Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial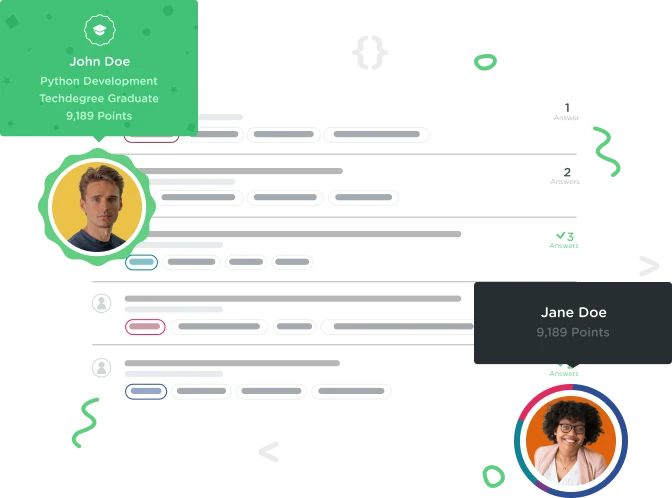

Andrew Dale
Courses Plus Student 7,030 PointsWorking with API's and JSON Objects
I am attempting to create a mobile application that uses an api to display the returned data on the page. So far so good, but now I need to loop through the entire JSON object returned by the XMLHTTPrequest and then display. All of the relevant info on the page.
EG. Here is the JSON object that is returned by the api call:
{
"201511250138DXSLG-34IS5": {
"type": "contact",
"customer_id": "201511250138DXSLG"
},
"201511250138DXSLG-ALA8A": {
"type": "contact",
"customer_id": "201511250138DXSLG"
},
"201511250138DXSLG-KRBIK": {
"type": "contact",
"customer_id": "201511250138DXSLG",
"name": "M D",
"custrole": "owner",
"addresses": {
"FAWSN": {
"address": "email",
"status": "new",
"accountsuppressall": false,
"type": "email"
},
"RQXHS": {
"status": "active",
"address": "+447Tel",
"accountsuppressall": false,
"type": "sms",
"net": 3
},
"AS5F5": {
"status": "active",
"address": "email",
"accountsuppressall": false,
"type": "email"
}
}
},
"201511250138DXSLG-MFTAY": {
"type": "contact",
"customer_id": "201511250138DXSLG"
},
"201511250138DXSLG-UDJG1": {
"type": "contact",
"customer_id": "201511250138DXSLG",
"name": "Andrew Dale",
"custrole": "edit",
"addresses": {
"P1A26": {
"status": "active",
"address": "adale@.co.uk",
"accountsuppressall": false,
"type": "email"
}
}
},
"201511250138DXSLG-ZLGLR": {
"type": "contact",
"customer_id": "201511250138DXSLG"
}
}
This is the function that is connected to my button which sends a GET request to the api and then prints the data to the page:
var getallContacts = function () {
xhr.open('GET', urlGenerator("contacts"), false);
xhr.send(null);
function outputResult(type, customer_id, name, custrole, addresses, address, status, type) {
var response = xhr.responseText;
var contactsArray = JSON.parse(response);
for (var i in contactsArray) {
for (var key in contactsArray[i]) {
/*----------BUILD THE TEXT WRITER--------------*/
document.getElementById("textDiv").innerHTML = "<h1>Contact Information</h1>" + "<div class ='textDiv'>" + "Name: " + contactsArray[i].name + "</br>" + "Contact Type: " + contactsArray[i].custrole + "</br>" + "Customer ID: " + contactsArray[i].customer_id + "</br>" + "Contact Details: " + contactsArray[i][key].address + "</br>" + "Type: " + contactsArray[i][key].type + "</br>";
}
}
}
outputResult();
};
I want to be able to loop over the JSON object and then print all of the relevent data within; "201511250138DXSLG-UDJG1" and "201511250138DXSLG-KRBIK". I have tried a "for(key = 0; key < contactsArray[i].length; key++){" but to no avail.
If any one has any ideas, they would be greatly appreciated.
Thanks
1 Answer
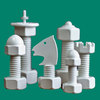
Steven Parker
232,176 Points
It looks like you intermixed two different methods.
If you want to display a fixed set of your own headings with data (or "undefined"), you could do this:
for (var i in contactsArray) {
/*----------BUILD THE TEXT WRITER--------------*/
document.getElementById("textDiv").innerHTML += "<h1>Contact Information</h1>"
+ "<div class ='textDiv'>"
+ "Name: " + contactsArray[i].name + "</br>"
+ "Contact Type: " + contactsArray[i].custrole + "</br>"
+ "Customer ID: " + contactsArray[i].customer_id + "</br>"
+ "Contact Details: " + contactsArray[i].address + "</br>"
+ "Type: " + contactsArray[i].type + "</br>";
+ "</div>";
}
Or if you wanted to display the actual keys present and their contents:
for (var i in contactsArray) {
/*----------BUILD THE TEXT WRITER--------------*/
document.getElementById("textDiv").innerHTML += "<h1>Contact Information</h1>"
for (var key in contactsArray[i]) {
document.getElementById("textDiv").innerHTML += key + ": " + contactsArray[i][key] + "</br>";
}
document.getElementById("textDiv").innerHTML += "</div>"
}
Both of these examples only work with the top-level data, and would need to be enhanced to display nested levels.
Andrew Dale
Courses Plus Student 7,030 PointsAndrew Dale
Courses Plus Student 7,030 PointsBrilliant, thanks Steven. Now all I need to do is access the nested elements of the Object but I'm pretty sure I can get that done myself.
Thanks again.