Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial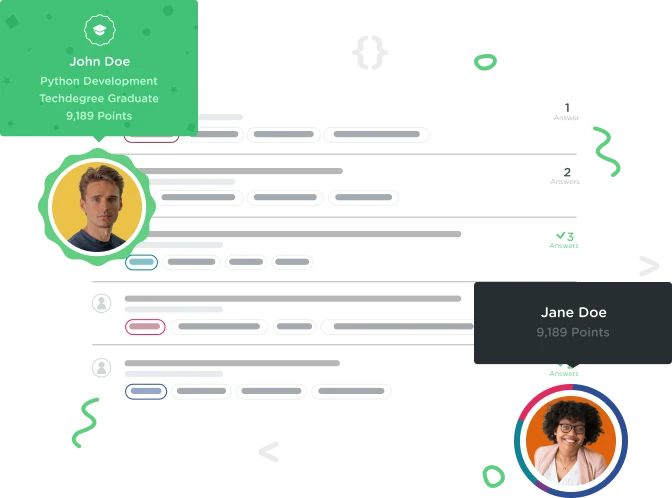

Steve Horton
776 Pointsword_count is causing a few problems!!
This is my code, Any tips!!
wordsList = "I am that I am" wordsList = wordsList.split() print(wordsList)
def wordCount(wordsList): count = 0 key ="" wordCounter ={key: count} for word in wordsList: for key in wordCount: if key != word: wordCount[key]=word wordCount[count]=1 else: count = wordCount.get(key, count) count = count + 1 wordCount.update(key, count) return wordCount
print(wordCount(wordsList))
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
2 Answers
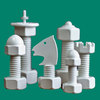
Steven Parker
229,744 PointsIt's a bit hard to read the unquoted code, but it looks like you have a good bit of work to do:
- you don't need to create a test string, the challenge will pass one to the function
- you don't need to print anything
- you only need to define the function, not call it
- the challenge asks for the function to be named "word_count" (not "wordCount")
- you need to declare your return dictionary before you assign anything to it
- you might want to split the string to begin with
- you will probably want to use the challenge's hint about "if word in dict"
- you can manage the counts directly in the dictionary, you won't need a local variable
- the "word" is your key
Hopefully, those hints will help get you on the right track.

Steve Horton
776 PointsThis code below works when I compile it in pycharm, its lists the works and next to a count of how many times the word appears, but in the exercise screen said that some some the words are missing.
wordsList = "I am that I am" wordsList = wordsList.lower() wordsList = wordsList.split()
def word_count(wordsList): word_dict ={} count = 0
for word in wordsList:
if word in word_dict.keys():
count = word_dict.get(word, count)
word_dict[word]=count+1
else:
word_dict[word]=count+1
return word_dict
print(word_count(wordsList))