Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial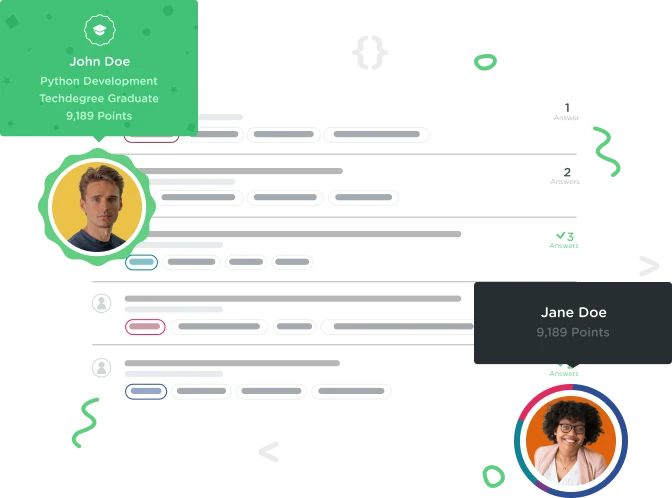

Alexander Lausten
3,223 Pointsword_length.py better aproach?
Hi people ^_^
so i just completed the challenge of word_length.py however in not satisfied with how i ended up doing it and i havent been able to find a better aproach so i tougth it better to ask here! normally when passing a variable to a string we are tought to use .format
so i tried r'\d{}'.format(count) and that returned: r'\d5'
next i tried: r'\d{{}}'.format(count) that return r'\d{{}}'
you can see my solution to the problem in the code but i'd like to khow does anyone know of a better aproach to solve this than using + to glue together strings?
import re
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
def find_words(count, string):
return re.findall(r'\d{'+str(count)+',}', string)
2 Answers

Boris Ivan Barreto
6,838 PointsIf you wanna use str.format statement, you should use a triple square brackets, for instance if you wanna print {4}, you should use {{{0}}}.format(4). So we use this in the return statement as follow
return re.findall(r'\w{{{0},}}'.format(count), string)

Mike Wagner
23,559 PointsIt may not be the best idea, nor am I sure it's "better" per se, but you can also use the old formatting style %
to carry on the format operation. Replacing
return re.findall(r'\d{'+str(count)+',}', string)
with
return re.findall(r'\w{%c,}' % (count), string)
will also yield a passing solution. This way also doesn't require count
to be cast as a string, though you still can to be safe, if you wanted. I'm not sure that it's any more readable with mine than yours or vice versa, but I imagine there isn't much of a difference in performance either.
There might be a better alternative that I can't think of at the moment. I suppose if you wanted to, you could add in extra code and use a recompile call to avoid concatenating strings but, I think, that would probably just make things overly complicated and probably make take up time and energy from both the coder and the program itself. Essentially, it's not really all that bad to concat strings and, if the code is readable and easy to understand, it's probably the best way to do something so trivial.
Mike Wagner
23,559 PointsMike Wagner
23,559 Points+1 - I had forgotten about triple-bracing. Well done.
Lauren Gainsbrook
12,539 PointsLauren Gainsbrook
12,539 PointsHey! I understand how to solve this with formatting.... I just don't know why the third set of braces is needed?! Can you explain?
Mike Wagner
23,559 PointsMike Wagner
23,559 PointsLauren Gainsbrook - I can't explain it as well as Boris Ivan Barreto probably will (since I had forgotten about it entirely) but the addition of the double braces on each side essentially tell it the string literally contains "\w{{0},}" which is almost exactly the same syntax as the old version formatting I showed in my answer. In this case, the {0} is your placeholder object for the .format() functionality.
Boris Ivan Barreto
6,838 PointsBoris Ivan Barreto
6,838 PointsThis is a Python standard string formatting. The format string contain “replacement fields”, which is surrounded by curly braces {}, and anything that is not surrounded by braces is considered as literal text.
However if you want to include the brace character in the literal text, you should escaped by doubling {{ }}.
Back to your question, we need to combine those two concepts in order to obtain the “replacement field” inside curly braces. {{{0}}}, so first two curly braces will print the braces character, and the {0} represents the “replacement field”
More information you can find in in the PEP 3101 -- Advanced String Formatting
Lauren Gainsbrook
12,539 PointsLauren Gainsbrook
12,539 PointsGreat explanation! Thank you so much!