Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial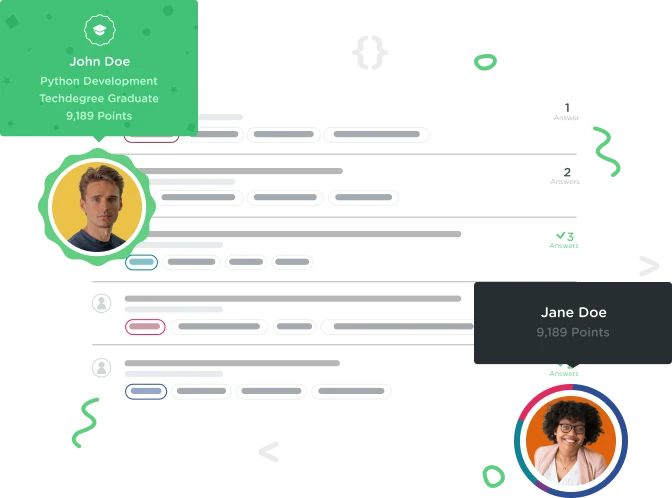

Sang-Ho Park
1,055 Pointsworks on console but still get "Bummer"
My code works in the console but I still get the Bummer message:
def just_right(apples): sin = str(apples) if len(sin) < 5: print("Your string is too short") elif len(sin) > 5: print("Your string is too long") else: return True
def just_right(apples):
sin = str(apples)
if len(sin) < 5:
print("Your string is too short")
elif len(sin) > 5:
print("Your string is too long")
else:
return True
3 Answers

Nicholas Grenwalt
46,626 PointsThere is no need to convert to a string. Your function already expects a string. I believe that is what is throwing it off because you are adding in an unnecessary variable that is being acted upon rather than just using what was passed in directly. Reformat your code so that it directly uses the argument passed in like this and should be good to go:
def just_right(some_string):
if len(some_string) < 5:
return "Your string is too short"
elif len(some_string) > 5:
return "Your string is too long"
else:
return True

Niko Tarmaa
3,038 PointsIthink it might have to be 6 that you compare, since apples is 6 characters long and you're checking if it is longer than 5. You can verify the lenght of the string in console with
def just_right(apples):
sin = str(apples)
print(len(sin))
if len(sin) < 5:
print("Your string is too short")
elif len(sin) > 5:
print("Your string is too long")
else:
return True

Niko Tarmaa
3,038 PointsThis is with the assumption that the apples is actually a string 'apples'

Sang-Ho Park
1,055 PointsI actually answered my own question. It turns out that since the question was asking for certain things to be returned, changing the print command to return solved the problem. I was using the print command since that was as far as the lectures got, and when I tried the return command in the console, I was getting a syntax error, so initially I didn't think that was the solution. But it turned out, that was the answer..
Nicholas Grenwalt
46,626 PointsNicholas Grenwalt
46,626 PointsThe quiz is not asking you to come up with a word to test. It does that when it tests behind the scenes. All you are supposed to do for this particular challenge is create the functionality where any word that gets passed in here would be evaluated. If you actually wanted to call this function with 'apples' as an argument then you'd do so after making the function by passing in 'apples' to just_right like this:
just_right('apples')
Remember, that can only be used after you make the function.
Sang-Ho Park
1,055 PointsSang-Ho Park
1,055 PointsActually, apples was the name of my input variable. And it got to the point that I was checking to make sure the input was str, because none of the simpler assumption worked. But, it seems that the problem was not with the logic, but in using print vs return; return has not been used in the course, so naturally, I was printing the outcomes through print and not return. That solved the problem I was having with the squared exercise as well. Although, when I try to use the return command in the console, I am hit with SyntaxError.