Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial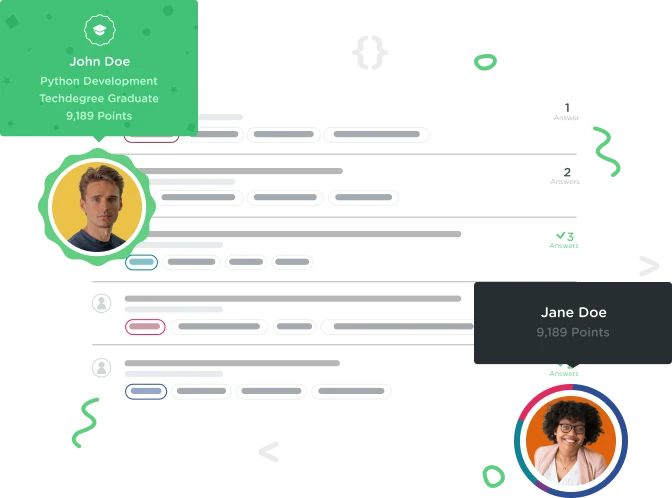
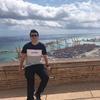
Tadjiev Codes
9,626 PointsWrite a program that asks the user for two integer numbers. Compare the two numbers and output the higher one ????
const higherLowerInteger = integer(prompt("Enter two integer numbers please?"));
function integer(a, b) {
var message = " ";
if (a >= 0 && b >= 0) {
if (a != b) {
message = "The larger number is " + Math.max(a, b);
} else {
message = "Both numbers are equal!";
}
} else {
message = "Please add an integer!";
}
}
console.log(integer(-1, -1));
2 Answers
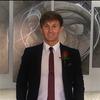
Liam Clarke
19,938 PointsHello
You've almost got it to show your console log, you just need to return the message after the function is run to display it:
function integer(a, b) {
var message = " ";
if (a >= 0 && b >= 0) {
if (a != b) {
message = "The larger number is " + Math.max(a, b);
} else {
message = "Both numbers are equal!";
}
} else {
message = "Please add an integer!";
}
return message; // This bit was missing
}
console.log(integer(2, 2));
However for the prompt, you have 2 options:
- Split the result to get the 2 numbers.
const higherLowerInteger = integer(prompt("Enter two integer numbers please?"));
function integer(numbers) {
const numArr = numbers.split(',');
const a = numArr[0];
const b = numArr[1];
if (a > b) {
return a;
} else if(b > a) {
return b;
} else if (a === b) {
return 'equal';
}
}
console.log(higherLowerInteger);
- Display 2 prompts.
const firstOne = integer(prompt("Enter an integer number please?"));
const secondOne = integer(prompt("Enter another integer number please?"));
function integer(a, b) {
if (a > b) {
return a;
} else if(b > a) {
return b;
} else if (a === b) {
return 'equal';
}
}
console.log(integer(firstOne ,secondOne ));
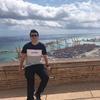
Tadjiev Codes
9,626 PointsHi, I actually came up with another solution ...
var firstOne = integer(parseInt(prompt("Enter an integer number please?"), prompt("Enter another integer number please?")));
function integer(a, b) { var message = " "; if (a >= 0 && b >= 0) { if (a != b) { message = "The larger number is " + Math.max(a, b); } else { message = "Both numbers are equal!"; } } else { message = "Please add an integer!"; } return message; }
console.log(integer(2, 3));
But the output now shows like "The larger number is 3" Why wouldn't it show the real number??? Thanks