Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial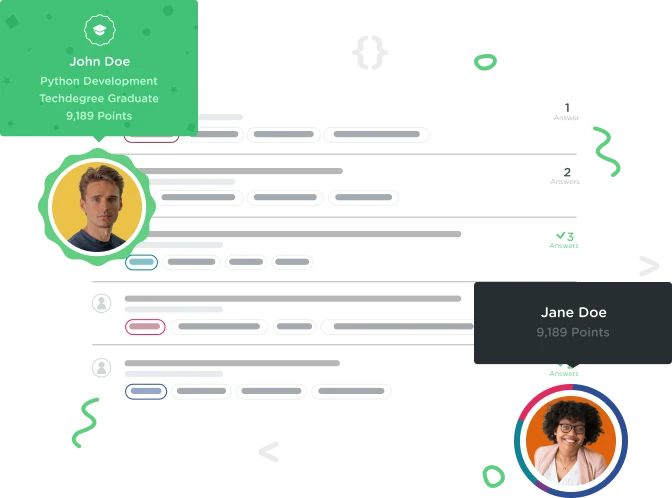
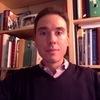
Giovanni Rinaldi
1,104 PointsWorking with Switch statements
I'm stuck with this error... Thanks
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch key {
case "BEL": europeanCapitals.append("Brussels")
case "LIE": europeanCapitals.append("Vaduz")
case "BGR": europeanCapitals.append("Sofia")
case "IND": asianCapitals.append("New Delhi")
case "VNM": asianCapitals.append("Hanoi")
default "USA", "MEX", "BRA": otherCapitals.append("Washington D.C.", "Mexico City", "Brasilia")
}
// End code
}
2 Answers

Addison Francisco
9,561 PointsHi Giovanni,
Your mind is on the right path, but there are some issues with how you've used the switch statement. Also, you are appending the right values into the arrays, but you are not doing it correctly. I'll explain.
For cases where the key is a European country, append the value (not the key!) to the
europeanCapitals
array. For keys that are Asian countries, append the value toasianCapitals
and finally for the default case, append the values tootherCapitals
.
What they are asking is for you to append the value of each key within the world
dictionary to the appropriate "capitals" array. Notice the for-in loop they provided for you has a key
and value
constant defined
for (key, value) in world { ...
For every iteration on the world
dictionary, they key/value constants will hold values for the item it pulled from the dictionary on that iteration. When we look at your implementation, you'll notice it is appending a string literal instead of using the for loop's local value
variable. It works, but it's prone to error because you aren't pulling values from the actual dictionary you are iterating over and instead you have redefined the correct values as new string literals. Also, the compiler will complain that you have an unused local variable (value
). So, you'll want to fix this like so...
for (key, value) in world {
// Enter your code below
switch key {
case "BEL": europeanCapitals.append(value)
case "LIE": europeanCapitals.append(value)
case "BGR": europeanCapitals.append(value)
case "IND": asianCapitals.append(value)
case "VNM": asianCapitals.append(value)
...
Now, everytime the for-in loop iterates on the world dictionary, it will hit the switch statement and evaluate key
, then when a case is matched, it will append the actual value of that dictionary key with the value
variable.
For cases where the key is a European country, append the value (not the key!) to the
europeanCapitals
array. For keys that are Asian countries, append the value toasianCapitals
and finally for the default case, append the values tootherCapitals
.
Again, you're on the right path for what they are asking, but the implementation is incorrect. The other problem is with your switch statement's default
case. Notices what Apple says about default cases...
Every switch statement must be exhaustive. That is, every possible value of the type being considered must be matched by one of the switch cases. If it’s not appropriate to provide a case for every possible value, you can define a default case to cover any values that are not addressed explicitly.
A default
case is the "catch all" for any cases that were not defined in the switch statement. That means you don't have to, nor can you, explicitly define cases to match for a default case. So, let's change that.
for (key, value) in world {
// Enter your code below
switch key {
case "BEL": europeanCapitals.append(value)
case "LIE": europeanCapitals.append(value)
case "BGR": europeanCapitals.append(value)
case "IND": asianCapitals.append(value)
case "VNM": asianCapitals.append(value)
default: otherCapitals.append(value)
}
...
So, now the switch statement will hit the default case for any keys that do not match the other cases you explicitly defined above it without exhaustively defining them yourself. If you did want to exhaustively define the switch cases, you would not use a default
. However, that's not what the challenge is asking for.
Our code should now look like this.
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch key {
case "BEL": europeanCapitals.append(value)
case "LIE": europeanCapitals.append(value)
case "BGR": europeanCapitals.append(value)
case "IND": asianCapitals.append(value)
case "VNM": asianCapitals.append(value)
default : otherCapitals.append(value)
}
// End code
}
I hope this was helpful. I would suggest watching/reading more on control flow. Apple's Swift documentation is always a great place to start.

Ian Billings
Courses Plus Student 7,494 PointsHi Giovanni,
A couple of things I have spotted. You don't need to type the string out, you just need to pass the value to the append, and also you can group the cases together, e.g.
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
This means that if the key is either "BEL", "LIE" or "BGR", the value for that key is appended to the European capitals array. Also, for the default case, you do not need to add the additional cases as default is the 'catch all' case. In other words, if it gets to the default without matching the other cases, thats the one that is run, and again you need to pass the value variable, rather than the string literals. Hope that makes sense.
Ian
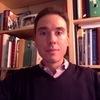
Giovanni Rinaldi
1,104 PointsThanks for your kind reply. I've to study a lot. I'm learning, but it's very hard! Have a good day!
Giovanni
Giovanni Rinaldi
1,104 PointsGiovanni Rinaldi
1,104 PointsYou're great, man! I need to study more and reinforce my learning points. Have a good day, thanks for you reply.
Giovanni