Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial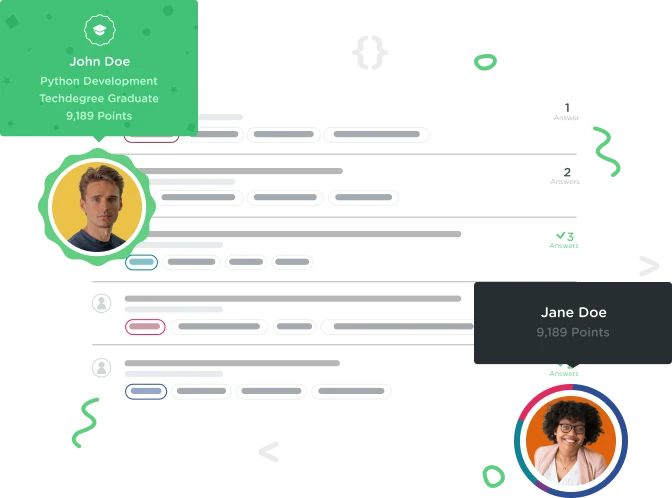
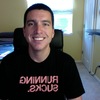
Stephen Printup
UX Design Techdegree Student 45,252 PointsWrite a test spec that proves the clone function in clone.js returns an object where all properties match.
I've looked through the docs and a method that tests properties isn't obvious. Am I supposed to loop through the keys or the values? Am I over thinking this?
var expect = require('chai').expect
describe('clone', function () {
var clone = require('./clone.js')
it('some description string', function () {
// YOUR CODE HERE
expect(clone).to.have.all.keys();
})
})
function clone (objectForCloning) {
return Object.assign({}, objectForCloning)
}
module.exports = clone
2 Answers
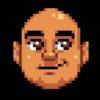
Mario Leiva
45,833 PointsI think this helps
expect(clone({ foo: 1, bar: 2 })).to.have.any.keys('foo', 'baz');
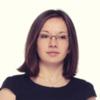
meftimova
Full Stack JavaScript Techdegree Student 8,349 PointsOr expect(clone({ foo: 1, bar: 2 })).to.have.all.keys('foo', 'bar');
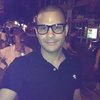
Brian Polonia
25,139 PointsWhat worked for me was:
var expect = require('chai').expect
describe('clone', function () {
var clone = require('./clone.js')
it('some description string', function () {
// YOUR CODE HERE
var obj1 = {foo: 1, bar: 2};
expect(clone(obj1)).to.deep.equal(obj1);
})
})
I created the arbitrary obj1 to pass to clone. I called clone inside expect which creates a clone of the object passed, obj1, and used .to.deep.equal
and passed the obj1 to see if the object and its properties match the clone created by the function clone.
A bit verbose, I know. I'm doing it for my benefit, to get better at explaining these things :)
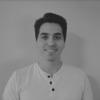
Jean Paul Giraldo
15,701 PointsI tried this exact same thing, but somehow mine didn't pass
Stephen Printup
UX Design Techdegree Student 45,252 PointsStephen Printup
UX Design Techdegree Student 45,252 PointsJoseph Fraley congrats on the treehouse gig! I remember you from PCS!