Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial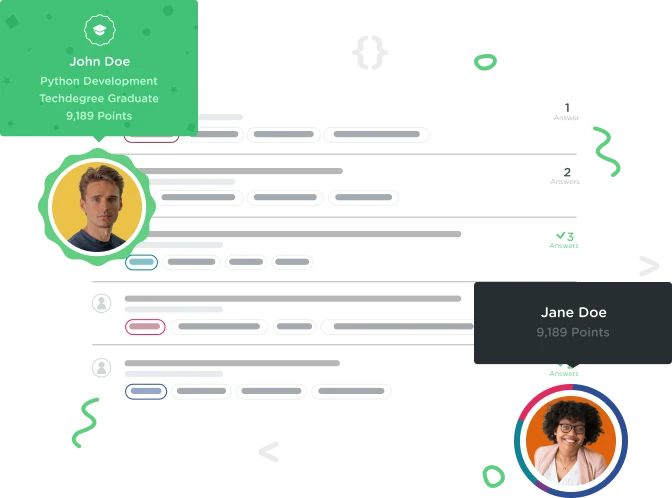

Joakim Vercaemst
9,036 PointsWorks in pycharm but not here
I tried this in pycharm and with dict of multiple keys and values and seems to get it right.
I might not have most elegant code, but it seems to work for me.
Thanks in advance for help :)
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
count = []
def num_teachers(teachers):
for key in teachers:
count.append(key)
return len(count)
count_courses = []
def num_courses(teachers):
values = teachers.values()
for val in values:
count = (len(values))
count += len(values)
return count
3 Answers

Umesh Ravji
42,386 PointsHi Joakim, your code works for the example given, but see what happens when another person is added to the dictionary.
def num_courses(teachers):
values = teachers.values()
for val in values:
count = (len(values)) # need to total length of val, not values
count += len(values) # ??
return count
print(num_courses({'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'], 'Kenneth Love': ['Python Basics', 'Python Collections']}))
# 4 (expected)
print(num_courses({'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'], 'Kenneth Love': ['Python Basics', 'Python Collections'], 'Alena Holligan': ['File Handling with PHP']}))
# 6 (answer should be 5)
What's happening in your code, is that each iteration of the for
loop is replacing the value of count
with the number of values that are in the teachers
dictionary. Then afterwards count
is incremented by the number of values that are in the teachers
dictionary. This appears to give you the correct answer for the first one, but the secound one displays this problem.
def num_courses(teachers):
values = teachers.values()
count = 0 # make sure to initialize this, it may cause you problems if you don't
for val in values:
count += len(val) # length of val (current list)
return count
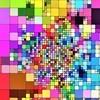
james south
Front End Web Development Techdegree Graduate 33,271 Pointsit works for this example of two teachers with two classes each, but that is just chance. add a third teacher with three classes and you won't get the correct result from the num_courses function. look carefully at the logic - the call to the values method returns 2, because there are 2 keys with 1 value each - a list. it is not reaching the two strings in each list. len(values) is not counting the length of each list, but the length of the return of the values method, which is just the number of keys, since each key has 1 value - a list. so the loop just sets the count variable to the number of keys (note that your count_courses list is unused). then after the loop, count is incremented again by len(values) which again is just the number of keys. so it's just a coincidence that you get 4 with 2 teachers with 2 classes each. a 3rd teacher with 3 classes returns 6 when it should be 7.

Joakim Vercaemst
9,036 PointsOk, now I see, thanks a lot!