Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial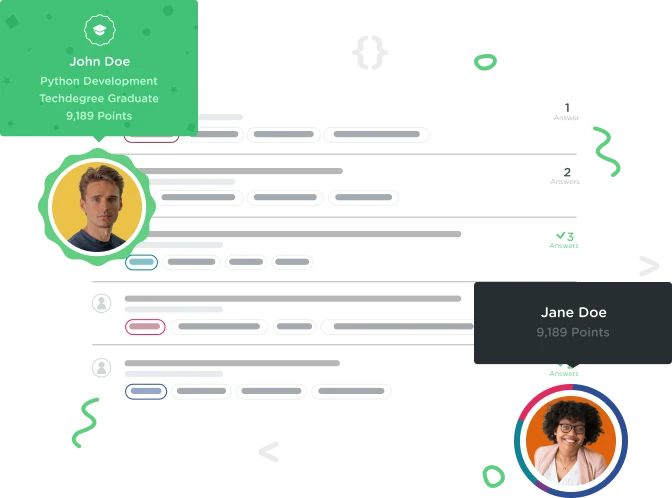
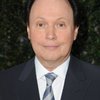
William Lopez
192 PointsWords are not being displayed when I press "Enlighten Me".
package com.william.crystallball;
import java.util.Random;
import android.os.Bundle; import android.support.v7.app.ActionBarActivity; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.TextView;
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declare our View variables and assign them the Views from the layout file final TextView answerlabel = (TextView) findViewById(R.id.textView1); Button getAnswerButton = (Button) findViewById (R.id.button1);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
String[] answers = {
"It is certain",
"It is decidedly so",
"All signs say YES",
"The stars are not aligned",
"My reply is no",
"Better not tell you now",
"It is doubtful",
"Concentrate and ask again",
"Unable to answer now"};
String answer = "";
// Randomly select one of three answers: Yes, No, or Maybe
Random randomGenerator = new Random(); // Construct a new Random number generator
int randomNumber= randomGenerator.nextInt(answers.length);
/* Convert the randomNumber to a text answer
* 0 = Yes
* 1 = No
* 2 = Maybe
*/
answer = answers[randomNumber];
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
this is my code
1 Answer
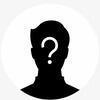
y0mki
14,218 PointsHi!
You need to add a line at the end of onClick(), which updates the answerlabel with the answer that you've set. This is how your code should look like:
getAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
String[] answers = {
"It is certain",
"It is decidedly so",
"All signs say YES",
"The stars are not aligned",
"My reply is no",
"Better not tell you now",
"It is doubtful",
"Concentrate and ask again",
"Unable to answer now"};
String answer = "";
// Randomly select one of three answers: Yes, No, or Maybe
Random randomGenerator = new Random(); // Construct a new Random number generator
int randomNumber= randomGenerator.nextInt(answers.length);
/* Convert the randomNumber to a text answer
* 0 = Yes
* 1 = No
* 2 = Maybe
*/
answer = answers[randomNumber];
answerlabel.setText(answer); // --> This is the magic line :-)
}
});
Hope this helps :)