Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial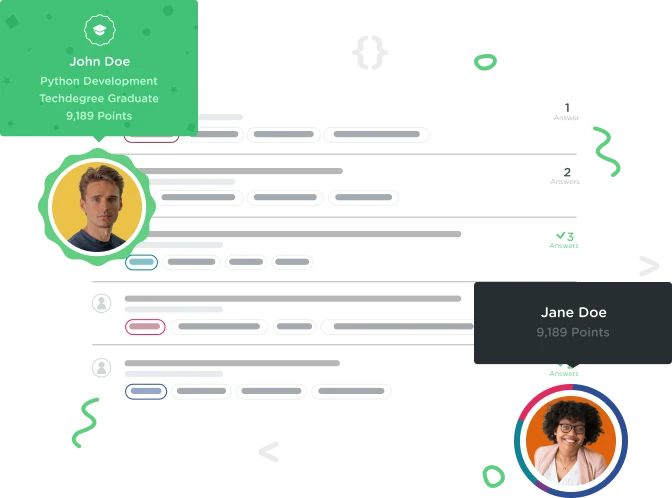
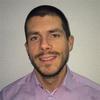
Stamos Bolovinos
4,320 PointsWrite function with less code
In the code challenge 'Returning a value from a function', the following code was used (I have added a document.write):
function getYear(){ var year = new Date().getFullYear(); return year; } var yearToday = getYear(); document.write('<h2 style="text-align: center;">' + yearToday + '</h2>');
I was wondering if this could be written shorter, and have tested it runs also with:
function getYear(){ return new Date().getFullYear(); } var yearToday = getYear(); document.write('<h2 style="text-align: center;">' + yearToday + '</h2>');
It could be written again shorter:
function getYear(){ return new Date().getFullYear(); } document.write('<h2 style="text-align: center;">' + getYear() + '</h2>');
Or even, as the content of the function is nothing else than a builtin function, the custom function to me makes little sense. It could be omitted:
document.write('<h2 style="text-align: center;">' + new Date().getFullYear() + '</h2>');
Any thoughts on this? Or am I wrong?
3 Answers
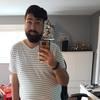
Nourez Rawji
3,303 PointsYou're right, calling
document.write('<h2 style="text-align: center;">' + new Date().getFullYear() + '</h2>');
will accomplish the exact same results as the functions in the challenge. However, I feel like you're missing the big picture of what this challenge is trying to accomplish. The idea here is to learn how functions are defined and how they are called, not to learn how to simply print the current year to a webpage.
The bigger picture here is that you can encapsulate a piece of code in a function to reuse it in your code. Obviously, storing date in a variable then returning it is a little contrived, but imagine if instead you were pulling 20 test scores from a database and averaging them. Obviously, in that case, you could do it all in a condensed set of a few lines as you did with the date above (basically a for loop and a little math), but what happens if you want to calculate the average again later in your program? That's the value of encapsulating data into a function. Reusablity.
So yeah, in the short term this example is a little silly, but when you're doing introductory programming a lot of it seems contrived. But the idea when you're doing the early examples is to simply learn the keywords and what they do. You'll start to see how to use functions and the like in practical cases later on.
P.S. It helps to learn the Treehouse markdown for posting in the community. The way you posted your code in plaintext makes it difficult to read.
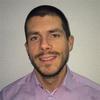
Stamos Bolovinos
4,320 PointsOk, got it - thanks for the markdown hint!
Still need to understand:
What is the advantage of
function getYear(){
var year = new Date().getFullYear();
return year;
}
var yearToday = getYear();
versus
function getYear(){
return new Date().getFullYear();
}
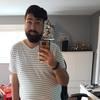
Nourez Rawji
3,303 PointsFunctionally, they're identical. It's purely a stylistic choice. When you eventually get to writing large pieces of code you'll want to try to write in a way that's easiest for you to follow (or, in the real world, the way that your team leader tells you to). Personally, I like doing calculations in a separate variable like in option 1, but in short functions like above it's pretty commonplace to do the calculation and return it in one single line like option 2. The important thing when writing is pick one style and stick with it. Be consistent!
You may want to look up JavaScript arrow functions a little later on when you get comfortable with writing functions. They're a pretty convenient shorthand way of writing out those single like functions like we have here. There's a Treehouse workshop on them, definitely take a look at it if you want to learn more about functions in JS.
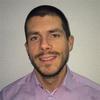
Stamos Bolovinos
4,320 PointsThanks for the detailed answer!