Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial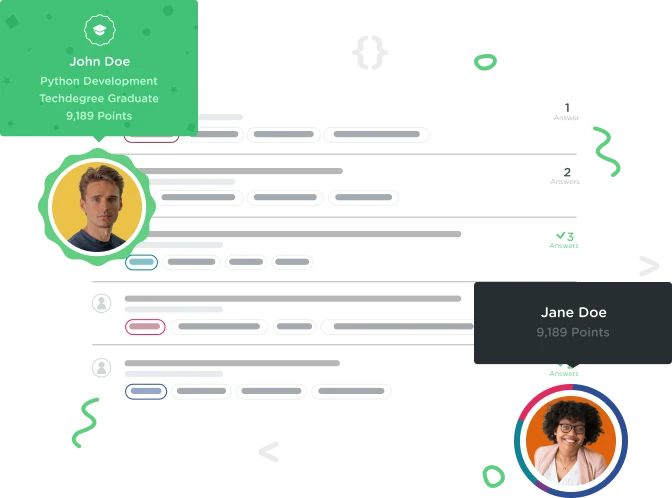

Evan Fraser
6,789 PointsWordPress Company Info Footer Widget - what is load_plugin_textdomain?
I have a couple questions here (I am totally new to this):
- What is the load_plugin_textdomain doing in the plugin?
- Does the register_widget go above or below the widget code?
<?php
/*-----------------------------------------------------------------------------------*
* Hawp Media HCARD Widget
*-----------------------------------------------------------------------------------*/
// Creating the widget
class HAWP_PRIMARY_HCARD_Widget extends WP_Widget {
public function __construct() {
parent::__construct(
// Base ID of the widget
'hawp_primary_hcard_widget',
// Widget name will appear in UI
__( 'HAWP PRIMARY HCARD Widget', 'hawpprimaryhcarddomain' ),
// Widget description
array(
'classname' => 'hawp_primary_hcard_widget',
'description' => __( 'Add the Primary Location HCARD and NAP Schema to this widget.', 'hawpprimaryhcarddomain' )
)
);
load_plugin_textdomain( 'hawpprimaryhcarddomain', false, basename( dirname( __FILE__ ) ) . '/languages' );
}
/**
* Front-end display of widget.
* @see WP_Widget::widget()
* @param array $args Widget arguments.
* @param array $instance Saved values from database.
*/
public function widget( $args, $instance ) {
extract( $args );
$title = apply_filters( 'widget_title', $instance['title'] );
$hclogo = $instance['hclogo'];
$hcbranding = $instance['hcbranding'];
$hccity = $instance['hccity'];
$hcstreet = $instance['hcstreet'];
$hcstate = $instance['hcstate'];
$hczip = $instance['hczip'];
$hcphone = $instance['hcphone'];
$hcurl = $instance['hcurl'];
$hcfax = $instance['hcfax'];
$hclat = $instance['hclat'];
$hclong = $instance['hclong'];
$hcmap = $instance['hcmap'];
$hcmapurl = $instance['hcmapurl'];
$hcmapwdth = $instance['hcmapwdth'];
$hcmaphght = $instance['hcmaphght'];
echo $before_widget;
if ( $title ) {
echo $before_title . $title . $after_title;
}
echo '<div id="hcard-'. (str_replace(' ', '-', $hcbranding)) .'" class="vcard">';
echo '<span id="LocalBusiness-'. preg_replace('/\s+/', '-',$hccity) .'" itemtype="http://schema.org/LocalBusiness" itemscope="" itemprop="parentOrganization" itemref="hours';
if(get_option('hawp_opt_productontology')) { echo ' MORE-SPECIFIC'; }
echo '">';
echo '<span id="'. preg_replace('/\s+/', '-',$hccity) .'-Location">';
echo '<a itemprop="url" class="url" href="'. $hcurl .'"></a>';
echo '<a itemprop="logo image" class="logo photo" href="'. $hclogo .'"></a>';
echo '<span itemprop="name" class="org author fn">'. $hcbranding .'</span><br>';
echo '<span id="'. preg_replace('/\s+/', '-',$hccity) .'-Address" itemtype="http://schema.org/PostalAddress" itemscope="" itemprop="address" class="adr">';
echo '<span itemprop="streetAddress" class="street-address">'. $hcstreet .'</span> <br>';
echo '<span itemprop="addressLocality" class="locality">'. $hccity .'</span>, ';
echo '<span itemprop="addressRegion" class="region">'. $hcstate .'</span> ';
echo '<span itemprop="postalCode" class="postal-code">'. $hczip .'</span><br>';
echo '</span>';
echo '<span itemprop="geo" itemscope itemtype="http://schema.org/GeoCoordinates" class="geo">';
echo '<span itemprop="latitude" content="'. $hclat .'"></span>';
echo '<span itemprop="longitude" content="'. $hclong .'"></span>';
echo '</span>';
if ( $hcphone ) {echo '<div>Phone: <span id="'. preg_replace('/\s+/', '-',$hccity) .'-Phone" itemprop="telephone" class="tel"><a href="tel:'. preg_replace('/\s+/', '',$hcphone) .'">'. $hcphone .'</a></span></div>';}
if ( $hcfax ) {echo '<div>Fax: <span>'. $hcfax .'</span></div>';}
if ( $hcmap ) {
echo '<br>';
echo '<h4>Our Location</h4>';
echo '<a itemprop="hasMap" href="'. $hcmap .'" target="_blank" title="'. $hcbranding .' on Google Maps"><img class="ftr-map-img" src="'. $hcmapurl .'" alt="'. $hcbranding .' on Google Maps" title="'. $hcbranding .' on Google Maps" width="'. $hcmapwdth .'" height="'. $hcmaphght .'"></a>';}
echo '</span>';
echo '</span>';
echo '</div>';
echo $after_widget;
}
/** Sanitize widget form values as they are saved.
* @see WP_Widget::update()
* @param array $new_instance Values just sent to be saved.
* @param array $old_instance Previously saved values from database.
* @return array Updated safe values to be saved.*/
public function update( $new_instance, $old_instance ) {
$instance = $old_instance;
$instance['title'] = strip_tags( $new_instance['title'] );
$instance['hclogo'] = strip_tags( $new_instance['hclogo'] );
$instance['hcbranding'] = strip_tags( $new_instance['hcbranding'] );
$instance['hccity'] = strip_tags( $new_instance['hccity'] );
$instance['hcstreet'] = strip_tags( $new_instance['hcstreet'] );
$instance['hcstate'] = strip_tags( $new_instance['hcstate'] );
$instance['hczip'] = strip_tags( $new_instance['hczip'] );
$instance['hcurl'] = strip_tags( $new_instance['hcurl'] );
$instance['hcphone'] = strip_tags( $new_instance['hcphone'] );
$instance['hcfax'] = strip_tags( $new_instance['hcfax'] );
$instance['hclat'] = strip_tags( $new_instance['hclat'] );
$instance['hclong'] = strip_tags( $new_instance['hclong'] );
$instance['hcmap'] = strip_tags( $new_instance['hcmap'] );
$instance['hcmapurl'] = strip_tags( $new_instance['hcmapurl'] );
$instance['hcmapwdth'] = strip_tags( $new_instance['hcmapwdth'] );
$instance['hcmaphght'] = strip_tags( $new_instance['hcmaphght'] );
return $instance;
}
/** Back-end widget form.
* @see WP_Widget::form()
* @param array $instance Previously saved values from database.*/
public function form( $instance ) {
$title = esc_attr( $instance['title'] );
$hclogo = esc_attr( $instance['hclogo'] );
$hcbranding = esc_attr( $instance['hcbranding'] );
$hccity = esc_attr( $instance['hccity'] );
$hcstreet = esc_attr( $instance['hcstreet'] );
$hcstate = esc_attr( $instance['hcstate'] );
$hczip = esc_attr( $instance['hczip'] );
$hcurl = esc_attr( $instance['hcurl'] );
$hcphone = esc_attr( $instance['hcphone'] );
$hcfax = esc_attr( $instance['hcfax'] );
$hclat = esc_attr( $instance['hclat'] );
$hclong = esc_attr( $instance['hclong'] );
$hcmap = esc_attr( $instance['hcmap'] );
$hcmapurl = esc_attr( $instance['hcmapurl'] );
$hcmapwdth = esc_attr( $instance['hcmapwdth'] );
$hcmaphght = esc_attr( $instance['hcmaphght'] );
?>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('title'); ?>"><?php _e('Title:'); ?></label>
<input class="widefat" id="<?php echo $this->get_field_id('title'); ?>" name="<?php echo $this->get_field_name('title'); ?>" type="text" value="<?php echo $title; ?>" />
</p>
<hr style="width: 109.5%;margin: 20px 0 0 -5%;border-top: 8px solid #e6e6e6;">
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hcbranding'); ?>"><?php _e('Business Name:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcbranding'); ?>" name="<?php echo $this->get_field_name('hcbranding'); ?>"><?php echo $hcbranding; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hclogo'); ?>"><?php _e('Logo URL:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hclogo'); ?>" name="<?php echo $this->get_field_name('hclogo'); ?>"><?php echo $hclogo; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hcstreet'); ?>"><?php _e('Primary Street Address:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcstreet'); ?>" name="<?php echo $this->get_field_name('hcstreet'); ?>"><?php echo $hcstreet; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hccity'); ?>"><?php _e('Primary City:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hccity'); ?>" name="<?php echo $this->get_field_name('hccity'); ?>"><?php echo $hccity; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hcstate'); ?>"><?php _e('Primary State:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcstate'); ?>" name="<?php echo $this->get_field_name('hcstate'); ?>"><?php echo $hcstate; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hczip'); ?>"><?php _e('Primary ZIP:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hczip'); ?>" name="<?php echo $this->get_field_name('hczip'); ?>"><?php echo $hczip; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hcurl'); ?>"><?php _e('Location URL:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcurl'); ?>" name="<?php echo $this->get_field_name('hcurl'); ?>"><?php echo $hcurl; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hcphone'); ?>"><?php _e('Phone #:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcphone'); ?>" name="<?php echo $this->get_field_name('hcphone'); ?>"><?php echo $hcphone; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hcfax'); ?>"><?php _e('Fax #:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcfax'); ?>" name="<?php echo $this->get_field_name('hcfax'); ?>"><?php echo $hcfax; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hclat'); ?>"><?php _e('Latitude:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hclat'); ?>" name="<?php echo $this->get_field_name('hclat'); ?>"><?php echo $hclat; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hclong'); ?>"><?php _e('Longitude:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hclong'); ?>" name="<?php echo $this->get_field_name('hclong'); ?>"><?php echo $hclong; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hcmap'); ?>"><?php _e('Business Map URL:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcmap'); ?>" name="<?php echo $this->get_field_name('hcmap'); ?>"><?php echo $hcmap; ?></textarea>
</p>
<p style="margin:0;">
<label for="<?php echo $this->get_field_id('hcmapurl'); ?>"><?php _e('Map Image:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcmapurl'); ?>" name="<?php echo $this->get_field_name('hcmapurl'); ?>"><?php echo $hcmapurl; ?></textarea>
</p>
<p style="width: 48%;float: left;margin:0;margin-right: 4%;clear:both;">
<label for="<?php echo $this->get_field_id('hcmapwdth'); ?>"><?php _e('Map Image Width:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcmapwdth'); ?>" name="<?php echo $this->get_field_name('hcmapwdth'); ?>"><?php echo $hcmapwdth; ?></textarea>
</p>
<p style="width: 48%;float: left;margin:0;">
<label for="<?php echo $this->get_field_id('hcmaphght'); ?>"><?php _e('Map Image Height:'); ?></label>
<textarea class="widefat" rows="1" cols="20" id="<?php echo $this->get_field_id('hcmaphght'); ?>" name="<?php echo $this->get_field_name('hcmaphght'); ?>"><?php echo $hcmaphght; ?></textarea>
</p>
}
}
/* Register and load the widget */
add_action( 'widgets_init', function(){
register_widget( 'HAWP_PRIMARY_HCARD_Widget' );
});
?>
1 Answer

martinjones1
Front End Web Development Techdegree Graduate 44,823 PointsHi,
Hopefully the information below will help,
1 - load_plugin_domain WordPress codec Essentially this builds a URL that tells WordPress where the multilingual files are stored, so if the plugin is available in other languages these can be loaded.
2 - Don't think this has an impact either way, but I found a good link below that covers both of these topics: WordPress Widget
Evan Fraser
6,789 PointsEvan Fraser
6,789 PointsAwesome thank you! This explains it perfectly!
Evan Fraser
6,789 PointsEvan Fraser
6,789 PointsSo, ill give a little background before my next question. I am using a wordpress theme i bought, basically I am adding a custom widget to display schema info in the site as I dont know how to create my own custom theme yet.
So this is some code i have in a child file in the body of my site:
I am wanting to pull some of the options I made in the widget but it is just not displaying and im thinking its because it's not global. I want to be able to make settings in the widget for the schema and be done, not really have my own options page. So how would I pull $hcbodyschema from the theme widget in the functions.php to my header.php in the body?