Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial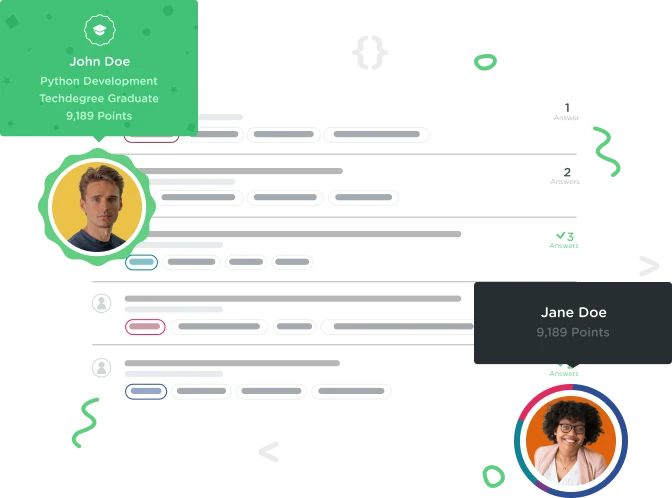
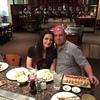
Matthew Earlywine
2,560 PointsWorkspace help
I am not sure what I am missing. I have a lot of errors and when I fix them it doesn't go away, so I am not sure what I am doing wrong.
package com.teamtreehouse;
import com.teamtreehouse.model.Song; import com.teamtreehouse.model.SongBook;
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.HashMap; import java.until.Map;
public class KaraokeMachine { private SongBook mSongBook; private BufferReader mReader; private Map<String, String> mMenu;
public KaraokeMachine(SongBook songBook){ mSongBook = songBook; mReader = new BufferReader(new InputStreamReader(System.in)); mMenu = new HashMap<String, String>(); mMenu.put("add", "Add a new song to the song book"); mMenu.put("quit", "Give up. Exit the program"); }
private String promptAction() throws IOException { System.out.printf("There are %d songs available. Your options are: %n", mSongBook.getSongCount()); for (Map.Entry<String, String> option : mMenu.entry()) { System.out.printf("%s - %s %n", option.getKey(), option.getValue()); } System.out.print("What do you want to do: "); String choice = mReader.readLine(); return choice.trim().toLowerCase(); }
public void run() { String choice = ""; do { try { choice = promptAction(); switch(choice) { case "add": Song song = promptNewSong(); mSongbook.addSong(song); System.out.printf(%s added! %n%n", song); break; case "quit": System.out,println("Thanks for playing!"); break; default: System.out.printf("Unknoown choice: '%s'. Try again. %n%n%n", choice); } } catch(IOException ioe) { System.out.println("Problem with input"); ioe.printStackTrace(); } } while(!choice.equals("quit")); }
private Song promptNewSong() throw IOException {
System.out.print("Enter the artist's name: ");
String artist = mReader.readLine();
System.out.print("Enter the title: ");
String title = mReader.readLine();
System.out.print("Enter the video URL: ");
String vidoeUrl = mReader.readLine();
return new Song(artist, title, videoUrl);
}
}
}
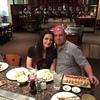
Matthew Earlywine
2,560 PointsSorry, this isn't part of a challenge. I tried to run this and I got a lot off errors. I tried to fix some of the ones I understood, but others I didn't quite get why it was wrong.
Karaoke.java: import com.teamtreehouse.KaraokeMachine; import com.teamtreehouse.model.Song; import com.teamtreehouse.model.SongBook;
public class Karaoke {
public static void main(String[] args) { SongBook songBook = new SongBook(); KaraokeMachine machine = new KaraokeMachine(songBook); machine.run(); } }
SongBook.java:
import com.teamtreehouse.KaraokeMachine; import com.teamtreehouse.model.Song; import com.teamtreehouse.model.SongBook;
public class Karaoke {
public static void main(String[] args) { SongBook songBook = new SongBook(); KaraokeMachine machine = new KaraokeMachine(songBook); machine.run(); } }
Song.java:
package com.teamtreehouse.model;
public class Song { private String mArtist; private String mTitle; private String mVideoUrl;
public Song(String artist, String title, String videoUrl) { mArtist = artist; mTitle = title; mVideoUrl = videoUrl; }
public String getTitle() { return mTitle; }
public String getArtist() { return mArtist; }
public String getVideoUrl() { return mVideoUrl; }
@Override public String toString() { return String.format("Song: %s by %s", mTitle, mArtist); } }
2 Answers
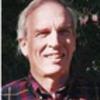
jcorum
71,830 PointsOk, I put your classes into my IDE and tried to compile them. Got lots of errors in KaraokeMachine. And, by the way, SongBook was empty.
I've added a comment each place the compiler found an error, and tried to correct them, if I saw a quick fix:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map; // you had .until. instead of .util.
public class KaraokeMachine {
private SongBook mSongBook;
private BufferedReader mReader; //you had BufferReader instead of BufferedReader
private Map mMenu;
public KaraokeMachine(SongBook songBook){
mSongBook = songBook;
mReader = new BufferedReader(new InputStreamReader(System.in)); //BufferReader again
mMenu = new HashMap();
mMenu.put("add", "Add a new song to the song book");
mMenu.put("quit", "Give up. Exit the program");
}
private String promptAction() throws IOException {
System.out.printf("There are %d songs available. Your options are: %n", mSongBook.getSongCount()); //no getSongCount() method in SongBook
for (Map.Entry option : mMenu.entry()) { //there is no entry() method for HashMaps
System.out.printf("%s - %s %n", option.getKey(), option.getValue());
} System.out.print("What do you want to do: ");
String choice = mReader.readLine();
return choice.trim().toLowerCase();
}
public void run() {
String choice = "";
do {
try {
choice = promptAction();
switch(choice) {
case "add":
Song song = promptNewSong();
mSongbook.addSong(song); //there is no addSong() method in SongBook
System.out.printf("%s added! %n%n", song); //you were missing a " at start of string
break;
case "quit":
System.out.println("Thanks for playing!"); // you had System, instead of System.
break;
default:
System.out.printf("Unknoown choice: '%s'. Try again. %n%n%n", choice);
}
}
catch(IOException ioe) {
System.out.println("Problem with input"); ioe.printStackTrace();
}
} while(!choice.equals("quit"));
}
private Song promptNewSong() throws IOException { //you had throw instead of throws
System.out.print("Enter the artist's name: ");
String artist = mReader.readLine();
System.out.print("Enter the title: ");
String title = mReader.readLine();
System.out.print("Enter the video URL: ");
String vidoeUrl = mReader.readLine(); //vidoeUrl was mis-spelled
return new Song(artist, title, videoUrl);
}
}
//} //you had an extra }
Hope this helps.
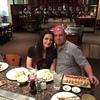
Matthew Earlywine
2,560 PointsI had another question. to post my workshop on here again, how do I make it like how you posted it instead of how I originally posted it.
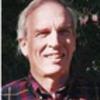
jcorum
71,830 PointsFor any code you post do the following. After a blank line type 3 accent marks (the same key as the ~). On Macs it's just above the ctrl key. Then, on the same line as the 3 accent marks type the kind of code it is: java, swift, php, html, css, etc. Then paste your code on the next line. Then, on the line after your code type 3 more accent marks. Then leave a blank line before typing any comments. It's most important that you have blank lines before and after your code.
See the Markdown Cheatsheet at the bottom of this page for more info.
jcorum
71,830 Pointsjcorum
71,830 PointsMatthew, if this is part of a challenge it would help if you could provide a link. If not, need the Song and SongBook classes in order to test it.