Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial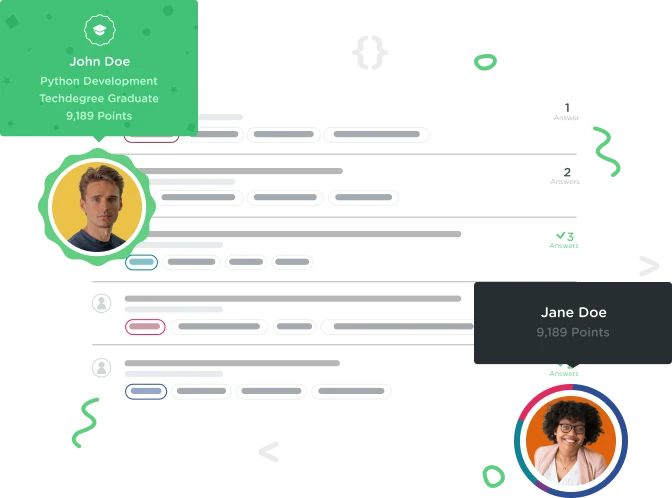

Matthew Stroh
2,287 PointsWouldn't this demonstration be far more useful and effective if you returned an optional Int? in the function?
It seems that a far more practical and understandable way of presenting optionals here is to return an optional Int (Int?
)
A bool is naturally true or false. However, returning, for example, the number of times that it can be divided would be both clearer and more useful.
For example:
func isDivisible(dividend:Int, #divisor Int) -> Int? {
if dividend % divisor == 0 { return dividend / divisor }
else return nil
}
if let result = isDivisible(10, divisor:2){
println("Divisible \(result) times")
}
else {
println("Does not divide evenly")
}
Note: I may not name the function isDivisible
in this case, mind you.
Although you example is correct, an optional int on a function named isDivisible makes less sense than just returning true or false on a standard Bool.
Great course, btw. Nice pace, easy to understand and good intro to the language.
Also, isn't apple recommending that named parameters usually start with the second parameter, unless the purpose is not clear?
1 Answer

Sebastian Röder
13,878 PointsI completely agree. A function isDivisible()
should only return true
or false
:
func isDivisible(dividend: Int, dividedBy divisor: Int) -> Bool {
return dividend % divisor == 0
}
isDivisible(21, dividedBy: 7) // => true
isDivisible(22, dividedBy: 7) // => false
Why would one ever want to return nil
instead of false
? And since we don't have to deal with nil
here in the first place the use case for an Optional does not exist anymore.
As you proposed, an Optional would be much more useful when you want to return the quotient if the dividend is evenly divisible by the divisor or nil
otherwise:
func quotient(dividend: Int, dividedBy devisor: Int) -> Int? {
return dividend % devisor == 0 ? dividend / devisor : nil
}
quotient(21, dividedBy: 7) // => {Some 3}
quotient(22, dividedBy: 7) // => nil