Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial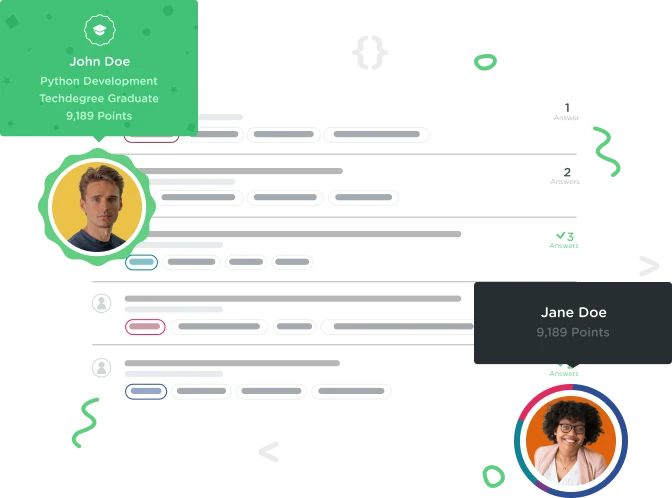

zachary hatsis
1,072 Pointsword_count() problem in python collections. stuck!
I am stuck on this one. How do i increment the key value of a key for how many times it has occured?
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
my_dict = {}
def word_count(mystring):
mystring = mystring.lower().split()
dict_count = 0
for i in mystring:
my_dict[i] = None
if i in my_dict:
my_dict[i] += 1
return my_dict
2 Answers

Frederick Pearce
10,677 PointsThere seems to be an extra loop in there over the dictionary that I suspect is the issue. Hanley Chan's answer should work, or something similar. The code below passed and is pretty compact. Good luck!
def word_count(mystring):
my_dict = {}
for string in mystring.lower().split():
if string not in my_dict:
my_dict[string] = 1
else:
my_dict[string] += 1
return my_dict

Hanley Chan
27,771 PointsHi,
When you loop through your mystring list you could check whether each word is already in your dictionary or not. If yes, increment your counter by one otherwise set it equal to one.
for i in mystring:
if i in my_dict:
my_dict[i] += 1
else:
my_dict[i] = 1

zachary hatsis
1,072 PointsWhen you try to do my_dict[i] += 1 you get:
TypeError: unsupported operand type(s) for +=: 'NoneType' and 'int'

Hanley Chan
27,771 PointsDid you remove the line
my_dict[i] = None
It looks like it's throwing an exception because you are trying to increment None.

zachary hatsis
1,072 Pointsif i remove my_dict[i] = None
it doesn't convert the list in 'mylist' to a dictionary.
zachary hatsis
1,072 Pointszachary hatsis
1,072 PointsThis is getting me closer, however once it iterates through the first 'i am that' it stops iterating over the last 'i am'