Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial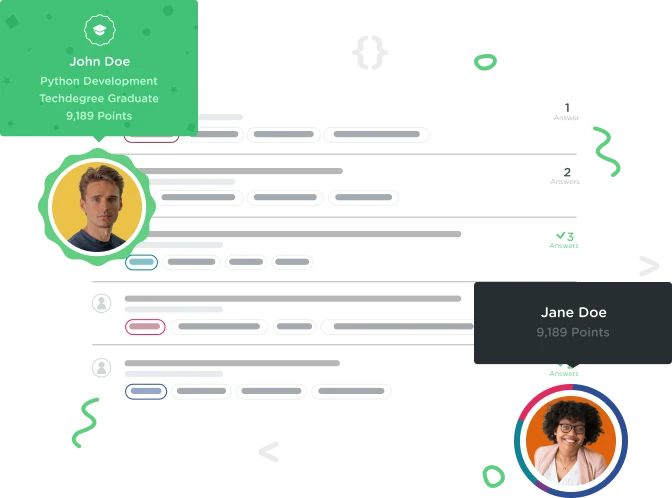

codyperryman3
2,427 PointsWould I move my if statements that are under the flipCoin() function to their own files? or keep in view controller
I am currently going through the beginner classes for Swift. Pasan suggested to create our own apps on our own and recommended a Coin Flip app. Below is my code. Should I create a model for the if statements under the flipCoin() function and have it talk to the view controller or is it ok to have these if statements in the view Controller?
import UIKit
import GameKit
class ViewController: UIViewController {
@IBOutlet weak var headsOrTails: UILabel!
@IBOutlet weak var ifHeads: UILabel!
@IBOutlet weak var ifTails: UILabel!
@IBOutlet weak var flipButton: UIButton!
var backgroundColor = RandomColor()
var coin = CoinFlip()
var headsIndex = 0
var tailsIndex = 0
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
headsOrTails.text = "Press Flip!"
ifHeads.text = "Number of heads: \(headsIndex)"
ifTails.text = "Number of tails: \(tailsIndex)"
}
@IBAction func flipCoin() {
// Random Background Color
let randomBackgroundColor = backgroundColor.randomNumber()
view.backgroundColor = randomBackgroundColor
// Flip Button Color
flipButton.tintColor = randomBackgroundColor
// When the button is pressed, the function pics a random number to
// display either heads or tails and then increments the correct label
// to keep a running total of how many times heads or tails is flipped.
let someCoin = coin.coinFlip()
if someCoin == "Heads" {
headsOrTails.text = "Heads"
headsIndex += 1
ifHeads.text = "Number of heads: \(headsIndex)"
}
if someCoin == "Tails" {
headsOrTails.text = "Tails"
tailsIndex += 1
ifTails.text = "Number of heads: \(tailsIndex)"
}
}
}
2 Answers
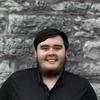
Michael Hulet
47,912 PointsIn a normal iOS app, your model should be holding onto your data and doing most of the real work, and your view controller should just be a bridge between your model and your view (what you display to the user). In this case, you have a CoinFlip
model that appears to be doing all the work of actually choosing what to display, and everything I see in your view controller seems to just be taking the data your model gives you and updating the view, which is exactly what it's supposed to be doing, so I'd say what you have is just fine. That being said, perhaps you could abstract the headsIndex
/tailsIndex
variables into a separate Counter
model?

codyperryman3
2,427 PointsThank you for the help, I appreciate it!