Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial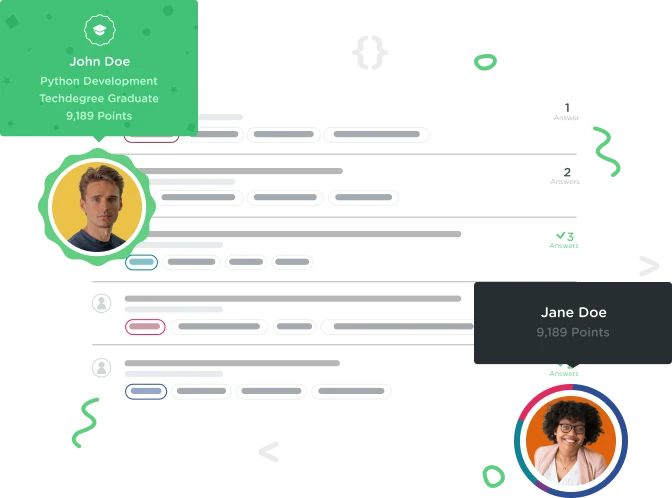
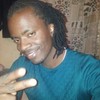
Adlight Sibanda
5,701 PointsWriting files
The following code is similar to what you just wrote. Modify this code to only write the file if it does not already exist. Hint: Use the method from the File class that checks to see if a file exists or not.
import java.io.InputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class FileUtilities {
public static boolean copyResult;
public static void saveAssetImage(Context context, String assetName) {
File fileToWrite = new File(context.getFilesDir(), assetName);
AssetManager assetManager = context.getAssets();
if (fileToWrite.exists() == false) { copyResult = copyFile(in, out); }
try {
InputStream in = assetManager.open(assetName);
FileOutputStream out = new FileOutputStream(fileToWrite);
copyResult = copyFile(in, out);
} catch(FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
private static boolean copyFile(InputStream in, FileOutputStream out) {
// Copy magic intentionally omitted
return true;
}
}
2 Answers
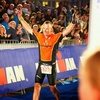
Steve Hunter
57,712 PointsHi there,
You're very close indeed!! Rather than testing for .exists() == false
(which is odd; if it doesn't exist does it know that?) test for that being not true:
if(!fileToWrite.exists()){
copyResult = copyFile(in, out);
}
I hope that helps!
Steve.

Alwin Lazar V
5,267 PointsI have solved and it works for me
public class FileUtilities {
public static boolean copyResult;
public static void saveAssetImage(Context context, String assetName) {
File fileToWrite = new File(context.getFilesDir(), assetName);
AssetManager assetManager = context.getAssets();
try {
InputStream in = assetManager.open(assetName);
FileOutputStream out = new FileOutputStream(fileToWrite);
// here I changed
if (fileToWrite.exists() == false) { copyResult = copyFile(in, out); }
} catch(FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
private static boolean copyFile(InputStream in, FileOutputStream out) {
// Copy magic intentionally omitted
return true;
}
}
Happy coding
[MOD: edited code block]
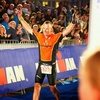
Steve Hunter
57,712 PointsGood work.
As in the above post, an alternative test to fileToWrite.exists() == false
, you can use !fileToWrite.exists()
- it amounts to the same thing.
Steve.
Adlight Sibanda
5,701 PointsAdlight Sibanda
5,701 PointsThanx Steve
seems in my code I had Capitalized: if (FileToWrite.exists() == false) { copyResult = copyFile(in, out); }
Instead of:
if (fileToWrite.exists() == false) { copyResult = copyFile(in, out); }
and it passed .... :-}
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsGreat work - glad you got it sorted out. :-)
Steve.