Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial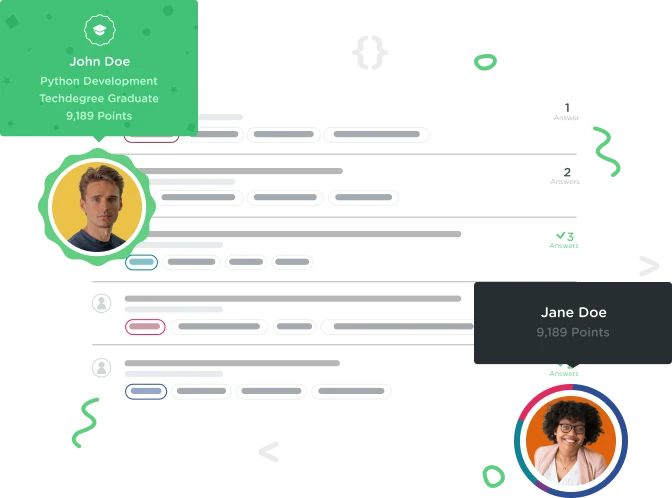
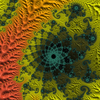
Wes Chumley
6,385 PointsWow! OK, now, create a class named Player that has those same three attributes,
I'm at a complete loss here. Please help and explain. This entire regular expressions lesson has me so confused
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.search(r'''
(?P<last_name>[-\w\s?]*),\s # Last Names
(?P<first_name>[-\w\s?]+):\s # First Names
(?P<score>[\d]+) # Score
''', string, re.X|re.M) # VERBOSE and MULTILINE
class Player:
last_name = str()
first_name = str()
score = str()
def __init__(self, **players.groupdict()):
self.last_name = input('last name: ')
self.first_name = input('first_name: ')
self.score = input('score: ')
4 Answers
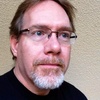
Chris Freeman
Treehouse Moderator 68,441 PointsIt looks like your only missing the proper parameters in the __init__()
method.
class Player:
last_name = ""
first_name = ""
score = ""
def __init__(self, last_name=last_name, first_name=first_name, score=score):
self.last_name = last_name
self.first_name = first_name
self.score = score
# Here is a simplified version that does not set class attributes nor use the class attributes as
# '__init__' defaults. This also passes the challenge:
class Player:
def __init__(self, last_name, first_name, score):
self.last_name = last_name
self.first_name = first_name
self.score = score
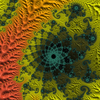
Wes Chumley
6,385 PointsChris,
this does pass the challenge but somehow it just doesn't seem to be exactly what the challenge is asking me to do. Seems more like a workaround to get the output the challenge needs to pass, rather than actually solving the question. Am I wrong?
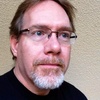
Chris Freeman
Treehouse Moderator 68,441 PointsHey Wes, I've revisited the challenge. I've added a simplified version of Player
that also pases the challenge.

Emmanuel Obi
14,912 PointsThis approach works (in a Python shell), but does not pass on the site for some reason:
class Player():
def __init__(self, string):
attrs = re.search(r'''
^(?P<last_name>[\w\s]+),\s
(?P<first_name>[\w\s]+):\s
(?P<score>[\d]+)$
''', string, re.X|re.M).groupdict()
self.last_name = attrs.get('last_name', '')
self.first_name = attrs.get('first_name', '')
self.score = attrs.get('score', '')
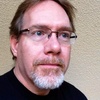
Chris Freeman
Treehouse Moderator 68,441 PointsThe challenge wants to see the re.search expression assigned to the variable players
outside of the class definition.

Emmanuel Obi
14,912 Pointsyeah, Chris Freeman, I figured as much... Come to think of it, my approach is brittle in that control of which attrs get set is based on the string. Not good. I concede that the keywords args are more extensible. I could loop over an array of match groupdicts and pass **kwargs to Player.__init__()
for great good!
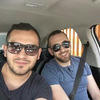
Matija Pildek
8,809 PointsAnother solution.
class Player(): def init(self, **players): for key, value in players.items(): setattr(self,key,value)

Serbay ACAR
147 PointsThis is a full solution for related challenge. In the init definition , this challenge expect from us that we must create a Plater object for each line in string.
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.search(r''' ^(?P<last_name>[-\w\s*\w*]+), \s(?P<first_name>[-\w\s*\w]+): \s(?P<score>[\d]+)$ ''',string, re.X | re.M)
class Player :
last_name = str()
first_name = str()
score = str()
def __init__(self):
for match in players.groupdict():
self.last_name = str(match.last_name)
self.first_name = str(match.first_name)
self.score = str(match.score)

Stephen Lorenz
3,271 Pointscan we use "" here instead of str(), or is there a reason str() is preferable?
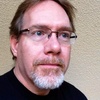
Chris Freeman
Treehouse Moderator 68,441 PointsYes.
>>> str() == ""
True
Wes Chumley
6,385 PointsWes Chumley
6,385 PointsChallenge text :
Wow! OK, now, create a class named Player that has those same three attributes, lastname, first_name, and score. I should be able to set them through _init.