Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial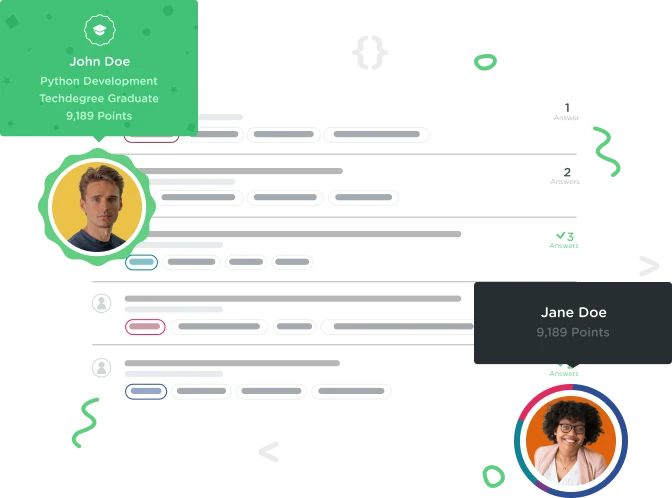
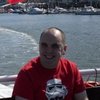
Sean Flanagan
33,235 PointsWrong error message
Why do I get a 500 error message instead of 404?
My app.js:
const express = require("express");
const bodyParser = require("body-parser");
const cookieParser = require("cookie-parser");
const app = express();
app.use(bodyParser.urlencoded({extended: false}));
app.use(cookieParser());
app.set("view engine", "pug");
app.use((req, res, next) => {
console.log("Hello");
const err = new Error("Oh no!");
err.status = 500;
next(err);
});
app.use((req, res, next) => {
console.log("world");
next();
});
app.get("/", (req, res) => {
const name = req.cookies.username;
if (name) {
res.render("index", {name});
} else {
res.redirect("/hello");
}
res.render("index", {name: name});
});
app.get("/cards", (req, res) => {
res.render("card", {prompt: "Who is buried in Grant's tomb?"});
});
app.get("/hello", (req, res) => {
const name = req.cookies.username;
if (name) {
res.redirect("/");
} else {
res.render("hello");
}
});
app.post("/hello", (req, res) => {
res.cookie("username", req.body.username);
res.redirect("/");
});
app.post("/goodbye", (req, res) => {
res.clearCookie("username");
res.redirect("/hello");
});
app.use((req, res, next) => {
const err = new Error("Not Found");
err.status = 404;
next(err);
});
app.use((err, req, res, next) => {
res.locals.error = err;
res.status(err.status);
res.render("error", err);
});
app.listen(3000, () => {
console.log("The application is running on localhost:3000!");
});
Thanks in advance for any help.
4 Answers
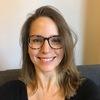
Natalie Cluer
18,898 PointsAt the beginning of your app you are throwing a 500 error:
app.use((req, res, next) => {
console.log("Hello");
const err = new Error("Oh no!");
err.status = 500;
next(err);
});
when you use app.use, the code inside will run every time. Your program never gets the chance to get to your 404 error.

Francisco Ortiz
2,673 PointsYes, he did mention that part at the end of the last video, and I think that if you have made it this far, you should be asking yourself why the code doesn't work. Imagine being in an interview and they ask you why this route gives off the wrong error.

Shawn Lindsey
20,952 PointsYeah, I also caught at the end of the last video where he said that we're going to clear out the middleware we just used, so I correctly surmised he was referring to the 500 error code and that we'd start the next video by getting rid of that in order to write a proper 404 error, but when we didn't do that I was a little confused and left the code in just in case. Just a minor miscommunication, but it was a little frustrating feeling like I missed something.
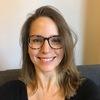
Natalie Cluer
18,898 Pointsno problem! happy coding:)

Kevin Gates
15,053 PointsTo be fair to Andrew, and to my classmates, at the end of the previous video he says we'll delete out the code causing the error.
So he did reference needing to do that. However it is also true that he didn't reiterate that at the beginning of this video (and I think he should have.)
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsHi Natalie.
I commented out that section of code. Then I ran app.js and got the 404 error.
Thanks!
Sean
Mike Hatch
14,940 PointsMike Hatch
14,940 PointsI enjoy Andrew's courses, but I notice in his courses he'll change the code without us knowing (usually between video changes). He never said to comment that part out. Perhaps he does this on purpose to challenge us and force us to debug our own code.
Steven Ventimiglia
27,371 PointsSteven Ventimiglia
27,371 PointsYeah, but it's extremely frustrating for no reason.
I've actually enjoyed this course as well, but he's the teacher and we shouldn't be asking other students why things aren't working, just to find out that it was omitted - which shows a serious lack of quality in the production of the lesson on the teacher's behalf. It's like finding a needle in a haystack, and unfair to the students.
Andrew expressed the flow of middleware, and placed that code up top. If we needed to remove it to understand why and make steady progress, he should have told us so the lesson flowed properly as well.
That's why Guil Hernandez is awesome. The guy is meticulous to the point of making sure his spacing is corrected, even if it's off by one character.
Yoan Herrera
21,137 PointsYoan Herrera
21,137 Points".... and it WORKS!!!. " famous words by Andrew. "...but HOW!!!" famous words by Andrew's students. LOL
JASON LEE
17,351 PointsJASON LEE
17,351 PointsI had the exact same issue and suspected that was the reason. However following through the video, the teacher Andrew never went over commenting out/modifying the
next(err)
part of the code at the top. So yes it could have been a good debugging challenge, had I known. But no... I'm sitting here thinking ... shouldn't we have removed thenext(err)
part of the code above? Am i missing something from the video? Hmmm... let me search through the video to see that section of the code to see if he has that.