Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial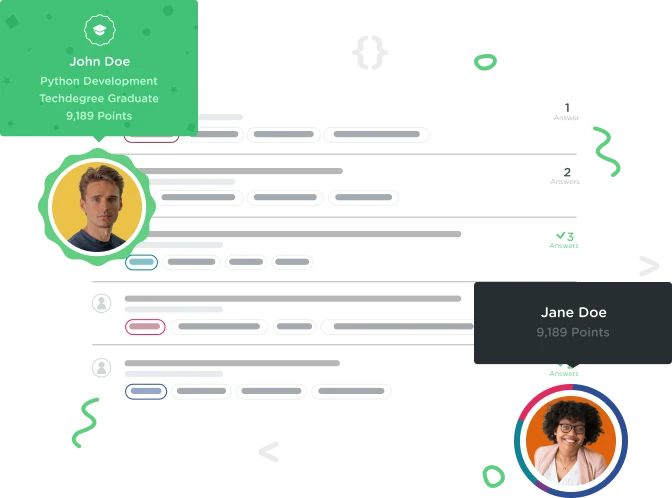
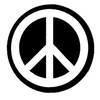
john larson
16,594 PointsWorking on the letter game before watching the whole video. Most things so far I looked up, but this...
So far this code prints out the letters guessed the order they appear in the word that is being guessed EXCEPT if I enter the last letter of the word first. Then the guesses go in out of order. Is this fixable? Should I start over?
#import random library
import random
# list for random choice to pick from
list_of_words = ["itty", "bitty", "teeny", "weenie", "tiny", "tidy" , "whitey"]
# random choice stored in word
word = random.choice(list_of_words)
# transform the random word into a list of letters
word_as_list = list(word)
# print the word as a list
print(word_as_list)
# assemling the new word
new_word_list = []
while True:
# store guessed letter in letter_guess
letter_guess = input("Guess a letter to make the word: ")
# get index value if letter_guess is present
if letter_guess in word:
#store index value of letter_guess in place
place = word.index(letter_guess)
# guess goes into new_word_list at the index and value
new_word_list.insert(place, letter_guess)
print(new_word_list)
if new_word_list == word_as_list:
print("".join(new_word_list))
print("You won!")
break
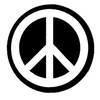
john larson
16,594 PointsHere, you can see I entered the letters out of order, and the code rearranged them to thier propper place according to index
C:\Users\smolderingflax\Python Basics>hello.py
['b', 'i', 't', 't', 'y']
Guess a letter to make the word: i
['i']
Guess a letter to make the word: b
['b', 'i']
Guess a letter to make the word: y
['b', 'i', 'y']
Guess a letter to make the word: t
['b', 'i', 't', 'y']
Guess a letter to make the word: t
['b', 'i', 't', 't', 'y']
bitty
You won!
3 Answers
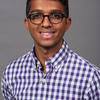
Sanjit Dutta
1,511 PointsI think what's happening has to do with the length of the new_word_list list upon creation. If I guess the sixth letter and I try to put it at index five, you find that it still gets placed at index 0. Now if I guess the fourth letter it will end up placed after the "sixth" letter. I imagine if you initialize new_word_list to be a list of empty strings with a length that is the same as the target word, you won't see this issue any more. For example,
>>>word_list
['b','a','n','a','n','a']
>>>new_word_list
['','','','','','']
>>>new_word_list.insert(5,'a')
>>>new_word_list
['','','','','','a']
Does that make sense? Also right now your code doesn't handle if the same letter appears more than once (list.index() only gives the first instance of the needle).
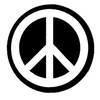
john larson
16,594 PointsThanks Sanjit, I'll take a look at that approach.

fahad lashari
7,693 PointsHi john,
I am having this exact same problem. I posted the question under this video. Did this solution work?
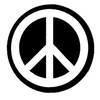
john larson
16,594 PointsHi Fahad. I kinda remember having to take a different approach. I I will look it up and see what I can find. For some reason I can't open that file. But if I remember, the video shows a different approach. Half of the learning it seems like is discovering things that DON'T work. That solution that Sanjit probably worked, but my whole approach was awkward.

fahad lashari
7,693 Pointsok thank you
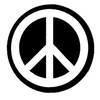
john larson
16,594 PointsFahad, I took a look back through my notes. This particular bit of code needs something that doesn't get covered in the video. Kenneth mentions it after. It's "set". It eliminates duplicate letters. I would love it if you would post your code so I can see what you did so far for the letter game.
john larson
16,594 Pointsjohn larson
16,594 PointsTo make it more confusing (to me anyway), it only seems to do the out of order thing randomly. Sometimes the letters go in the right order even if I type the last letter first. Maybe there's more glitches I just haven't noticed yet.