Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial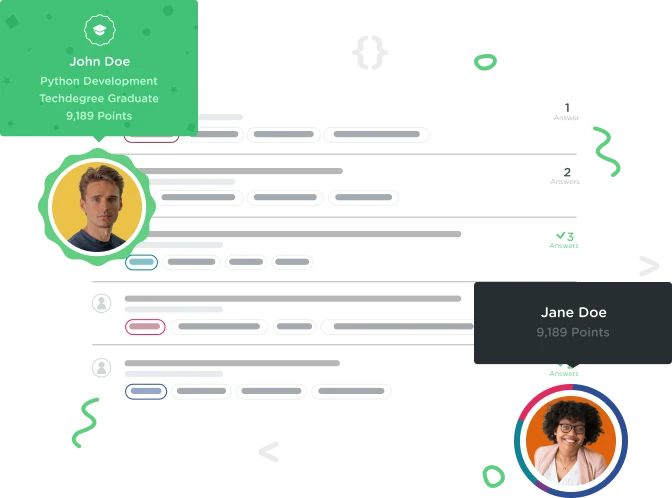
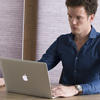
Boris Kamp
16,660 Pointswrap text with <p> tag with jQuery
Hi!
I using advanced custom fields to let users add data, in this case contents of an item. Advanced Custom Fields (wordpress) has no option to wrap each line of a textarea in a tag by default, it does offer the option to convert html code in html, but that means the user must manually wrap all their lines in a <p> tag. What it does offer is to include a <br> tag between all lines which results in this html:
<div class="panel-body side-info">
2 sprues
<br>
2 rubber tires
<br>
test
<br>
test
</div>
now I would like to add a <p> tag around those four lines by default for css purposes, how can I do this?
Thanks!
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsThis may work out for you:
lines = $(".panel-body").html().split("<br>");
$(".panel-body").html('<p>' + lines.join("</p><p>") + '</p>');
It gets the html inside the div and splits it into an array around the <br>
's. Then joins it back together with <p>
tags.
I'm not sure if each of your lines has a trailing and leading space in the actual html or it was just how you posted. The code may have to be updated if you need to get rid of extra spaces.
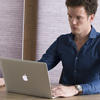
Boris Kamp
16,660 PointsThank you for your help! It's working pretty good! However, I have two post types I want to apply this two, each with three .panel-body divs. When I use your code from above, it copies the html from the first type and pastes it in the other two div's as well. Off course this is not what I want because the content of those div's is different. I fixed this by doing this:
//Wrap side-info lines in <p> tags
//review page template
//Contents review
lines = $(".panel-body.side-info.contents").html().split("<br>");
$(".panel-body.side-info.contents").html('<p>' + lines.join("</p><p>") + '</p>');
//Pros review
lines = $(".panel-body.side-info.pros").html().split("<br>");
$(".panel-body.side-info.pros").html('<p>' + lines.join("</p><p>") + '</p>');
//Cons review
lines = $(".panel-body.side-info.cons").html().split("<br>");
$(".panel-body.side-info.cons").html('<p>' + lines.join("</p><p>") + '</p>');
//finished page template
//Accessories finished
lines = $(".panel-body.side-info.used-accessories").html().split("<br>");
$(".panel-body.side-info.used-accessories").html('<p>' + lines.join("</p><p>") + '</p>');
//Paints finished
lines = $(".panel-body.side-info.used-paints").html().split("<br>");
$(".panel-body.side-info.used-paints").html('<p>' + lines.join("</p><p>") + '</p>');
//Weathering finished
lines = $(".panel-body.side-info.used-weathering").html().split("<br>");
$(".panel-body.side-info.used-weathering").html('<p>' + lines.join("</p><p>") + '</p>');
This works, but only for ONE page template. for example, with this code, it only affects the review page template, and not the finished page template. When I switch them out, the finished page template works, and the review template doesn't How do I fix this? by the way, I get this error in Chrome console: Uncaught TypeError: Cannot read property 'split' of undefined
Thank you very much for your support!

Jason Anello
Courses Plus Student 94,610 PointsOk, I didn't know there was more than one.
We can use the .each()
method to iterate over all 3 div's and run those 2 lines of code for each one. This will remove all the repetition that you have above.
$(".panel-body.side-info").each(function () {
var lines = $(this).html().split("<br>");
$(this).html('<p>' + lines.join("</p><p>") + '</p>');
});
This should get you closer. You won't have the problem of it grabbing the text from one and putting it into all of them.
Other than these 3 div's on the page, are there any other elements on the page that have both a "panel-body" class and a "side-info" class? For the above code to work, you need a selector that will target all 3 div's but not anything else on the page.
Keep checking for that TypeError but I think this code will fix that error.
Let me know how it turns out.
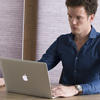
Boris Kamp
16,660 PointsTHANKS! That did the job! I made special ID's for the div's I wanted to target and used your code to make it work! Thank you very much!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Boris,
Is this your goal?