Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial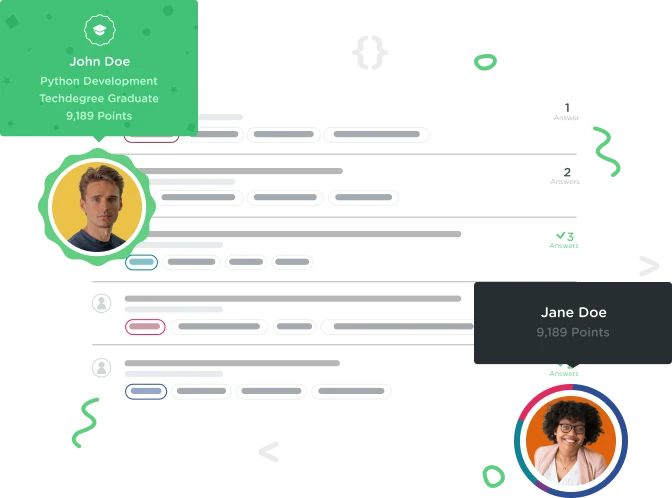

Eduardo Diaz
Courses Plus Student 6,048 Pointswrong number of prints
Make a while loop that runs until start is falsey.
Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99.
If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number.
Finally, decrement start by 1.
i dont get why am i getting wrong number of loops, the excercise clearly states to decrease the start value by 1 i know i can also put the start -= 1 outside of the branching conditionals and it ill execute either way, buuuuuuut that aint working either :/
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start == 5:
num = random.randint(1,99)
if even_odd(num) == True:
print("{} is even".format(num))
start -=1
else:
print("{} is odd".format(num))
start -= 1
1 Answer

andren
28,558 PointsThe issue is the condition of the loop. You are telling Python to only run the code while start
equals 5. That means it will only run once since you change start from 5 to 4 within the loop.
The instructions tell you to:
Make a while loop that runs until start is falsey.
Python will automatically convert non-boolean (true
or false
) values to a boolean based on whether they are considered a truthy or falsey value.
Python has a list of values it considers falsey
which is this:
- None
- False
- zero of any numeric type, for example, 0, 0.0, 0j.
- any empty sequence, for example, '', (), [].
- any empty mapping, for example, {}.
- instances of user-defined classes, if the class defines a bool() or len() method, when that method returns the integer zero or bool value False. [1]
Any value not found in that list is considered truthy.
That means that start
will be a truthy value while it contains any number above 0, and be considered falsey the moment it becomes 0. Therefore the loop does not need to have any condition beyond the start
variable itself. Like this:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start: # Run until start is falsey (0 in this case)
num = random.randint(1,99)
if even_odd(num) == True:
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start -= 1
That will result in the loop running 5 times which is the intended outcome. You could of course also use a condition like start != 0
but that would be redundant in this case.
Cheo R
37,150 PointsCheo R
37,150 PointsThe problem is because of the line:
It is only true for the the first iteration. Once start is no longer 5, that line is no longer true, and breaks out of the loop.
Changing it to something like
should get you five loops before breaking out of the while statement.