Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial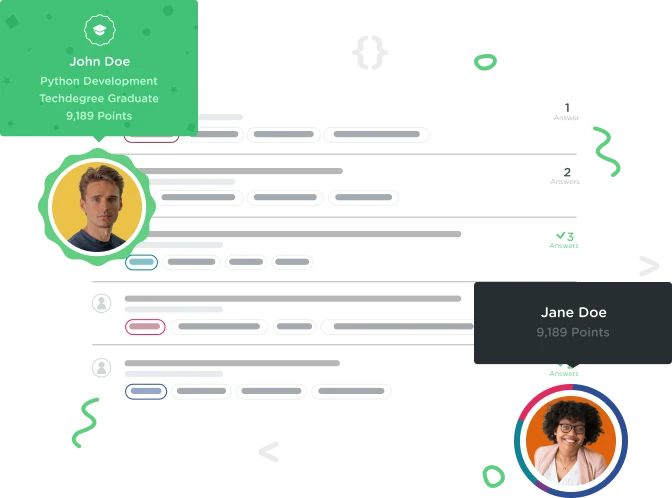

Carson Carbery
9,876 PointsWorking solution in playground doesn't work in code challenge screen
So here's my code again: Seems the solution I came up with, though it works doesn't fit in with the code challenge screen :-/
import Foundation
var randomNumber = Int(arc4random_uniform(UInt32(50)))
let divByThree = randomNumber % 3 let divByFive = randomNumber % 5
if divByThree == 0 && divByFive == 0 { print("FizzBuzz") } else if divByFive == 0 { print("Fizz") } else if divByThree == 0 { print("Buzz") } else { print(randomNumber) }
func fizzBuzz(n: Int) -> String {
import Foundation
var randomNumber = Int(arc4random_uniform(UInt32(50)))
let divByThree = randomNumber % 3
let divByFive = randomNumber % 5
if divByThree == 0 && divByFive == 0 {
return "FizzBuzz"
} else if divByFive == 0 {
return "Fizz"
} else if divByThree == 0 {
return "Buzz"
} else {
return randomNumber
}
return "\(n)"
}
1 Answer
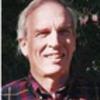
jcorum
71,830 PointsCarson, the Treehouse workspace is quite picky. You can do it "your way" in a Playground. But if you want to complete the challenge you have to do it the way Treehouse asks you to.
A couple of observations though. import statements must be at the very top of the code. You really don't need any random numbers. As he said, he was going to call your function inside a for in loop over a range of 1...100. The function returns a String, so all the return statements have to return Strings. Your last return statement in the if...else... tries to return an Int. I've also found that it really helps, when trying to find errors, to indent at the beginning of each code block, and to outdent at the end of each code block when I'm writing the code.
So here's what they want. You can compare it to yours.
func fizzBuzz(n: Int) -> String {
if n % 3 == 0 && n % 5 == 0 {
return "FizzBuzz"
} else if n % 3 == 0 {
return "Fizz"
} else if n % 5 == 0 {
return "Buzz"
} else {
return "\(n)"
}
}