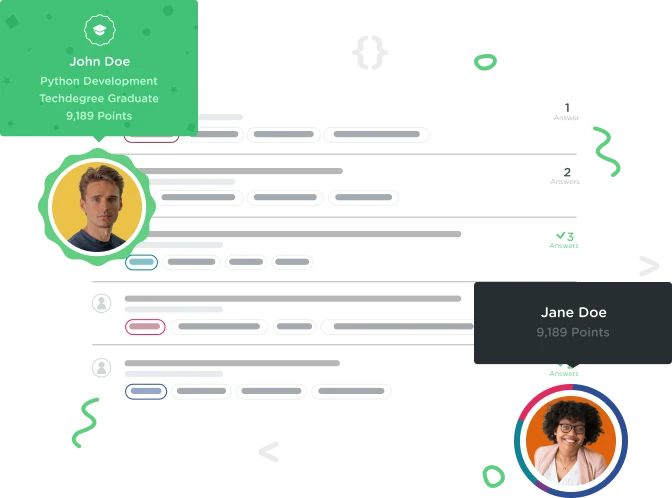

Zach Wright
2,727 Pointswordcount challenge: My code works in the code editor, but not in the code challenge.
I have been using a test case with many different scenarios all of which have worked, though I cannot get the expected output for the code challenge.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(words):
words = removePunctuation(words)
words = words.lower()
i = 0
found = {}
while(i < len(words)):
if((words[i].isspace() or i +1 == len(words)) and i > 0):
word = words[:i]
i += 1
words = words[i:]
i = 0
if(found.get(word) == None):
found[word] = 1
else:
found[word] += 1
elif(words[i].isspace()):
i += 1
words = words[i:]
i = 0
else:
i += 1
return found
def removePunctuation(para):
i = 0
while(i < len(para)):
if(para[i] == '.' or para[i] == ',' or para[i] == '!' or para[i] == ';' or para[i] == ':' or para[i] == '?'):
para = para[:i] + para[i+1:]
i += 1
return para
1 Answer

Cooper Runstein
11,850 PointsYour initial while loop is too long, and too complicated. I'm having a lot of trouble trying to figure out what you're trying to do. You should use a for loop, after all, you're iterating over each word in the sentence which should look like:
for word in words:
dosomething(word)
Further, if you split your words on spaces, you have no reason to use the isspace method. I'm also confused by this line:
elif(words[i].isspace()):
i += 1
words = words[i:]
i = 0
Why are you adding 1 to the i variable, and then resetting it to 0? The i += 1 line isn't ever going to do anything. The biggest problem with this code is it's not very Pythonic, it doesn't flow the way that Python normally flows. I would retry this challenge using only one function, you don't need a separate function for stripping out the punctuation, if you want to abide by the rule "only do one thing per function", create two sub functions within word_count. Also get rid of the while loops, they're making your code really hard to understand and are far less concise when compared to for loops. Lastly, try and avoid having conditions within conditions within conditions; if you can help it don't nest an if/else condition within an if statement within a while loop, it's over complicating your code. Good luck!
Wesley Trayer
13,812 PointsWesley Trayer
13,812 PointsI ran your code and got back the following result:
What I noticed was there is no "am" in the returned dictionary, just "a". Repeated the test with different strings and found the last letter is always removed. That's why it fails the challenge.
Hope this will help you debug it.