Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial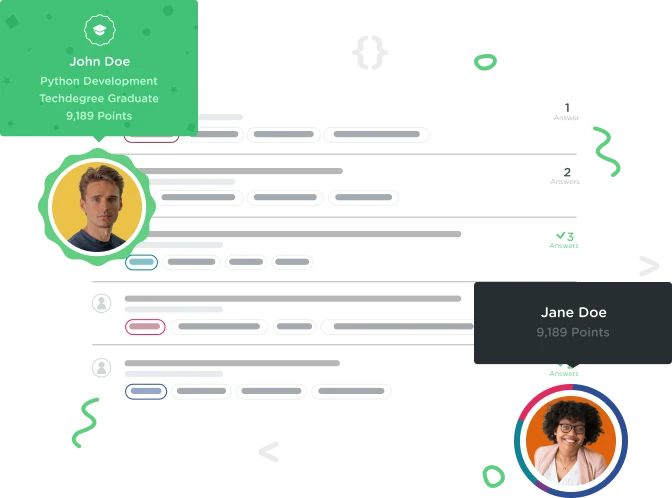
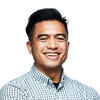
Neil Martin Orbase
4,137 PointsWould my code be considered "lengthy" or acceptable in terms of the goals of "well-written/clean code"?
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
let correctAnswers = 0;
// 2. Store the rank of a player
let rank;
// 3. Select the <main> HTML element
const main = document.querySelector('main');
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
//Questions
const question1 = "Who was the lead singer of Linkin Park?";
const question2 = "What is the name of the operating system for Apple's flagship tablet device?";
const question3 = "What is the full name of Apple's latest and highest tiered smartphone?";
const question4 = "What is the name of Apple's latest 'budget' smartphone?";
const question5 = "What was the name of Apple's original signature digital music player device?";
//Answer Key
const answer1 = 'CHESTER BENNINGTON';
const answer2 = 'IPADOS';
const answer3 = 'IPHONE 11 PRO MAX';
const answer4 = 'IPHONE SE';
const answer5 = 'IPOD';
//If input answer equals stored answer,
const input1 = prompt(question1);
if (input1.toUpperCase() === answer1) {
//Add 1 point,
correctAnswers += 1;
//otherwise,
} else {
//Add 0 points
correctAnswers += 0;
}
const input2 = prompt(question2);
if (input2.toUpperCase() === answer2) {
correctAnswers += 1;
} else {
correctAnswers += 0;
}
const input3 = prompt(question3);
if (input3.toUpperCase() === answer3) {
correctAnswers += 1;
} else {
correctAnswers += 0;
}
const input4 = prompt(question4);
if (input4.toUpperCase() === answer4) {
correctAnswers += 1;
} else {
correctAnswers += 0;
}
const input5 = prompt(question5);
if (input5.toUpperCase() === answer5) {
correctAnswers += 1;
} else {
correctAnswers += 0;
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if (correctAnswers === 0) {
rank = 'No crown';
} else if (correctAnswers >= 1 && correctAnswers < 3) {
rank = 'Bronze';
} else if (correctAnswers >= 3 && correctAnswers < 5) {
rank = 'Silver';
} else {
rank = 'Gold';
}
// 6. Output results to the <main> element
main.innerHTML = `
<h2>You got ${correctAnswers} out of 5 questions correct.</h2>
<p>Crown earned: <strong>${rank}</strong></p>
`;
My solution for this exercise ^
1 Answer
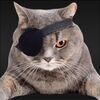
Nicole Antonino
12,834 PointsHey Neil, I can see you put a great deal of thought into the challenge! You are in the JavaScript Basics course, so you will slowly learn how to condense your code as you go along. This is something that comes with time as you practice more and learn all the different JavaScript techniques at your disposal. I would say the code you wrote is certainly well written as you clearly put an effort to make each step be very clear. However, it wouldn't be considered clean. Lots of your code is repetitive, but this is again something you only learn through practice so please don't be discouraged! I won't rewrite your whole code for you, but a little input to make it all less repetitive:
1) It isn't necessary to put each question and answer into their own variables individual variables. All the questions can go into an array with each question as a string value and the same can be done for all the answers.
2) To avoid creating repeating if/else statements, compare the questions with the answers using a nested for loop:
for(let i=0; i < questionsArray.length; i++ {
for (let j=0; j <answersArray.length; i++ {
if (questsionsArray[i] === answersArray[i]) {
correctAnswers ++;
correctAnswersArray.push(questionsArray[i]
}
}
}
This basically does what each of your if/else statements do, but in much less code. Also the else{correctAnswers += 0;}
you're adding to each if statement comparing the inputs isn't necessary. If the input doesn't match the if statement then it just does nothing, so it's not necessary to write that portion.
3) Lastly, you'll see eventually that the comments you are writing are almost too detailed. You won't need to explain the setting of every variable because ideally the variable should have a name that sums up its purpose.
I'm not sure how far along in that JS Basics course you are, but if any of what I wrote is a mystery then don't worry because the JavaScript courses address all these points eventually.