Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial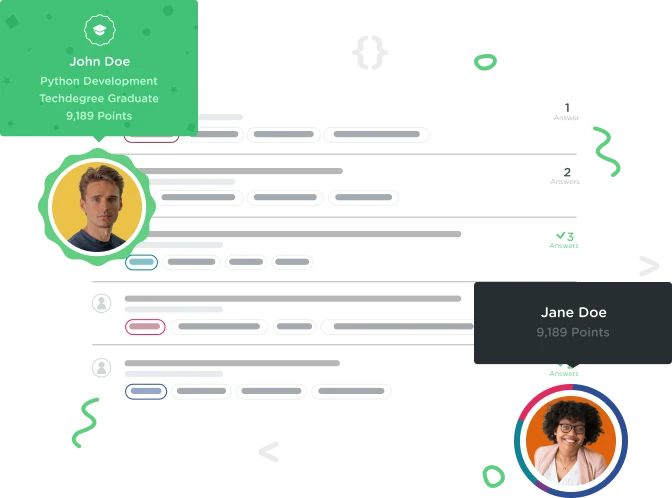

Jorge Grajeda
Python Development Techdegree Student 4,983 PointsWorking with a Plant Database
I keep getting an error message that says "Bummer: Make sure your new_plant variable is inside of the form function's if statement.".
from models import db, Plant, app
from flask import render_template, url_for, request, redirect
@app.route('/')
def index():
plants = Plant.query.all()
return render_template('index.html', plants=plants)
@app.route('/form', methods=['GET', 'POST'])
def form():
if request.method == 'POST':
new_plant = Plant(type=request.form['plant_type'],
status=request.form['plant_status'])
db.session.add(new_plant)
db.session.commit()
return redirect(url_for('index'))
return render_template('form.html')
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8000)
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///plants.db'
db = SQLAlchemy(app)
class Plant(db.Model):
id = db.Column(db.Integer, primary_key=True)
plant_type = db.Column(db.String())
plant_status = db.Column(db.String())
{% extends 'layout.html' %}
{% block content %}
<form action="{{ url_form('form') }}" method="POST">
<label for="type">Plant Type:</label>
<input type="text" name="type" id="type" placeholder="Plant type">
<p>Plant Status:</p>
<label for="good">GOOD</label>
<input type="radio" name="status" id="good">
<label for="ok">OK</label>
<input type="radio" name="status" id="ok">
<label for="bad">BAD</label>
<input type="radio" name="status" id="bad">
</form>
{% endblock %}
{% extends 'layout.html' %}
{% block content %}
<h1>Our Plants:</h1>
<div>
<h2>Plant Type</h2>
<p>Plant Status</p>
</div>
{% endblock %}
1 Answer
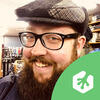
Rohald van Merode
Treehouse StaffHey Jorge Grajeda π
There are a couple things you'll want to adjust to get your code to pass the challenge. First up where you're creating the new plant you're currently passing it the type
and status
properties while looking at your model it's expecting plant_type
and plant_status
properties.
For the values of those properties you're accessing the 'plant_type'
and 'plant_status'
while in the form.html
the name attributes of the input fields are currently set to be type
and status
.
After making both those adjustments your new_plant should look like this:
new_plant = Plant(plant_type=request.form['type'],
plant_status=request.form['status'])
Then for adding and committing this to the database you'll want to call the .add()
and .commit()
methods directly on the db
:
db.add(new_plant)
db.commit()
After these adjustments your code should pass the challenge as expected! Hope this helps! π
Jorge Grajeda
Python Development Techdegree Student 4,983 PointsJorge Grajeda
Python Development Techdegree Student 4,983 PointsI am still receiving the same error message after making these adjustmentsπ