Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial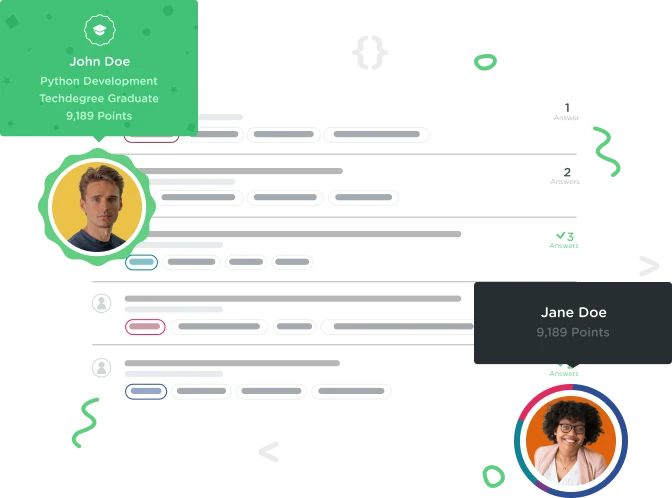

Fahmi Eshaq
3,002 PointsWrite a computed property named Area that returns the area - Help
I keep getting this error: Bummer! Did you compute the area as the square of SideLength?
Where did I go wrong?
using System;
namespace Treehouse.CodeChallenges
{
class Square : Polygon
{
public double SideLength { get; private set; }
public double Area
{
get
{
return Math.Sqrt(SideLength);
}
}
public Square(double sideLength) : base(4)
{
SideLength = sideLength;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Polygon
{
public int NumSides { get; private set; }
public Polygon(int numSides)
{
NumSides = numSides;
}
}
}
4 Answers

andren
28,558 PointsYour code is fine, the problem is your math, square root and square are (despite the name) not the same thing.
To square something means multiplying it by itself, the square root of something is a number which when squared will result in said number.
So 4 squared would be 16 (4*4 = 16), and the square root of 16 would be 4 (16 = 4*4).
So to solve this task you can either return "SideLength * SideLength", or if you prefer using the Math class you can use "Math.Pow(SideLength, 2)" Pow is a "power to" method, and raising a number to the power of 2 is the same as multiplying it by itself.
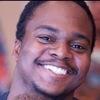
Clinton Johnson
28,714 Pointsusing System;
namespace Treehouse.CodeChallenges
{
class Square : Polygon
{
public double SideLength { get; private set; }
public Square(double sideLength) : base(4)
{
SideLength = sideLength;
}
public double Area
{
get
{
return Math.Pow(SideLength,2);
}
}
}
}
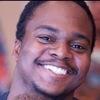
Clinton Johnson
28,714 Pointsandren Thank you so much for your explanation. I have taken the liberty to post the code based off of both your explanation and what i've learned. Hope this helps the next person.
Also for everyone seeing this please review andren overview for explaning how to solve this challenge before looking at the code i posted, because both will help you understand and pass the challenge.
Thank you, Happy coding!

Alwin Landler
1,070 PointsOr if you don't know / don't want to search for the corresponding Math method for this case, you could also keep it as simple as: public double Area { get { return SideLength * SideLength; } }
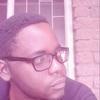
FHATUWANI Dondry MUVHANGO
17,796 Pointsthat definitely helped me Clinton, thank you very much
Carel Du Plessis
Courses Plus Student 16,356 PointsCarel Du Plessis
Courses Plus Student 16,356 Points