Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial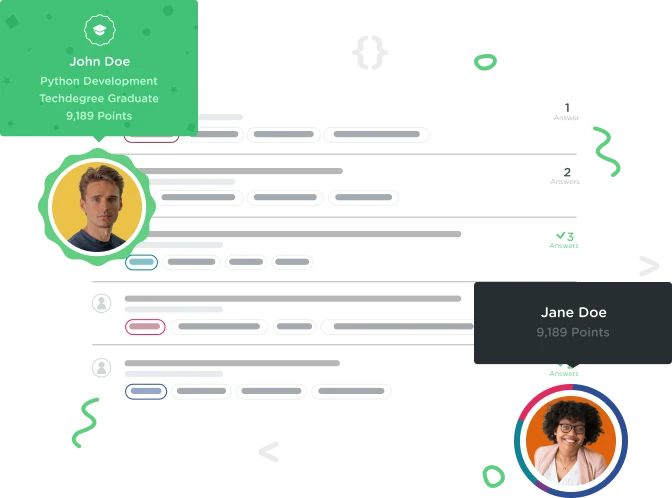
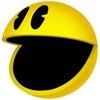
Richard Eldridge
8,229 PointsWould this work?
As much as I appreciate the succinctness of the solution code, and I'm always down for learning new methods, I'm still in a place where I'm trying to cement my understanding of the basics. On that vein, I was wondering if the following would perform the same as the .filter() method mentioned in the solution:
get unusedTokens(){
const tokensRemaining = [];
for (let i = 0; i < this.tokens.length; i++) {
if (this.tokens[i].token.dropped !== false) {
tokensRemaining.push(this.tokens[i]);
}
}
return tokensRemaining;
}
get activeToken(){
return this.unusedTokens[0];
}
I'm not sure if this.tokens[i].token.dropped
is a thing. Plus I'm not sure if creating a new variable tokensRemaining
is necessary. I feel like it might interfere with the activeToken() getter method. I'm so lost...
1 Answer
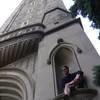
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsHey Richard, it's difficult to know in what context you're using this code and the more succinct solution you are referring to since you have not included them. However, just by examining your code it does look like there would be no reason it would not work fine for your purposes so long as token.dropped
evaluates to a boolean, which I can't be sure of since I cannot see the rest of your code base. Does token.dropped
refer to a method or to a property? is each token itself an object that has a property of dropped with a boolean? Sorry if these questions are silly, I just haven't done the challenge you are working on. Either way, test out your code by trying to run the program and then see if any bugs or errors arise and then if there are, challenge yourself to logically deduce the problem!
Richard Eldridge
8,229 PointsRichard Eldridge
8,229 PointsThis is part and parcel of my frustration. It's the back and forth of classes, properties, and methods referring to each other in multiple ways. It's hard to keep up with even for a small program like this. In this context.
token.dropped
is a property with a boolean value. Each token is created as an object in an arraytokens
in yet a different class. So, my real question really boiled down to my conditional. I'm not sure ifthis.tokens[i].token.dropped
is a valid conditional.tokens
refers to an array created by a method in a class called Player. Within that method, a reference to the Token class is made, and the Token class has property ofthis.dropped
set to a boolean. I got another response that stated my code was correct with the exception of thetoken
. That seems right since each object in the arraytokens
should have the propertydropped
. Basically, according to another helpful user, it should have readthis.tokens[i].dropped
.The exercise is about object oriented programming, which is a new concept to me. I just haven't got my head wrapped around it yet. Well, I guess I kind of get it, sort of, maybe. It just seems....scattered. I don't think I could ever synthesize something new, at least, not yet.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsI know exactly how you're feeling Richard. I got my first intro to object oriented programming back in the spring, while working through a practice project similar to how you are. I was finishing a beginner's book in which the last two chapters guided the reader through the building of the old arcade game Snake. I followed the chapters, studied them, and built the program but I found myself getting very discouraged by the sheer complexity of the program and I got myself to a point of utter confusion several times trying to understand and debug the program on my own. After that experience I didn't touch much OOP for several months until I began to take JS courses on Treehouse. Gradually as JS began to make more sense in general, some of the lessons I learned from that beginner book began to sink in. When I took the OOPJS course on Treehouse I found that it made a lot more sense than the first time I encountered those concepts. and I am now in the final stages of building a full-scale app with OOPJS with very little outside help. Some times you need some initial exposure, a chance to struggle with it, and then a break before coming back to it. Some times difficult concepts need to percolate a bit when you're a beginner.
Also, remember that ideally, the functionality of your program should be broken up into atomistic class methods that each do one single thing. Then, when you start putting them together like a set of Russian dolls, if something doesn't work you don't have to go and re-write your whole code base or even a large part of it, you just need to fix the method that is causing the problem. Any program will inevitably depend on a number of interlocking parts operating together at a higher level, but at the lowest level of the program, each piece of the functionality should be written in such a way that it is independent of all others and can be altered without causing side effects throughout your code base. Then figuring out what is wrong with your program when it doesn't work will be more just a matter of deductive reasoning rather than a nightmare that leaves you pulling out your hair while your brain turns to mush.
For what it's worth, you may get something out of this article: https://medium.freecodecamp.org/how-to-think-like-a-programmer-lessons-in-problem-solving-d1d8bf1de7d2
It has helped me. He talks a lot about breaking down complexity into manageable chunks.
Richard Eldridge
8,229 PointsRichard Eldridge
8,229 PointsI'm sure these are just the trials and tribulations of getting comfortable with these concepts. I can't thank you enough for your tips and encouragement!