Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial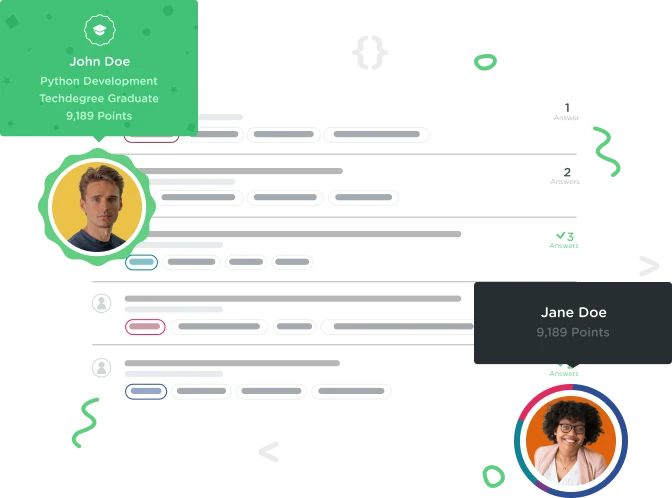

mattmilanowski
15,323 PointsWorkspaces vs Visual Studio Python Extension
I've been using Treehouse workspaces and also Visual Studio Python Extension as a way to understand how Python works out in the 'real world'. This set exercise can be answered in this way:
def covers(x): courses_list = [] for course in COURSES: if x.intersection(COURSES[course]): courses_list.append(course) return courses_list
This works in workspaces, but doesn't work in VS Python. I get an AttributeError: 'str' object has no attribute 'intersection'.
I can get around this error by changing this line: if x.intersection(COURSES[course]): to this - if set(x).intersection(COURSES[course]):
My thinking here was that the error message was telling me that it thinks 'x' is a string. If I wrap it in set() then it will change it's type.
From my solution above, can you tell me how Python knows 'x' is a 'set' type in workspaces? It's not really defined anywhere. I'm mostly a C# guy so this whole thing about not declaring data types has been really throwing me off. Python seems to be the 'Wild West' to me! Anything goes!
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
3 Answers

andren
28,558 PointsWhat type of parameter did you pass to the covers
function when you tested it in Visual Studio? If the answer is a regular string then that would be the reason why it complains about strings not having an attribute intersection.
Since x
just represents the value that was passed in its type will be equal to whatever was passed in as well. While we can't see what code Treehouse uses to call the function it is likely something along these lines:
covers({"strings", "floats"})
By placing the values inside of brackets {} python will treat the values inside as a set. Have you ever used var
to declare a variable in C#? When you use var
C# sets the type of the variable automatically based on the value you assign to it. Python basically does the exact same thing, it just does it automatically behind the scenes for all variables.
If you call your method in Visual Studio with the argument I specified as an example above, then your code should work in visual studio without you having to convert the value to a set.

mattmilanowski
15,323 PointsI just passed in 'Ruby'. Should I have tried to pass {'Ruby'} instead? Something like - print(covers({'Ruby'})? I don't have it in front of me right now but I'll give it a shot.
And good point about using var in C#. I do use it often. That's a good comparison to help me make sense of things.

andren
28,558 PointsYep that's correct. 'Ruby'
is considered a string since it is simply text wrapped by quotes, by wrapping that string in brackets {'Ruby'}
Python will treat it as a set.

mattmilanowski
15,323 PointsI have confirmed that was my problem. Passing covers({'Ruby'}) works and returns 'Ruby Basics'. I guess the part tripping me up was that the challenge doesn't ask you to go as far as passing it a value. You can submit it and it will accept your answer. When I'm testing in my Visual Studio Python extension though I have to pass it a value to verify it works. Thanks for your help Andren