Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial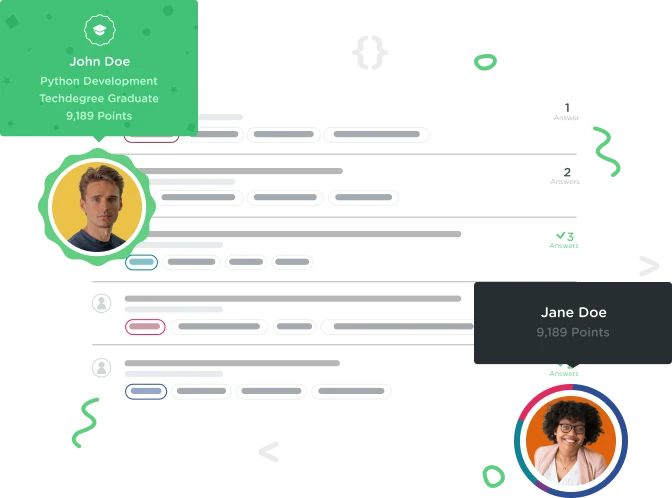

Jonathan Osteen
Courses Plus Student 4,439 PointsWrong number of prints... why?
I can see how to make this work from the forum, but I don't see what's wrong with what I did. Anyone have any idea why it is returning "Wrong number of prints"?
Thx!
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
#run while loop until start is false
while start is True:
#get a random number between 1 and 99
num = random.randint(1,99)
#if even, print as much
if (even_odd(num) is True):
print("{} is even".format(num))
#if odd, print as much
else:
print("{} is odd".format(num))
#decrement start by 1
start -= 1
3 Answers
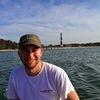
Thomas Harris
7,647 PointsJonathan,
I'm relatively new to Python myself, but I do have a background in C where this makes a bit more sense. I've thought a bit about this and the best I can come with is that 'True' or 'False' literally equate to 1 and 0 in Python (to illustrate this, here I use int() in a Python shell to cast 'True' to an integer):
tommy@tommy ~ $ python3.5
Python 3.5.2 (default, Nov 17 2016, 17:05:23)
[GCC 5.4.0 20160609] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> True
True
>>> print(True)
True
>>> int(True) # cast the Boolean 'True' to its integer value
1
>>>
So in the code we're working with here, the variable 'start' is set to 5. Because the first bit of code is the function declaration for 'even_odd()', the first line of code that runs is the while statement. Another thing to note is that in Python, the word 'is' is synonymous with 'equals', or the comparison operator '=='. (not entirely true, see my edit below).
So if you break down the while statement:
while start is True:
# in this case, when start = 5, then this is the comparison that is taking place:
while (5 == 1):
This is why the program doesn't appear to run; the criterion for this while statement is not met and the program skips the entire loop and moves on (to the end of the program, in this case). I recommend you read the Python Documentation about truth value testing, which is what a while statement is based on.
To sum up, when you use "while start:", you're saying while the variable start is not zero, then run this loop. When you say "while start is True:", you're saying "while start is literally equal to True", then continue. I hope this makes sense, sorry if it's a bit long-winded! Let me know if it's still not clear.
Regards,
-Tommy Harris
EDIT: Upon reading a bit more, 'is' and '==' are not necessarily synonymous... to quote this answer from Stack Overflow:
is will return True if two variables point to the same object, == if the objects referred to by the variables are equal.
Hopefully this isn't too complicated... but when you use 'is', you're essentially saying: is the variable 'start' the same object as the literal 'True', which is not the case here.

Jonathan Osteen
Courses Plus Student 4,439 PointsThank you Thomas.
As it relates to the response I received, this is what the Treehouse quiz console itself returned. Running it in workspace, I didn't get any response.
I can get it to work making the changes above, but I don't understand why simply indenting the decrementing line to be within the while statement is not enough? As an inexperienced programmer, I like to make the code something I can read linguistically. Do you know why having "is True" written out prevents the code from working?
Thank you

Jonathan Osteen
Courses Plus Student 4,439 PointsHi Thomas,
Thank you, that was very helpful indeed! I appreciate the time and consideration you put into it.
Thomas Harris
7,647 PointsThomas Harris
7,647 PointsHello Jonathan,
I'm not entirely certain what you mean by "returning 'Wrong number of prints'". Looking at your code here though, the while loop will never end because the start decrement falls outside of the while loop (the start variable needs to be decremented at the end of each iteration inside of the loop). Furthermore, I couldn't even get this to start properly on my Linux workstation because of some of the comparison statements. Instead of saying 'while start is True:", it is sufficient to say "while start:", or "if even_odd(num):". I was able to get your code working with this:
Regards,
-Tommy Harris