Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial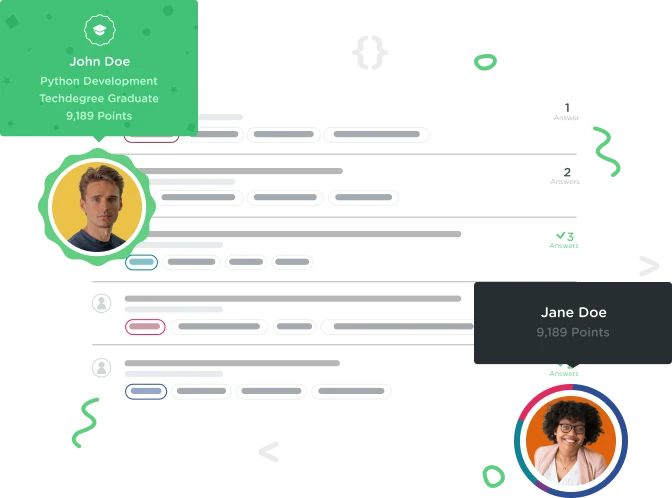

Thomas Katalenas
11,033 Pointsword_count(arg): i cant add to dictionary i tried everything!!?
def word_count(arg): type(arg) == str p = arg.split() count = 0 for i in p: j = p.pop() count += 1 word_dict = dict([(j, count)]) return word_dict
I can not figure this out, big problem plus the poping isn't working right, im sick of it.
my head hurts. can you help me?
4 Answers
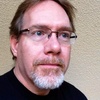
Chris Freeman
Treehouse Moderator 68,460 PointsLet's look at your code first:
def word_count(arg):
# this is a Boolean statement not assigned to a variable
# type checking not necessary for challenge
type(arg) == str
# Split arg into words on whitespace
p = arg.split()
# initialize count to zero
count = 0
# for each item i in wordlist p
for i in p:
# pop item off of p
# this also shortens p causing loop to end sooner
j = p.pop()
# increment count
# count is same as item's index in p
count += 1
# assign a new dict to word_dict
# overwrites word_dict every loop
word_dict = dict([(j, count)])
# return last word_dict
return word_dict
Let now break the task down
# Create a function named word_count()
# that takes a string [as an argument]
def word_count(arg):
# Return a dictionary
word_dict = {}
# with each word in the string
for word in arg.split():
# check if word has been seen (already in dict key list)
if word in word_dict:
# increment count
word_dict[word] += 1
else:
# initialize new word in dict
# word as the key and the number of times it appears as the value
word_dict[word] = 1
return word_dict
The difficulty in using a snippet of code without fully understanding why it works can be confusing when using the same snippet in a different context.
The syntax dict([(j, count)])
is legal, but reassign it to word_list
will overwrite the previously created dictionary.
>>> words = ['first', 'second', 'last']
>>> for word in words:
... pass
...
>>> count = 0
>>> for word in words:
... word_list = dict([(word, count)])
... print(word_list)
... count += 1
...
{'first': 0}
{'second': 1}
{'last': 2}
>>> print(word_list)
{'last': 2}
Instead of overwritting word_list
you can update it with the additional dict:
>>> word_list = {}
>>> count = 0
>>> for word in words:
... word_list.update(dict([(word, count)]))
... print(word_list)
... count += 1
...
{'first': 0}
{'second': 1, 'first': 0}
{'second': 1, 'first': 0, 'last': 2}
>>> print(word_list)
{'second': 1, 'first': 0, 'last': 2}
Note: The above example is using the incorrect count
values from original post.

Clara McKenzie
691 Pointsmy answer was rejected but it works fine in my MacOS intepreter:
def word_count( thestr ):
index=0
mydict={}
mylist=thestr.split()
while (index < len(mylist)):
mydict[ mylist[index] ] = thestr.count(mylist[index])
index += 1
return mydict
[MOD: added ```python formatting -cf]
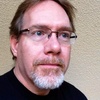
Chris Freeman
Treehouse Moderator 68,460 PointsYou are very close. In your code you need the count on mylist
instead of thestr
:
def word_count( thestr ):
index=0
mydict={}
mylist=thestr.split()
while (index < len(mylist)):
mydict[ mylist[index] ] = mylist.count(mylist[index])
index += 1
return mydict

Thomas Katalenas
11,033 Pointssee the dict([(j, count)]) approach was confusing it was at the bottom of one of the videos dict( key, value)
but it does'nt work for me when I try the increment, it wants to return two values error. So I don't know the Python API is confusing and ambiguous in the docs which is why I really like python syntax is clear and concise but the API documentation is not as transparent as i would like, what do you think? Although I am learning Julia programming it is nice! it clearly helps you learn machine learning algorythms
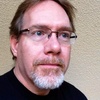
Chris Freeman
Treehouse Moderator 68,460 PointsThe difficulty in using a snippet of code without fully understanding why it works can be confusing when using the same snippet in a different context.
I have updated my answer to add a way to use the dict([(j, count)])
approach.

Thomas Katalenas
11,033 Pointshave you done this before? ;b
Shadow Skillz
3,020 PointsShadow Skillz
3,020 PointsHey Chris just want to thank you for the easy break down I looked over most of the examples but still couldn't wrap my head around the concept but you explained it perfectly thanks