Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial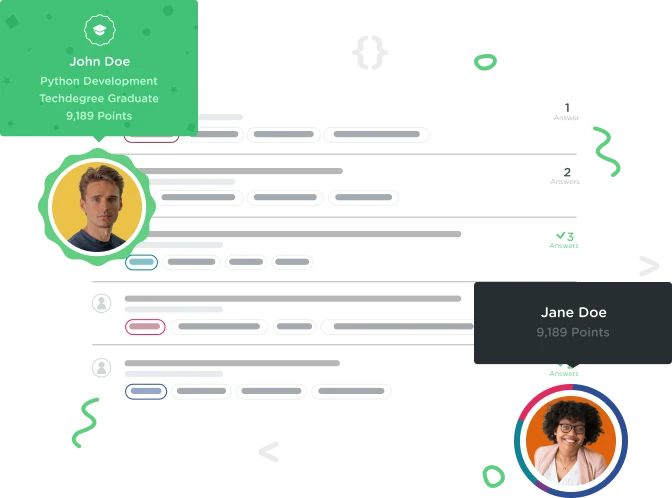

Amaka Nnorom
215 Pointswriting a program for math calculation
A typical quadratic equation is of the form ax² + bx + c = 0 where 'a', 'b' and 'c' are constants. 'a' can never be equal to zero. 'x' is a variable (the root of the quadratic equation). A quadratic equation has 2 roots and the formula for calculating the roots of a quadratic equation is given in the image below. Given the quadratic equation 2x² + 5x + 3 then, 'a' = 2, 'b' = 5 and 'c' = 3. Write a python program to calculate the roots of a quadratic equation for any combination of values for 'a', 'b', 'c' and display the roots as output. HINT: You can use three input functions to receive the values of a, b and c from the user. Also, write an expression for the quadratic formula. Do not use the python math module. Captionless Image
1 Answer

jhon white
20,006 Pointsdef sqrt(n):
return n**(1/2)
print("Quadratic function : (a * x^2) + b*x + c")
a = float(input("a: "))
b = float(input("b: "))
c = float(input("c: "))
r = b**2 - 4*a*c
if r > 0:
num_roots = 2
x1 = (((-b) + sqrt(r))/(2*a))
x2 = (((-b) - sqrt(r))/(2*a))
print("There are 2 roots: %.2f and %.2f" %(x1,x2))
elif r == 0:
num_roots = 1
x = (-b) / 2*a
print("There is one root: ", x)
else:
num_roots = 0
print("No roots, discriminant < 0.")
exit()