Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial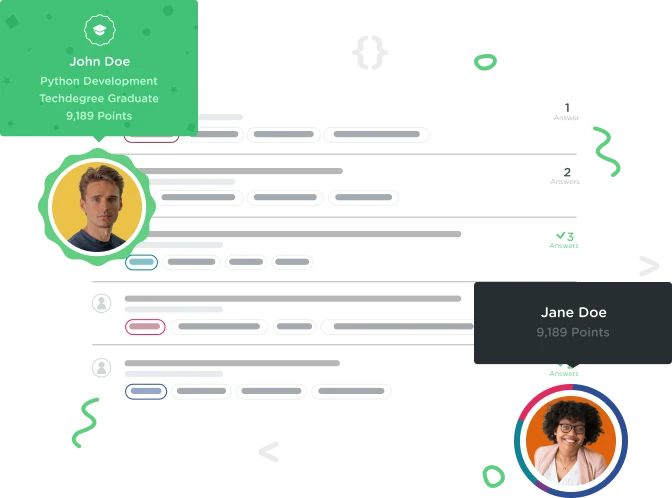

Khaleel Yusuf
15,208 PointsWrite a function named time_machine that takes an integer and a string of "minutes", "hours", "days", or "years". This d
I need so much help.
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
7 Answers
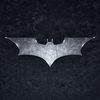
Logan R
22,989 PointsHi!
This challenge took me a second to understand as well when I did it, so no worries.
What it's really saying is that they are going to give you a number and a unit of time. It wants you to add that much time to the starter
variable. So let's say that starter is today and I pass in 5
and days
. It wants you to return the starter date plus 5 days.
So IE:
starter = ...
def time_machine(time, units):
if units == "days":
return datetime.timedelta(days=time) + starter
......
time_machine(5, "days") # Returns the "starter" date + 5 days.
Hopefully this helps you out!
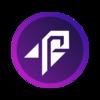
Rocket Dollar Invest
7,129 PointsA simple solution for this problem is to first check if the string is 'years' and if so change the string to 'days' since timedelta will not except years as an argument. Then multiply the integer by 365 to get the equivalent number of days for the given number of years. Finally, return the duration as the difference of the starter datetime and the timedelta of the string and integer passed to the method as a literal dictionary with the ** prefix operator to unpack the dictionary.
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
def time_machine(integer, string):
if string == 'years':
string = 'days'
integer *= 365
return starter + datetime.timedelta(**{string: integer})

Khaleel Yusuf
15,208 PointsWhat do the 6 dots mean?
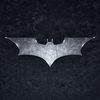
Logan R
22,989 PointsThe dots are just a way to say that there's more code that just isn't shown.

Khaleel Yusuf
15,208 PointsWell, I don't know what to write next.
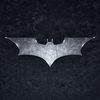
Logan R
22,989 PointsYou need to look at each unit case ("minutes", "hours", "days", and "years") and return the appropriate time delta for each one. In the example code I posted in the original answer, it already has "days" completed.

Khaleel Yusuf
15,208 PointsOK, Thanks!

Khaleel Yusuf
15,208 PointsBut that didn't work.
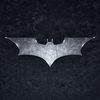
Logan R
22,989 PointsCan you paste in the code you tried to run that didn't work?

Khaleel Yusuf
15,208 Pointsimport datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
def time_machine(time, units):
if units == "days":
return datetime.timedelta(days=time) + starter
else if units == "minutes":
return datetime.timedelta(minutes=time) + starter
else if units == "hours":
return datetime.timedelta(hours=time) + starter
time_machine(5, "days")
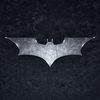
Logan R
22,989 PointsSo you're pretty close. You still need to add the years
block, the else if
should be elif
, and you don't need to call the time_machine (5, "days")
. It will call it automatically. I just added that as an example for what it would look like to call the function.
You're almost there ;) Just don't forget that there is no years
for timedelta. You need to use days and multiply by 365!