Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial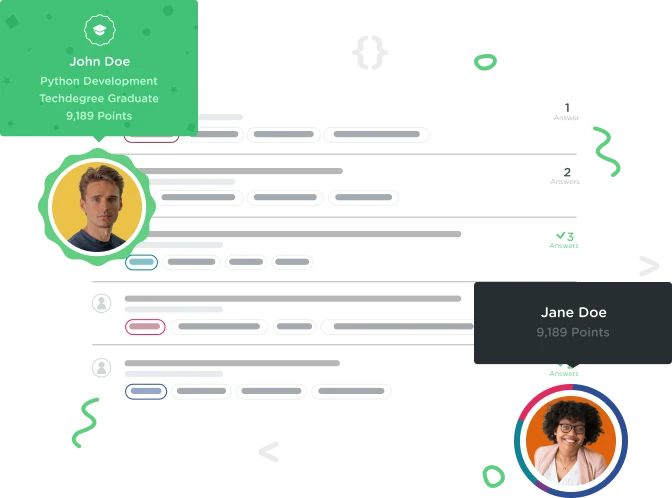
James Moran
7,271 PointsWorking with a Backend dev as a Frontend dev
I am working with a Backend developer on a website, and am wondering what to use instead of JSON/AJAX when a page only requires 1 section to be updated. For example just a username and link to a picture.
Surely, receiving the singular username in JSON is the wrong method for this? Is there an alternative? (maybe plain text??)
Thank you!
2 Answers
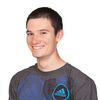
Josh Alling
17,172 PointsIn an ajax call you can use 'html' as the dataType instead of 'json' and it will come over as plain text.
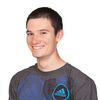
Josh Alling
17,172 PointsThat depends on how your backend is set up. You might be able to add a parameter to the url like '?action=username' to request a username. You could also pass an object that tells what you want as your data in ajax, or if there is a different endpoint for each piece of data then the url itself will let them know. Either way, you will probably just have to sit down with your backend devs and find out how it is set up, or read some documentation.
James Moran
7,271 PointsJames Moran
7,271 PointsGreat! Thank you Josh.
So, I'd have to create a separate AJAX request for each piece of plain text?
As it wouldn't be possible to do something like: $.each(response, function (index, value) {...} Over plain text. Right?
Thanks again!
Josh Alling
17,172 PointsJosh Alling
17,172 PointsYes, you would have to use separate ajax calls, unless you can get all of the data from the same endpoint.
James Moran
7,271 PointsJames Moran
7,271 PointsThanks so much for all of your help. I think I've got it:
But I'm a bit stuck. How will they know what data to give me for each AJAX call?