Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial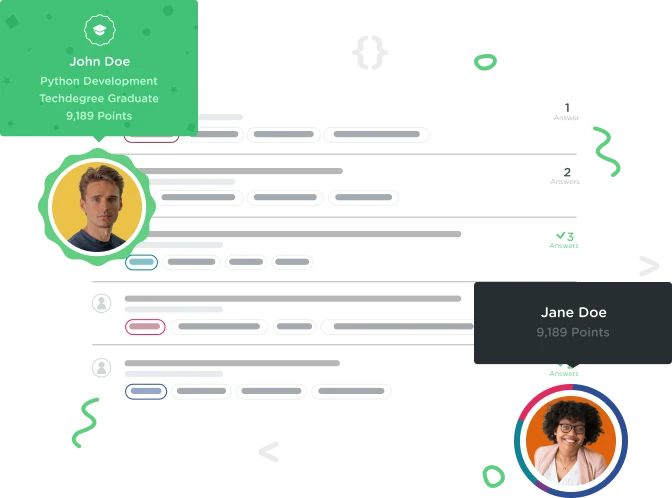

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsWorking with Callbacks, there is an error in my code, any hints or suggestions is very much welcome thank you.
Task:
create a function called filterArray that take 2 arguments:
myArray: this is an array of numbers. isEvenCallBack: this is a function that returns the boolean true if a number is even, and returns false if it's an odd number.
Feedback Make sure you're looping through items in myArray.
Checks Custom Test Incomplete Find even numbers
JS CODE:
/ This is the array that contains numbers for you to work with var myArray = [1, 2, 5, 6, 12, 23, 15, 31];
// This array should only contain even numbers var evenArray = []; function filterArray(myArray, isEvenCallBack) { // TODO: use filterArray to determine if a number is even or odd. //If the number is even, add it to the array 'evenArray' for (let i = 0; i < myArray.length; i++) { if (isEvenCallBack(myArray[i])) evenArray.push(myArray[i]); } return evenArray; }
// This function should return 'true' if a number is even and 'false' if a number is odd function isEvenCallBack(number) { // TODO: use the mod operator (%) to determine if number is even or odd if (number % 2 == 0) return true; else return false; } // This code will call your function when the page loads up // Don't edit this function! window.onload = () => { console.log(filterArray(myArray, isEven)); };
function filterArray(myArray, isEvenCallBack) { // TODO: use filterArray to determine if a number is even or odd. //If the number is even, add it to the array 'evenArray'
return evenArray; }
// This function should return 'true' if a number is even and 'false' if a number is odd function isEvenCallBack(number) { // TODO: use the mod operator (%) to determine if number is even or odd }
// Do not edit code past this point if (typeof module !== "undefined") { module.exports = { filterArray, isEven }; }
Again thank you for your contribution, participation and understanding.