Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial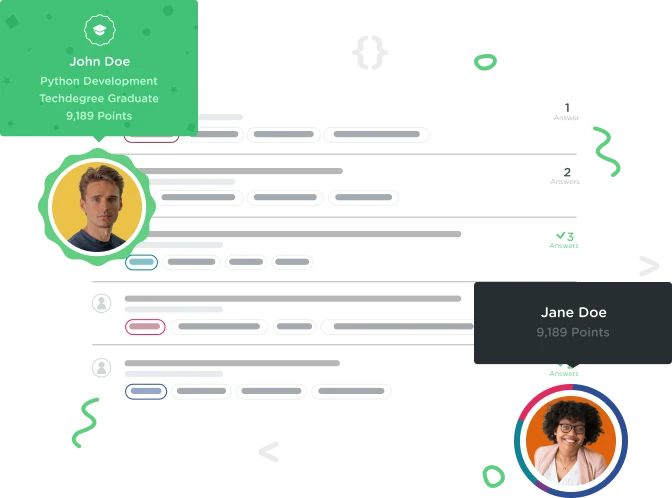

Guillermo Gomez
7,413 PointsWorkspace is retrieving the index.html if the file doesn't exist
When I'm on the Workspace, whenever I type in an unexistent URL, in the main path or in a subfolder, the server retrieve the index.html instead a 404 error. The following is the code from the AJAX Basics Course:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<link href='//fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<title>AJAX with JavaScript</title>
<script>
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function(){
if(xhr.readyState === 4){
if(xhr.status === 200){
document.getElementById("ajax").innerHTML = xhr.responseText;
} else {
alert(xhr.statusText);
}
}
};
xhr.open("GET", "missing.html");
function sendAjax(){
xhr.send();
document.getElementById("load").style.display = "none";
}
</script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Bring on the AJAX</h1>
<button id="load" onclick="sendAjax()">Bring it!</button>
</div>
<ul id="ajax">
</ul>
</div>
</div>
</div>
</div>
</body>
</html>
2 Answers
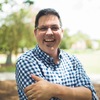
Dave McFarland
Treehouse TeacherThanks for pointing this out. You're right, you literally can't get a 404 error in the workspace right at this moment -- it loads the index.html file if you request a file that doesn't exisit. This is NOT a common behavior -- most web servers DO NOT act this way: they return a 404 if you request a resource that doesn't exist. I believe we are going to change the workspace behavior BACK to returning a 404 error, since that more accurately reflects how it works in the real world.

Guillermo Gomez
7,413 PointsThank you very much for your answer, I've been having troubles with this when I tried to make intentionally lost AJAX calls or when I had some typos.

Iain Simmons
Treehouse Moderator 32,305 PointsIn regards to your question (or statement?), it's probably so that the user can call their base html file anything they want and it will still act like the index page. Otherwise there would need to be some sort of routing rules put in place for each specific Workspace to match the files the user creates.
Treehouse could either:
- Force users to use index.html (making things confusing across different stages in the same course)
- Add an option in the Workspaces to mark a page as the 'home' or 'index' page (adding complexity to the structure of each workspace)
- Leave it as is
Personally, I don't see it as being a big issue, since most users will prefer to see something familiar than a big scary error message or a generic 404 page.
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsFor the code highlighting to work in the forums you'll need to add a new line after the three backticks and
html
before you paste in the code to highlight.e.g.