Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial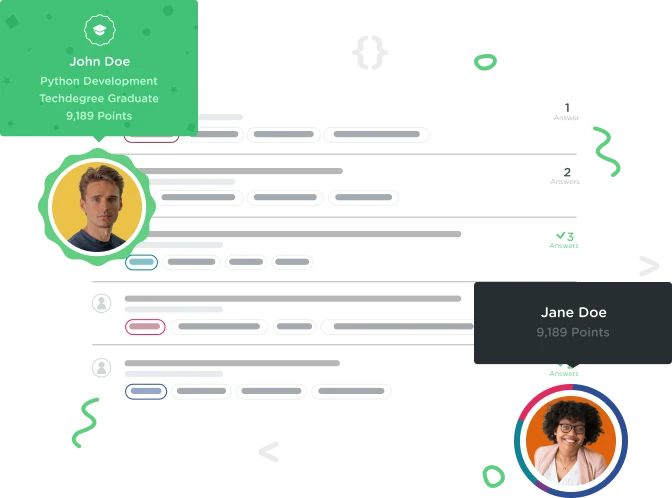

Suleiman Leadbitter
15,805 PointsWrite Our First Tests: Build a Todo List Application with Rails 4 - Rspec Error
Having issues with all this rspec malarkey.
My terminal is saying:
bin/rspec spec/features/todo_lists/create_spec.rb
F
Failures:
1) Creating todo lists redirects to the todo list index page on success
Failure/Error: click_link "New Todo List"
Capybara::ElementNotFound:
Unable to find link "New Todo List"
# ./spec/features/todo_lists/create_spec.rb:6:in `block (2 levels) in <top (required)>'
Finished in 0.75462 seconds
1 example, 1 failure
Failed examples:
rspec ./spec/features/todo_lists/create_spec.rb:4 # Creating todo lists redirects to the todo list index page on success
My create_spec.rb is
require 'spec_helper'
describe "Creating todo lists" do
it "redirects to the todo list index page on success" do
visit "/todo_lists"
click_link "New Todo List"
expect(page).to have_content("New todo_list")
end
end
I'm completely oblivious as to what is going wrong here so any guidance would be greatly appreciated.
Many thanks
6 Answers
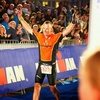
Steve Hunter
57,712 PointsTaking a break sounds wise ... but I'll put an answer together for you to work through later. Feel free to pick this up whenever you're ready - I'm always around to help.
OK, so your error is the following:
expect(page).to have_content("New Todo list") expected to find text "New Todo list" in "New Todo List Title Description Back"
The first bit of this is your test - expect(page).to have_content("New Todo list")
and the reason it failed was because: expected to find text "New Todo list" in "New Todo List Title Description Back".
So, it wanted to find the string "New Todo list" but it found "New Todo List Title Description Back" instead. That just lists all the test on the page that is being displayed. The difference is exactly the same as before - just the other way round!! So, your test is looking for "New Todo list" but the page has on it "New Todo List". You need to be consistent with the capital L between the test and the view.
The test failures are quite verbose so learning to read what it is trying to pass and why it fails is key to fixing the issues. I hope that all helps!
Steve.
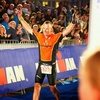
Steve Hunter
57,712 PointsHi Suleiman,
The Ruby on Rails course is a bit of a journey, it has to be said. You will come across all sorts of weird things that aren't immediately clear. Stick at it, Google it, ask in here - you will get there!
In this instance, your test is suggesting that you should click the link called New Todo List. It is failing because there is no link called that.
So, in the View (I'm not sure which one you should be in, to be honest) your link may be called something slightly different?
Let me know and I'll do some more digging!
Steve.
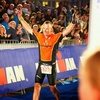
Steve Hunter
57,712 PointsIn my version, for example, the link is "New Todo list" with a non-caps initial on 'list'.
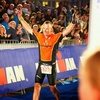
Steve Hunter
57,712 PointsYes, I replicated that error.
In your index.html.erb
file, there's a link declared like:
<%= link_to 'New Todo list', new_todo_list_path %>
or some such. Mine, as above says 'link' not 'Link'. Your test is looking for the capitalised version. Make sure your test and the link are the same else it'll definitely fail.
Did the course suggest it should pass by now? We generally write the test first, fail it, then fix that by adding the correct code.
That's one thing to look at, though, and it is a clear difference between my code and your - and it would cause the failure you are seeing.
Steve.

Suleiman Leadbitter
15,805 PointsMy index.html.erb
is like this:
<p id="notice"><%= notice %></p>
<h1>Listing Todo Lists</h1>
<table>
<thead>
<tr>
<th>Title</th>
<th>Description</th>
<th colspan="3"></th>
</tr>
</thead>
<tbody>
<% @todo_lists.each do |todo_list| %>
<tr>
<td><%= todo_list.title %></td>
<td><%= todo_list.description %></td>
<td><%= link_to 'Show', todo_list %></td>
<td><%= link_to 'Edit', edit_todo_list_path(todo_list) %></td>
<td><%= link_to 'Destroy', todo_list, method: :delete, data: { confirm: 'Are you sure?' } %></td>
</tr>
<% end %>
</tbody>
</table>
<br>
<%= link_to 'New Todo list', new_todo_list_path %>
I changed my create_spec.rb
to have the lower case 'L'
require 'spec_helper'
describe "Creating todo lists" do
it "redirects to the todo list index page on success" do
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
end
end
Although I'm still having the same problem :(
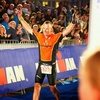
Steve Hunter
57,712 PointsThat looks fine now - did you save the files after you amended them? And is the failure identical?

Suleiman Leadbitter
15,805 PointsYeah, still the same (I think)
bin/rspec spec/features/todo_lists/create_spec.rb
F
Failures:
1) Creating todo lists redirects to the todo list index page on success
Failure/Error: expect(page).to have_content("New todo_list")
expected to find text "New todo_list" in "New Todo List Title Description Back"
# ./spec/features/todo_lists/create_spec.rb:7:in `block (2 levels) in <top (required)>'
Finished in 3.27 seconds
1 example, 1 failure
Failed examples:
rspec ./spec/features/todo_lists/create_spec.rb:4 # Creating todo lists redirects to the todo list index page on success
Randomized with seed 59697
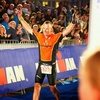
Steve Hunter
57,712 PointsNo - that's a completely different error.
Ignore all the annoying deprecation issues - they're not relevant (and you can silence them too).
The initial error (at the top of the readout) was:
1) Creating todo lists redirects to the todo list index page on success
Failure/Error: click_link "New Todo List"
Capybara::ElementNotFound:
Unable to find link "New Todo List"
That's how I knew it was failing to find a link called "New Todo List". Now, your error is:
1) Creating todo lists redirects to the todo list index page on success
Failure/Error: expect(page).to have_content("New todo_list")
expected to find text "New todo_list" in "New Todo List Title Description Back"
This is because your test is incorrect. You are not expecting the page to have the content "New todo_list" are you? You need to rewrite the test to say that you're expecting the page to contain (or have_content) "New Todo list".
So, your test should be:
require 'spec_helper'
describe "Creating todo lists" do
it "redirects to the todo list index page on success" do
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New Todo list")
end
end
After correcting the first issue, your test successfully clicked through the link, landed on the New Todo List page but then expected to find the test "New todo_list". It didn't find that, so it failed. Revising the test to look for "New Todo List" or "New Todo list" (depends what's in your new.erb.html
- mine has "New Todo List" in the h1 elements).
That should then pass the test and you're a step closer to completing the app!
I hope that makes sense - shout if not.
Steve.
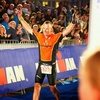
Steve Hunter
57,712 PointsYours is capitalised too - the test failure shows us that as it says it found "New Todo List Title Description Back" when it failed to find "New todo_list"
I've deleted all the deprecation warnings to make that all more readable. You can see what the errors are saying to you, that way.

Suleiman Leadbitter
15,805 PointsSorry Steve,
You are being very very patient and helpful. I'm a bit mashed at the moment and fasting whilst coding (something new) is never a good idea :/ That and the new Dragons cartoon from Netflix is on the TV to distract me. :P
Failures:
1) Creating todo lists redirects to the todo list index page on success
Failure/Error: expect(page).to have_content("New Todo list")
expected to find text "New Todo list" in "New Todo List Title Description Back"
# ./spec/features/todo_lists/create_spec.rb:7:in `block (2 levels) in <top (required)>'
This is the new problem. I'm determined to get this sorted so I can progress through the Rail course :)

Suleiman Leadbitter
15,805 PointsI think I'll take a breather and then come back to this later.
Suleiman Leadbitter
15,805 PointsSuleiman Leadbitter
15,805 PointsI'm really sorry and obviously totally naive to this type of coding but I've looked over my code for the lowercase and uppercase 'L' but I have no idea where or what needs changing :(
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsOK - your test is looking for the text "New Todo list" contained in the page
new.html.erb
- it is finding "New Todo List".I'm sorry - I didn't make that clear. The test clicks through from
index.html.erb
(the first failure, remember?) with the 'New Todo List' link which routes you to thenew.html.erb
page.In there, the test is looking for the text "New Todo list" which isn't there because it is currently "New Todo List". So that needs amending from 'List' to 'list'. (Or change the test from 'list' to 'List')
OK now?
Suleiman Leadbitter
15,805 PointsSuleiman Leadbitter
15,805 PointsYAY!!! It worked!!
To be honest I didn't think the
h1
would have been the problem and I thought it was more looking for<%= link_to
type of code :/My bad. Seriously though THANK YOU! Now give it five minutes and I'll get stuck on the next video lol. But seriously, I probably will :/
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHey - no problem!
Keep plugging away - that track presents a LOT of challenges (indeed I gave up right at the last hurdle!).
But keep going, and feel free to ask away in the forum; tag me if you want a quicker response; just add an @ symbol and it links to the Treehouse users.
Back to your issue, though. The test was looking at the content of the page. That can be anything, whether it's an h1, or whatver - the
expect(page).to have_content("Steve")
means precisely that - the page must contain letter-for-letter the word Steve somewhere on the page.The erb stuff which is shown with the <%= and <% tags can cause issues, yes, but the tests for those would be a little different. You'll see! And learning the difference between the two tags is useful too. I'm not sure that's ever made clear within the course.
Best of luck - shout if you need a hand!
Steve.