Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial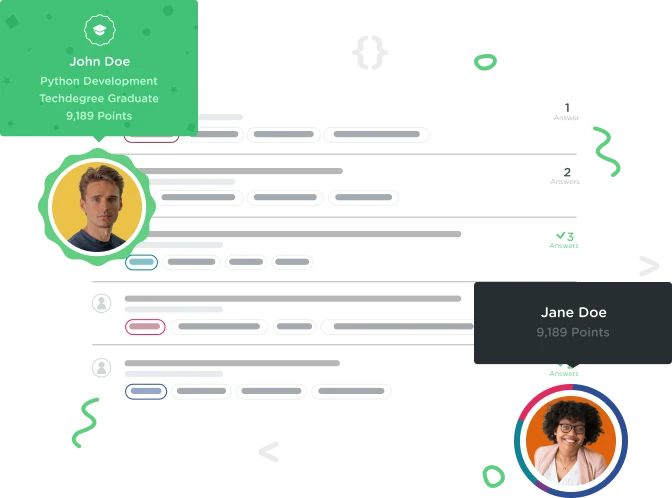

Alexander Olson
13,067 PointsWriting a Detail Query
Need help
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
[Required, StringLength(200)]
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == c.Courses.Id);
}
}
}
}
3 Answers
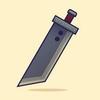
Allan Clark
10,810 Pointslooks like you just need to check the id of the course with the passed in parameter. This is what you have
.SingleOrDefault(c => c.Id == c.Courses.Id);
the issue is that the variable 'c' here stands for the course that is being compared, so 'c' should only be on one side of the '==' operator. Try changing 'c.Courses.Id' to just the passed in id parameter.
p.s. for future reference, when you have a compile error click the 'Preview' button and copy and paste the error along with you code. Helps out tons.
Happy Coding!!

Alexander Olson
13,067 Pointspublic static Course GetCourse(int id) { return context.Courses .Include(c => c.Teacher) .SingleOrDefault(c => c.Id == GetCourseId); }
Repository.cs(32,20): error CS0103: The name `context' does not exist in the current context Compilation failed: 1 error(s), 0 warnings

Alexander Olson
13,067 PointsAre you importing a screen capture to get the look in your answer? This answer didn't work for me.
How do I post the code like you did?
Thanks for your help Allan!
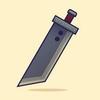
Allan Clark
10,810 Pointsyou can mark a segment of your post as code by using three back ticks ``` and the name of the language. click where it says 'Markdown Cheatsheet' below the text box for full details.
try it with this:
public static Course GetCourse(int id)//this
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == id); //here we need the 'id' variable that is passed in as a parameter
}
}