Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial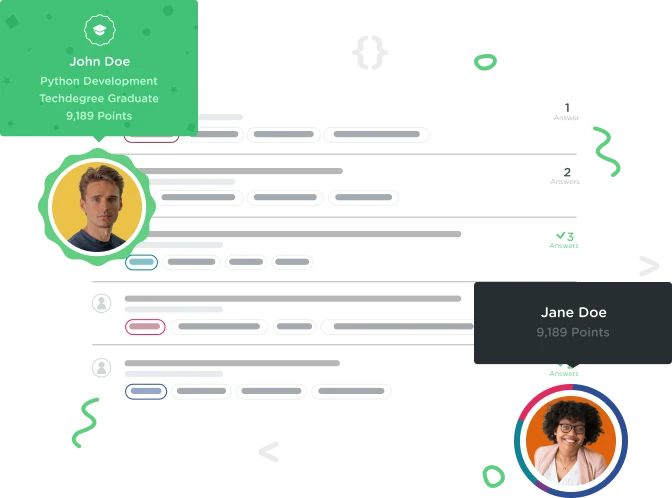

Steven Wheeler
2,330 Pointsword_count() Code Challenge, Python.. really stuck
So the challenge is: "Create a function named word_count() that takes a string. Return a dictionary with each word in the string as the key and the number of times it appears as the value."
I can't think how to increment the number of times it appears.. I know that currently the count will only go up to "2". I've been trying for about 45 minutes now and I just can't figure it out.. -_-.
I understand the flaw in my logic (count is either 1 or count += 1 (2)) but I have no idea how to make that counter only consider each individual instance of each word, rather than considering all instances of all words (if that makes sense!).
def word_count(sentence):
new_sentence = sentence.split(" ")
newDict = {}
for word in new_sentence:
if word in newDict:
count += 1
newDict[word] = count
return(newDict)
word_count("one two two three three three")
6 Answers

mrben
8,068 PointsYou need to change what happens in your if
statement as well as add an else
.
If the word is not in the dictionary you can just set it to 1; otherwise increase it by 1.
Hint: Do away with the count
variable.
Hope that helps!

Ron Fisher
5,752 PointsStephen,
From the code posted above, I don't understand what "new_word" is doing. Do you mean:
for word in new_sentence:
Ron

Steven Wheeler
2,330 PointsYes, apologies, I've updated it now. I tried to make the code a bit easier to read by naming the variables a bit more sensibly but I completely forgot to change that one!
Steve

MUZ140953 Herbert Samuriwo
7,929 Pointsdef word_count(sentence):
new_sentence = sentence.split(" ")
newDict = {}
for word in new_sentence:
if word in newDict:
newDict[word] += 1
else:
newDict[word] = 1
return(newDict)
this work for me,try this one

saro lin
Courses Plus Student 531 PointsThe below code is really simple.
count=dict() a="I am that I am"
def word_count(a): words=a.split() for word in words: count[word]=count.get(word,0)+1 return count

saro lin
Courses Plus Student 531 PointsThe below code is really simple.
count=dict() a="I am that I am"
def word_count(a): words=a.split() for word in words: count[word]=count.get(word,0)+1 return count

saro lin
Courses Plus Student 531 PointsThe below code is really simple.
count=dict() a="I am that I am"
def word_count(a): words=a.split() for word in words: count[word]=count.get(word,0)+1 return count
Steven Wheeler
2,330 PointsSteven Wheeler
2,330 PointsGot it! Thank you! I finally did it -_-
mrben
8,068 Pointsmrben
8,068 PointsCongrats!